Use jQuery to hide a DIV when the user clicks outside of it
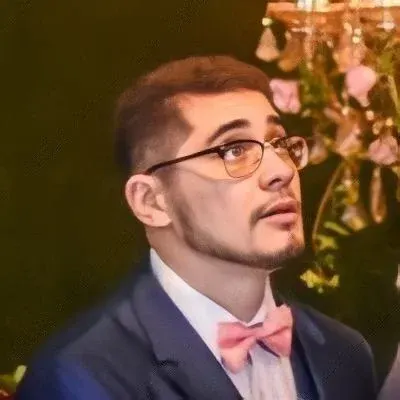
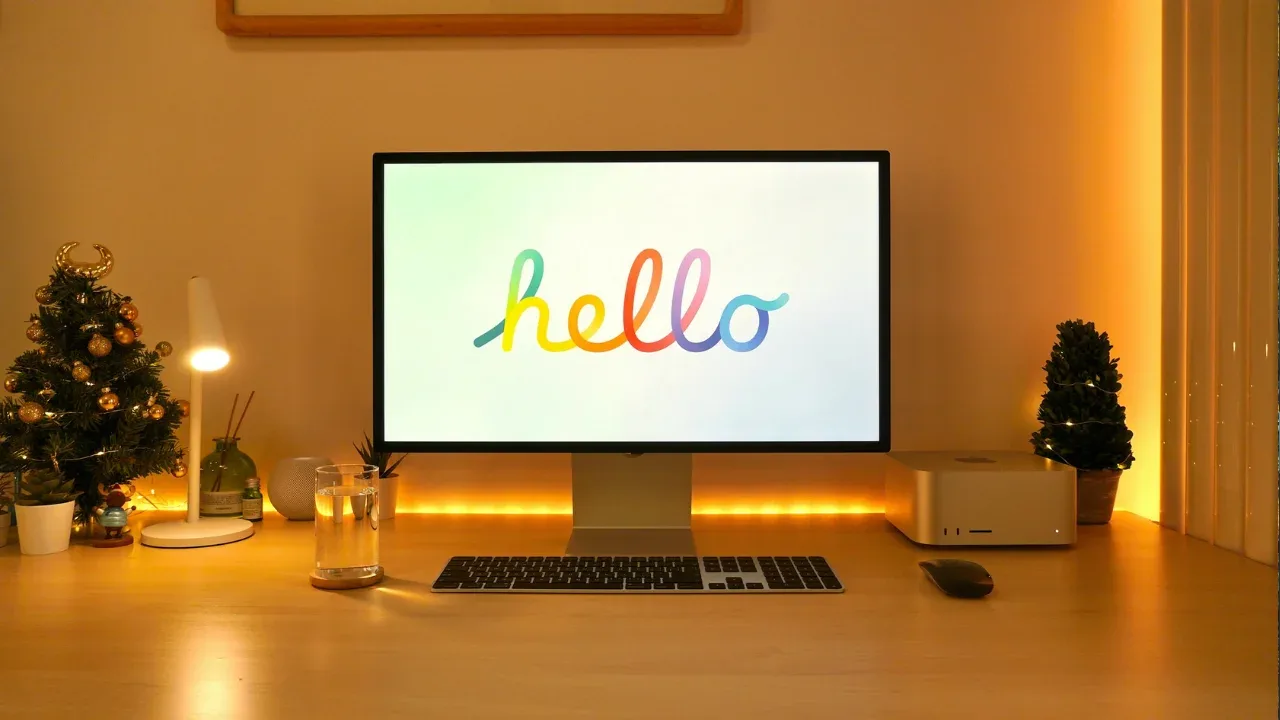
🕶 Hide a DIV When Clicking Outside: Easy Solution Guide
Hey there! 👋 Are you struggling with hiding a <div>
when the user clicks outside of it using jQuery? Don't worry, I've got your back! In this post, I'll address a common issue related to this and provide you with an easy solution. Let's dive in! 🏊♂️
The Problem: Links Inside the DIV Stop Working
So, you have implemented the following code:
$('body').click(function() {
$('.form_wrapper').hide();
});
$('.form_wrapper').click(function(event){
event.stopPropagation();
});
And your HTML looks like this:
<div class="form_wrapper">
<a class="agree" href="javascript:;">I Agree</a>
<a class="disagree" href="javascript:;">Disagree</a>
</div>
But now you've encountered a pesky problem. The links inside the <div>
no longer work when clicked. 😱
The Cause: Event Bubbling and Event Propagation
The issue arises due to event bubbling and event propagation in jQuery. When you click on a link inside the <div>
, the click event propagates to the surrounding <div>
and triggers the hide action. As a result, the links become unresponsive. 😓
The Solution: Prevent Event Propagation for Links
To resolve this problem and make the links inside the <div>
clickable, you need to modify your jQuery code slightly. Here's the updated solution:
$('body').click(function() {
$('.form_wrapper').hide();
});
$('.form_wrapper a').click(function(event){
event.stopPropagation();
});
By using the selector $('.form_wrapper a')
, we only target the links inside the <div>
. Then, we prevent the event from propagating further using event.stopPropagation()
. This way, the links retain their click functionality. Problem solved! 🎉
Try It Yourself
You can copy the updated code snippet above and paste it into your project. Once done, your links should be clickable inside the <div>
. Give it a go and see the magic happen! 💫
Share Your Experience!
I hope this easy solution helped you overcome the issue of hiding a <div>
when the user clicks outside of it without affecting the links inside. If you found this guide useful, share it with your fellow developers and spread the knowledge! 🌟
Got more questions or any suggestions? Feel free to leave a comment below. Let's learn from each other! Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
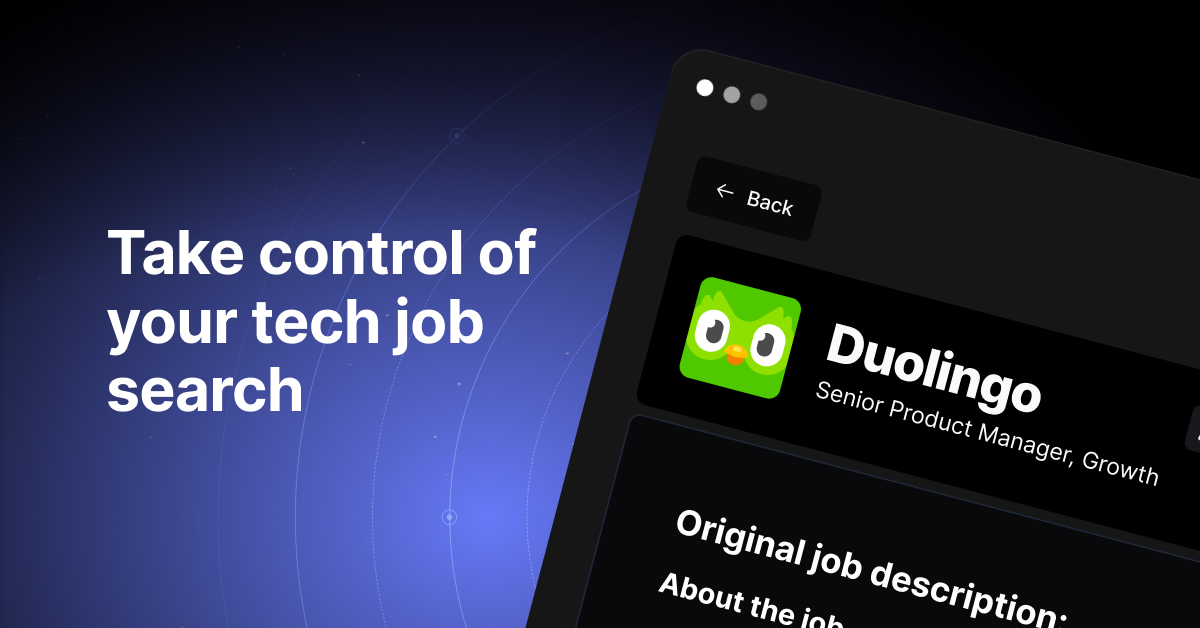