Can an AWS Lambda function call another
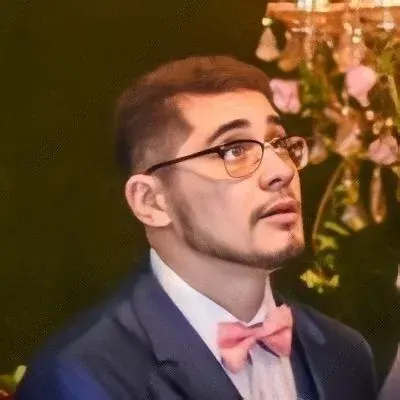
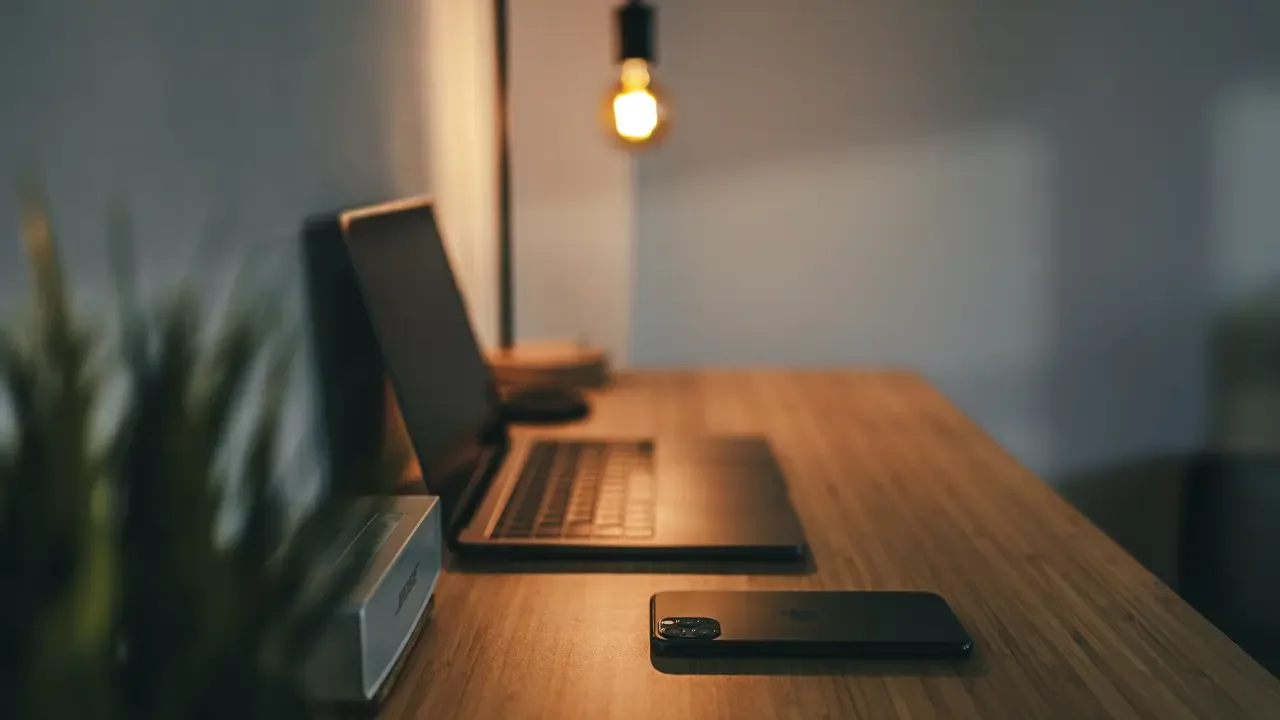
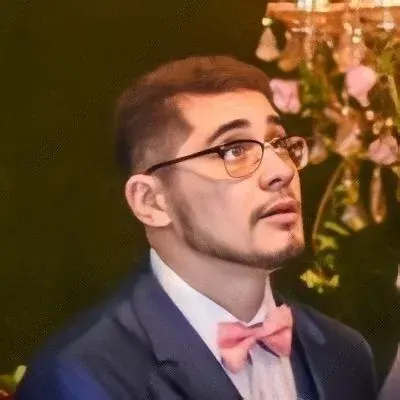
🤔 Can an AWS Lambda function call another?
Hey there tech enthusiasts! 👋 Are you wondering if it's possible for one AWS Lambda function to call another? 🤔 Well, you're in luck! In this blog post, we'll dive into this common issue and provide you with easy solutions. So let's get started! 💡
🔄 Chaining AWS Lambda Functions
The scenario you mentioned in the context is a perfect use case for chaining AWS Lambda functions. You have two functions: one that produces a quote and another that turns a quote into an order. Instead of receiving the quote directly from an untrusted client, you want the order function to call the quote function to regenerate the quote. Sounds reasonable, right? Let's see how we can achieve this! 🚀
1️⃣ Synchronous Invocation
One way to make a Lambda function call another is by using synchronous invocation. With this approach, the order function directly calls the quote function and waits for the response before proceeding further.
To achieve synchronous invocation, you need to ensure the following:
Both Lambda functions are in the same region.
The order function has the necessary permissions to invoke the quote function.
Configure the order function to invoke the quote function directly.
Here's a code snippet example for synchronous invocation in Node.js:
const AWS = require('aws-sdk');
const lambda = new AWS.Lambda();
exports.handler = async (event, context) => {
const quoteParams = {
FunctionName: 'quoteLambda', // Name of the quote function
InvocationType: 'RequestResponse',
Payload: JSON.stringify({}) // Optional payload to pass as input
};
try {
const quoteResponse = await lambda.invoke(quoteParams).promise();
const regeneratedQuote = JSON.parse(quoteResponse.Payload);
// Now you can use the regenerated quote in your order logic
// ...
return {
statusCode: 200,
body: 'Order placed successfully!'
};
} catch (error) {
console.error(error);
return {
statusCode: 500,
body: 'Failed to place the order.'
};
}
};
2️⃣ Asynchronous Invocation
Another option to make a Lambda function call another is through asynchronous invocation. In this case, the order function triggers the quote function but doesn't wait for the response. Instead, it continues with its execution and can retrieve the response later if needed.
Similar to synchronous invocation, you need to ensure you have the necessary permissions set up for invoking the quote function. Here's a code snippet example for asynchronous invocation in Python:
import boto3
def lambda_handler(event, context):
client = boto3.client('lambda')
quote_params = {
'FunctionName': 'quoteLambda', # Name of the quote function
'InvocationType': 'Event',
'Payload': json.dumps({}) # Optional payload to pass as input
}
response = client.invoke_async(**quote_params)
# Continue with order logic
return {
'statusCode': 200,
'body': 'Order placed successfully!'
}
🏁 Let's Get Chaining!
And there you have it! Whether you want to use synchronous invocation or asynchronous invocation, you can easily chain AWS Lambda functions to accomplish your goal. Remember, synchronous invocation waits for the response, while asynchronous invocation allows your order function to proceed without waiting.
Now it's your turn! 🙌 Have you ever chained Lambda functions in your projects? Or do you have any other creative use cases for AWS Lambda? Share your thoughts, experiences, and suggestions in the comments below. Let's learn and grow together! 🌟