Is there a way to get version from package.json in nodejs code?
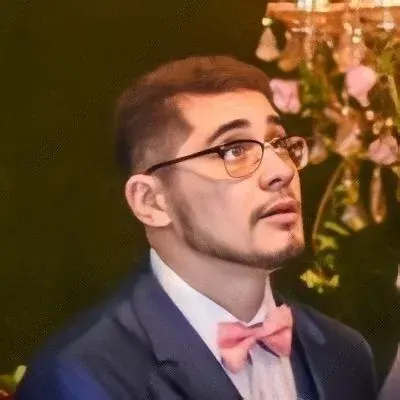
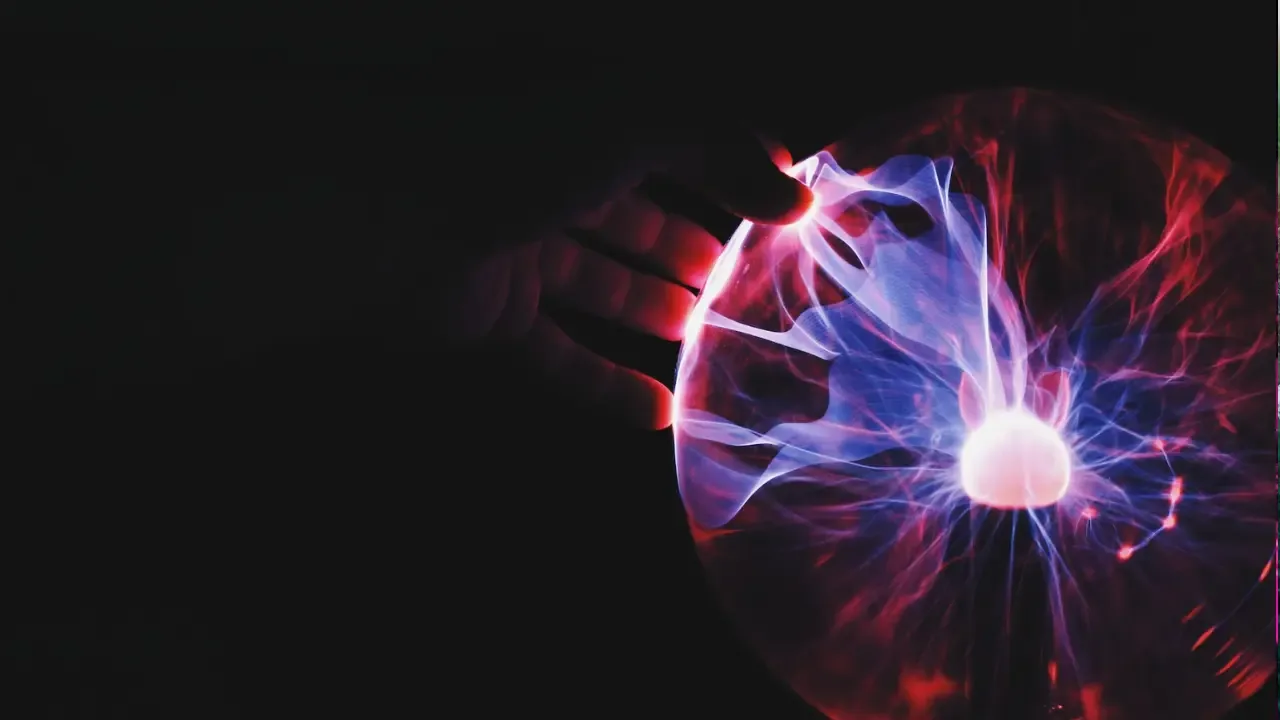
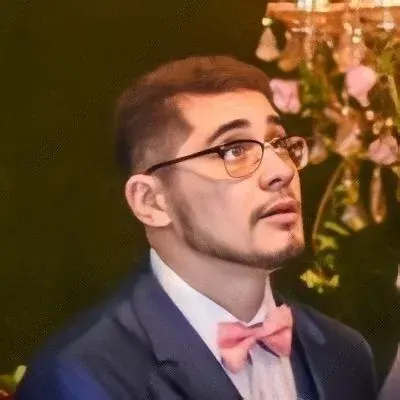
Get the Version from package.json in Node.js Code: A Simple Guide
Introduction
Are you working on a Node.js application and wondering if there's a way to fetch the version defined in the package.json
file? 🤔 Well, you're in the right place! In this guide, we will explore a simple solution to this common problem and make your life as a Node.js developer easier. So, let's dive right in! 🚀
The Problem
Imagine you have a Node.js app, and you want to access the version specified in the package.json
file programmatically in your code. You might be using a framework like Express, and would like to log the version along with other server information. Here's an example:
var port = process.env.PORT || 3000;
app.listen(port);
console.log("Express server listening on port %d in %s mode %s", app.address().port, app.settings.env, app.VERSION);
The question is: how can we access the VERSION
from package.json
and display it dynamically in our code? 🤔
The Solution
Luckily, Node.js provides a straightforward way to access the package.json
file and read its contents. All you need to do is follow these three simple steps:
Step 1: Require the package.json
File
In your Node.js script, require the package.json
file using the fs
module:
const fs = require('fs');
const packageJson = fs.readFileSync('./package.json', 'utf-8');
Step 2: Parse the JSON Data
Parse the JSON data from package.json
using the JSON.parse()
method:
const packageData = JSON.parse(packageJson);
Step 3: Access the Version
Finally, access the version
property from the parsed packageData
object:
const version = packageData.version;
Putting It All Together
Let's combine the three steps and integrate the solution into our original code:
const fs = require('fs');
const packageJson = fs.readFileSync('./package.json', 'utf-8');
const packageData = JSON.parse(packageJson);
const version = packageData.version;
var port = process.env.PORT || 3000;
app.listen(port);
console.log("Express server listening on port %d in %s mode %s", app.address().port, app.settings.env, version);
That's it! You can now dynamically access the version from package.json
in your Node.js application. How cool is that? 😎
Conclusion
In this guide, we learned a simple yet powerful way to fetch the version from package.json
in a Node.js application. By following the three easy steps, you can seamlessly integrate the version into your code and display it dynamically. Now, go ahead and apply this solution to your own projects, and let us know if you found it helpful! 💪
Do you have any other Node.js-related questions or want to share your thoughts? We'd love to hear from you in the comments below! Let's keep the conversation going. 🎉