mongodb/mongoose findMany - find all documents with IDs listed in array
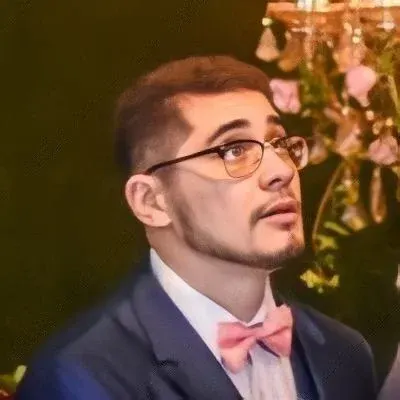
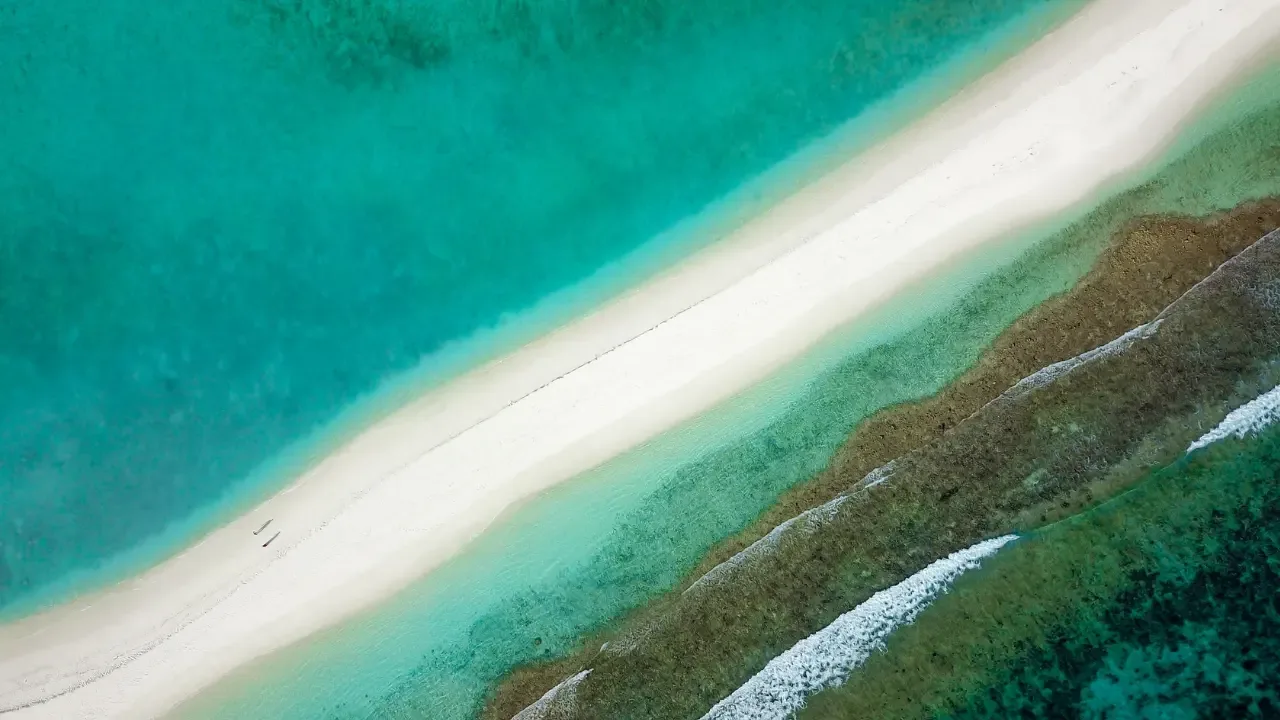
MongoDB/Mongoose: Finding Many Documents with IDs Listed in an Array
Are you grappling with the challenge of finding multiple MongoDB documents based on a list of IDs? You're not alone! Many developers struggle to implement an efficient solution for this common issue. 🧩
The Problem
Let me set the stage for you. You have an array of _ids
, and your objective is to retrieve all the corresponding documents that match those IDs. Naturally, your first instinct might be to use the find()
method from the Mongoose library:
model.find({
'_id' : [
'4ed3ede8844f0f351100000c',
'4ed3f117a844e0471100000d',
'4ed3f18132f50c491100000e'
]
}, function(err, docs){
console.log(docs);
});
However, you quickly discover that this solution doesn't work as expected. 😫
The Solution
But fret not! There is a simple and elegant solution to this conundrum. 🎉 To find multiple documents with IDs listed in an array, you need to use MongoDB's $in
operator in conjunction with Mongoose's find()
method.
Here's how you can achieve this:
const ids = [
'4ed3ede8844f0f351100000c',
'4ed3f117a844e0471100000d',
'4ed3f18132f50c491100000e'
];
model.find({
'_id': { $in: ids }
}, function(err, docs){
console.log(docs);
});
By using $in
and passing the array of IDs, you can now retrieve all the documents that match those IDs. 🎯
Handling Large Arrays
Okay, let's address another critical point. 📌 What if your array contains a whopping number of IDs, potentially hundreds or more? You may encounter performance issues. Fear not! MongoDB has your back with a feature called "Batch Size."
By default, MongoDB processes query results in batches of 101 documents. However, you can change this value according to your needs. For example, to set the batch size to 500, you can modify your query like this:
model.find({
'_id': { $in: ids }
}).batchSize(500).exec(function(err, docs){
console.log(docs);
});
Adjusting the batch size allows you to optimize performance and retrieve documents more efficiently. 🚀
The Call-to-Action
There you have it! A simple solution to finding many documents with IDs listed in an array using MongoDB and Mongoose. 🙌
If you found this blog post helpful, don't forget to share it with fellow developers who might be facing the same issue. Let's spread the knowledge! 💡
Have you encountered any other MongoDB challenges? Share them in the comments below, and let's brainstorm solutions together! Let's keep learning and growing as a tech community. 👩💻👨💻
Keep coding and happy querying! 🖥️💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
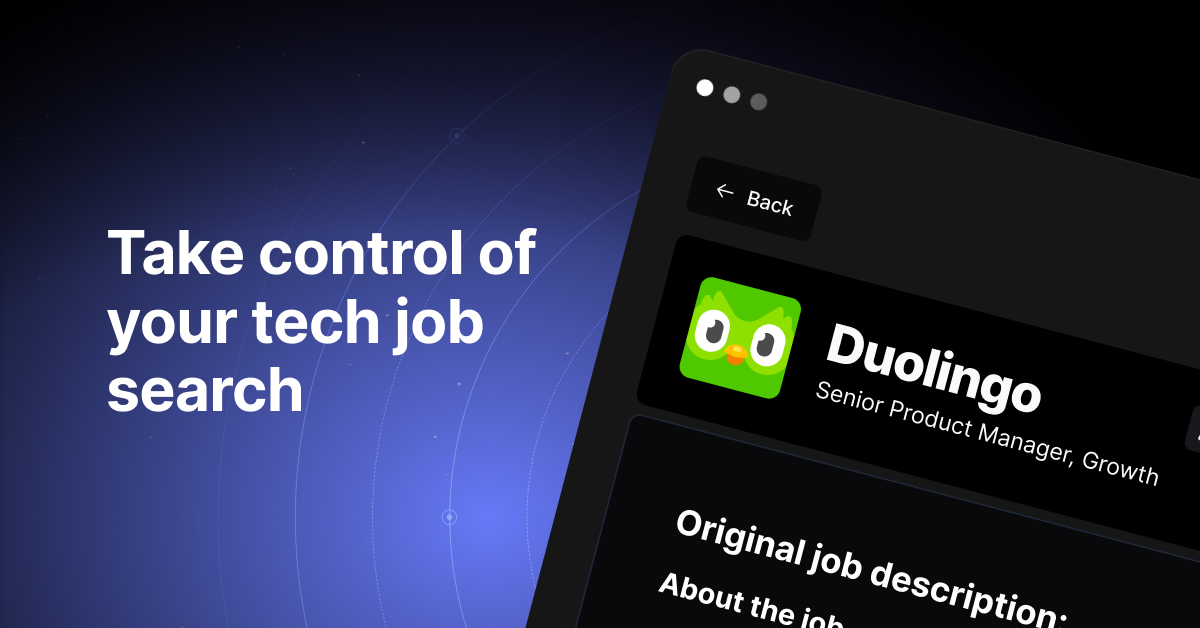