How to send a correct authorization header for basic authentication
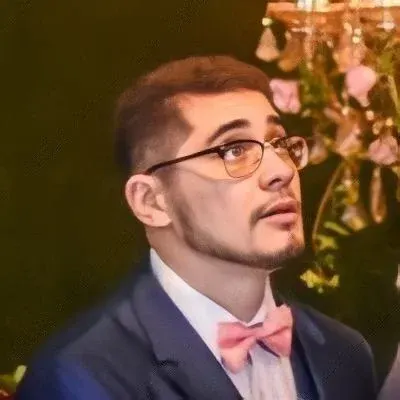
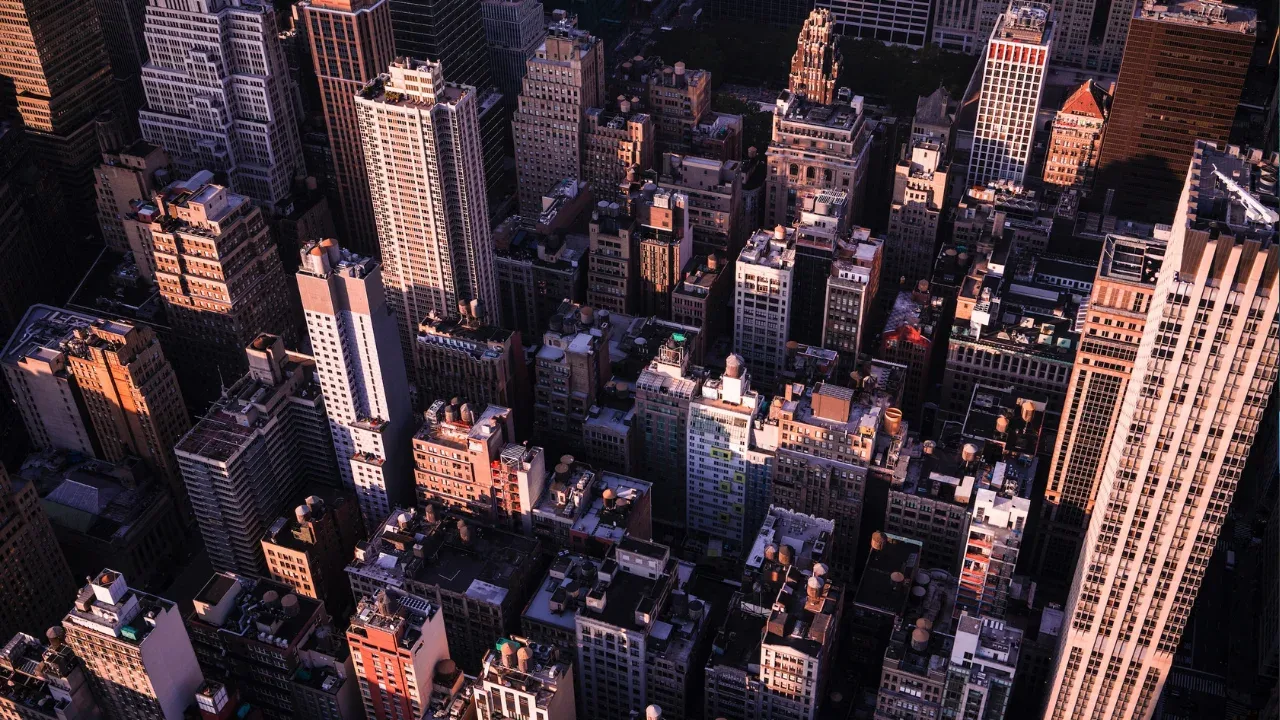
📝 How to send a correct authorization header for basic authentication
Have you ever encountered issues when trying to send data from your API using basic authentication? 🤔 It can be frustrating, but fear not! In this blog post, we will address common problems and provide easy solutions to help you send a correct authorization header.
Let's start with the basics. Basic authentication requires the client to include an Authorization
header in the request. This header consists of the word "Basic" followed by a base64-encoded string of the username and password, separated by a colon.
In the context you shared, you attempted to send the authorization header using jQuery's $.ajax()
function. Here's an example of how you can do it correctly:
$.ajax({
type: 'POST',
url: 'http://theappurl.com/api/v1/method/',
data: {},
crossDomain: true,
beforeSend: function(xhr) {
var username = 'your_username';
var password = 'your_password';
var authHeader = btoa(username + ':' + password);
xhr.setRequestHeader('Authorization', 'Basic ' + authHeader);
}
});
In the code snippet above, we first encode the username and password using JavaScript's btoa()
function. This ensures that the credentials are transmitted securely. Then, we set the Authorization
header by concatenating the word "Basic" with the encoded credentials.
Now, let's address the issue you encountered. Based on the server configuration and response you provided, it seems that the server is not properly configured to handle the OPTIONS
method. The OPTIONS
method is used to determine the server's capabilities before sending the actual request. In this case, it should be allowed to handle the POST
method.
To solve this problem, you can update your server configuration to allow the OPTIONS
method and properly handle the subsequent POST
request. Here's an example of response headers that should resolve the issue:
response["Access-Control-Allow-Origin"] = "*"
response["Access-Control-Allow-Methods"] = "POST, OPTIONS"
response["Access-Control-Max-Age"] = "1000"
response["Access-Control-Allow-Headers"] = "*"
Make sure to include the POST
method in the Access-Control-Allow-Methods
header and specify the allowed headers in the Access-Control-Allow-Headers
header. This will ensure that the browser can make the request with the correct authorization header.
Lastly, if you're still experiencing trouble, you can compare the headers you receive from the Advanced REST Client (Chrome Extension) with the ones you're sending through your code. Pay close attention to the User-Agent
, Authorization
, and Content-Type
headers. They should match or be similar to ensure compatibility.
I hope this guide has helped you understand how to send a correct authorization header for basic authentication. Remember to check your server configuration, use the correct encoding method, and double-check the headers. Happy coding! 💻🔒
Do you have any other questions or need further assistance? Feel free to leave a comment below! Let's tackle this together. 💪
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
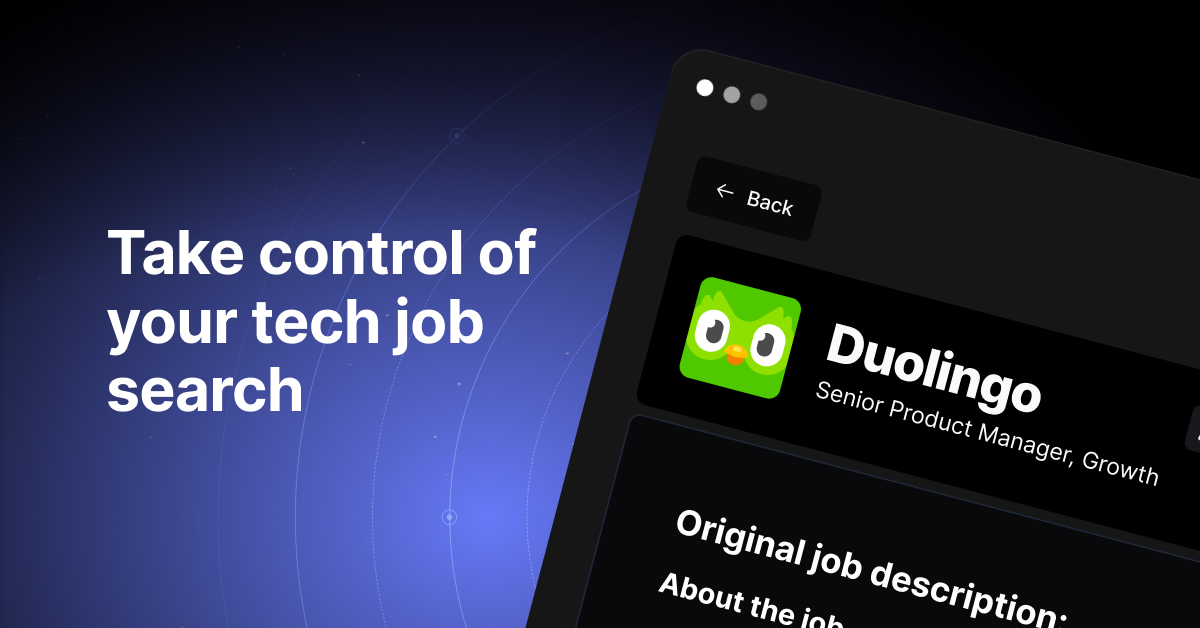