setState() or markNeedsBuild called during build
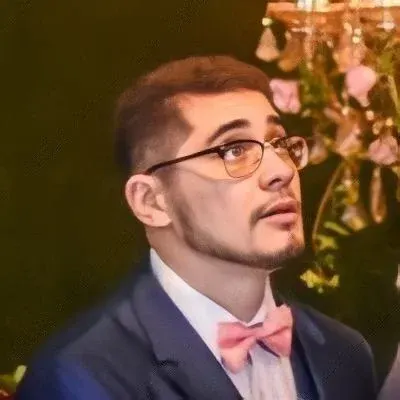
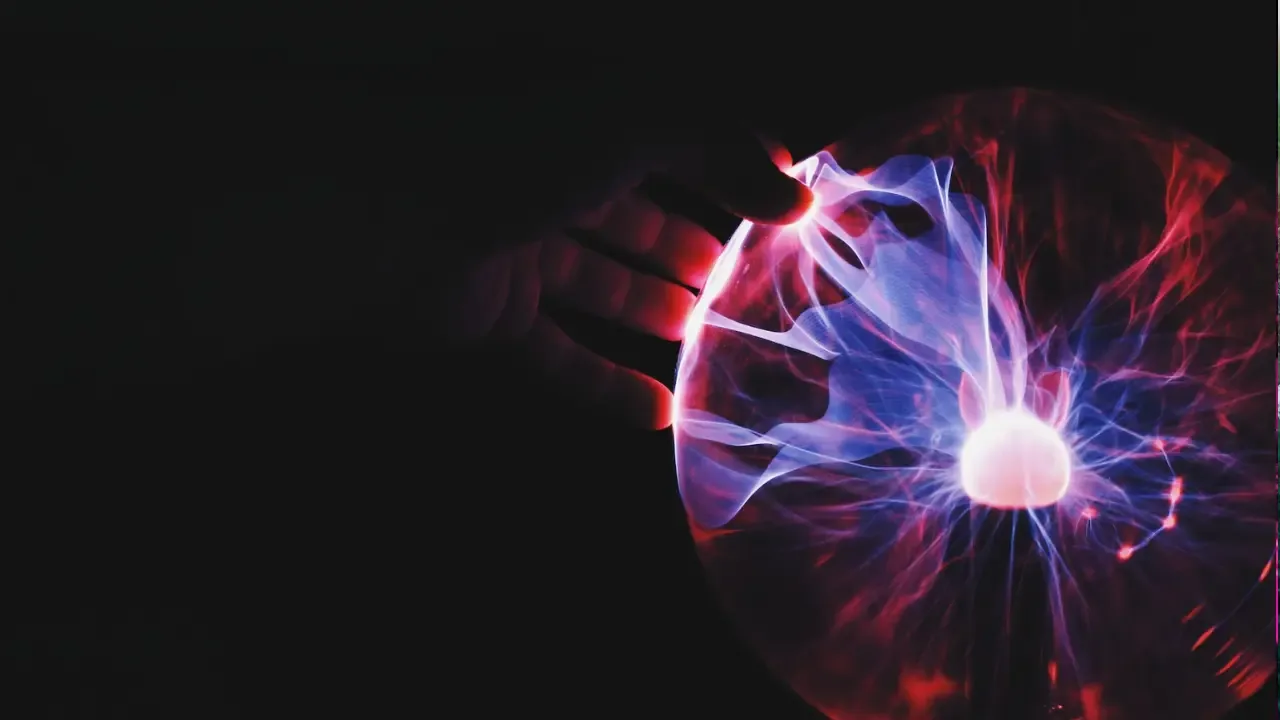
Blog Post: Understanding and Fixing "setState() or markNeedsBuild called during build" Error
š Hey there, tech enthusiasts! Today, we're going to tackle a common issue that many Flutter developers face: the dreaded "setState() or markNeedsBuild called during build" error. 𤯠This error usually occurs when you try to update the state of a widget while it's still being built. But don't worry ā we've got your back! Let's dive in and find some easy solutions to resolve this error. šŖ
Understanding The Problem
First, let's understand why this error occurs. In the provided code, the setState()
method is called inside the buildlist()
method, which is invoked when a button is pressed. However, calling setState()
during the build phase triggers a rebuild, leading to an infinite loop and causing the "setState() or markNeedsBuild called during build" error. š±
š« Problem 1: The "Horizontal viewport was given unbounded height" Error
The first error you encountered, "Horizontal viewport was given unbounded height," is caused by the ListView.builder()
widget inside the build()
method. To fix this, simply wrap the ListView.builder()
with an Expanded
widget, allowing it to take up the remaining available space. š
Expanded(
child: ListView.builder(
...
),
)
By using Expanded
, the ListView.builder()
will adapt to the available height and prevent the "unbounded height" error. š
š« Problem 2: The "setState() or markNeedsBuild called during build" Error
The second error, "setState() or markNeedsBuild called during build" occurs due to the same setState()
call in the buildlist()
method. To resolve this issue, we need to separate the time when setState()
is called from the build phase. š
To achieve this, we can use a GestureDetector
widget with an onTap
callback instead of directly calling buildlist()
in the onPressed
property of the FlatButton
. This way, the state will only be updated when the button is tapped, after the build phase is complete. š”
Here's an example of how you can implement the GestureDetector
widget:
GestureDetector(
onTap: () => buildlist('button' + index.toString()),
child: FlatButton(
...
),
)
By implementing the GestureDetector
and moving the setState()
call out of the build()
method, you'll no longer face the "setState() or markNeedsBuild called during build" error. š
š ļø Final Code
Here's the updated code with the solutions implemented:
class MyHomePage2 extends State<MyHome> {
List items = [];
buildlist(String s) {
setState(() {
// Your logic here
});
}
@override
Widget build(BuildContext context) {
// Your existing code here
return Scaffold(
drawer: drawer,
appBar: AppBar(
title: Text('Booze Up'),
),
body: Column(
children: <Widget>[
Expanded(
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: 4,
itemBuilder: (BuildContext context, int index) {
return Column(
children: <Widget>[
GestureDetector(
onTap: () => buildlist('button' + index.toString()),
child: FlatButton(
child: Image.asset(
'images/party.jpg',
width: width,
height: width,
),
onPressed: null,
),
),
],
);
},
),
),
Expanded(
child: ListView(
padding: EdgeInsets.fromLTRB(10.0, 10.0, 0.0, 10.0),
children: items,
scrollDirection: Axis.vertical,
),
),
],
),
floatingActionButton: FloatingActionButton(
onPressed: null,
child: Icon(Icons.add),
),
);
}
// Your existing code here
}
š¢ Your Next Steps
Give the updated code a try, and both of the errors should be resolved. š„³ If you encounter any other issues or have any further questions, feel free to ask in the comments below. Our community is always here to help you out. š¬
Remember, š practice makes perfect! Keep exploring Flutter and building amazing apps. And if you found this blog post helpful, don't forget to share it with your fellow developers. Let's spread the knowledge! š
Happy coding! š»āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
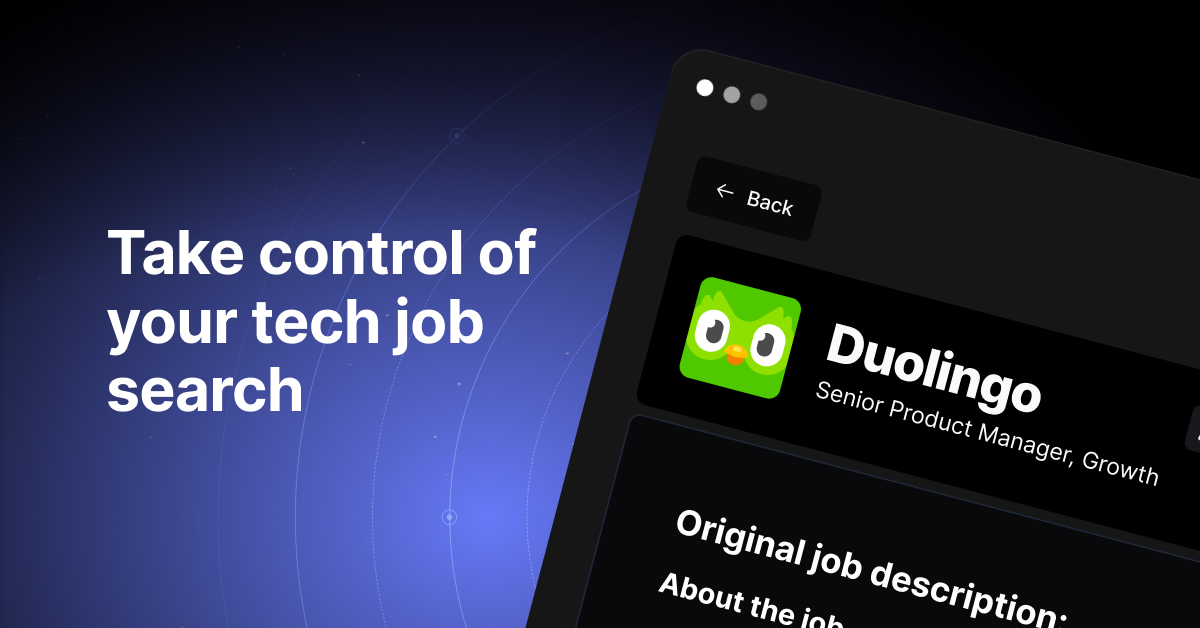