What is the equivalent of Java static methods in Kotlin?
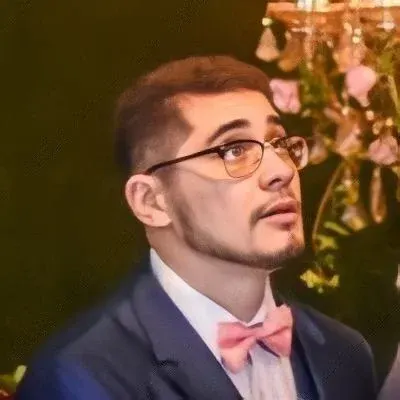
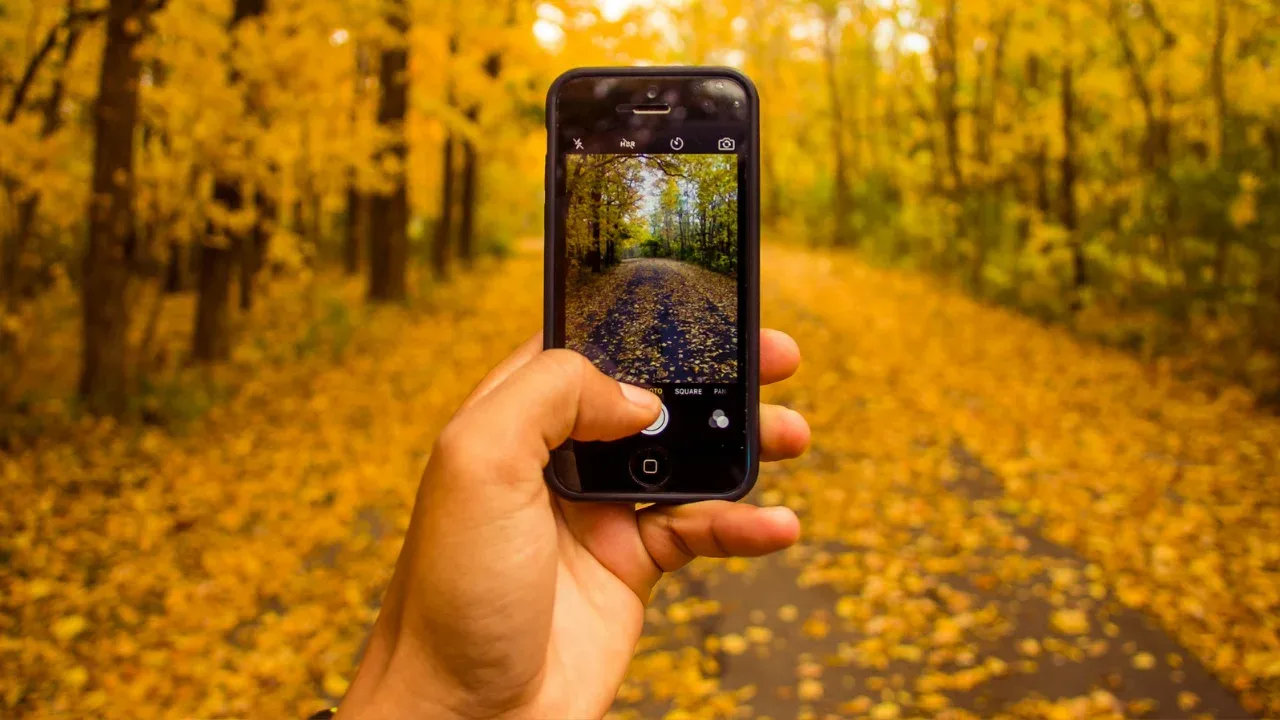
🚀 The Equivalent of Java Static Methods in Kotlin
If you're a developer who is transitioning from Java to Kotlin, you might have wondered about the absence of the static
keyword in Kotlin. While Kotlin doesn't have a direct equivalent to static methods in Java, there are alternative ways to achieve similar functionality. In this blog post, we'll explore the best approach to represent a static Java method in Kotlin and provide easy solutions to common issues. Let's dive in! 💡
Understanding the Concept 🤔
Java's static methods are class-level methods that can be accessed and called without creating an instance of the class that defines them. They are useful for groupings of utility functions, as they allow you to call these methods directly on the class itself.
How Kotlin Handles Static Methods ✨
In Kotlin, the concept of static methods is replaced by the idea of package-level functions and companion objects.
Package-Level Functions
The simplest way to represent a static method in Kotlin is by using a package-level function. Package-level functions are declared outside of any class or object and can be accessed directly in any Kotlin file within the same package.
package com.example.myapp
fun helloWorld() {
// logic goes here
}
To call the helloWorld
method, you just need to import the containing package and call the function:
import com.example.myapp.helloWorld
fun main() {
helloWorld()
}
Companion Objects
If you want to associate a function or property with a specific class, you can use a companion object. A companion object is a singleton object that is tied to the enclosing class and can have its own methods and properties.
class MyClass {
companion object {
fun myStaticMethod() {
// logic goes here
}
}
}
To call a static method using a companion object, you can use the class name as a qualifier:
MyClass.myStaticMethod()
Common Issues and Easy Solutions 💪
Issue 1: Accessing Static Java Methods in Kotlin
What if you're working with a Java library that exposes static methods and you want to call them in Kotlin? Kotlin allows you to call static Java methods directly as if they were written in Kotlin, without any additional steps.
import com.example.myapp.MyJavaClass
fun main() {
MyJavaClass.myStaticMethod()
}
Issue 2: Using Static Methods in a Derived Class
Java allows derived classes to override static methods defined in their base classes. However, Kotlin doesn't provide this capability. If you need to call a method in a derived class, you can use Kotlin's standard inheritance and override functionality, rather than relying on static methods.
open class MyBaseClass {
open fun myMethod() {
// logic goes here
}
}
class MyDerivedClass : MyBaseClass() {
override fun myMethod() {
// overridden logic goes here
}
}
Takeaways and Next Steps 📚
While Kotlin doesn't have a direct equivalent to Java static methods, package-level functions and companion objects serve as effective alternatives. Remember:
Package-level functions are declared outside of any class or object and can be accessed directly in the same package.
Companion objects are tied to their enclosing class and offer their own methods and properties.
Now that you have a better understanding of how to represent static Java methods in Kotlin, take the opportunity to refactor your Java code and explore the possibilities that Kotlin offers to enhance your projects. Happy coding! 🎉
*[Java]: A popular programming language used for building enterprise software applications.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
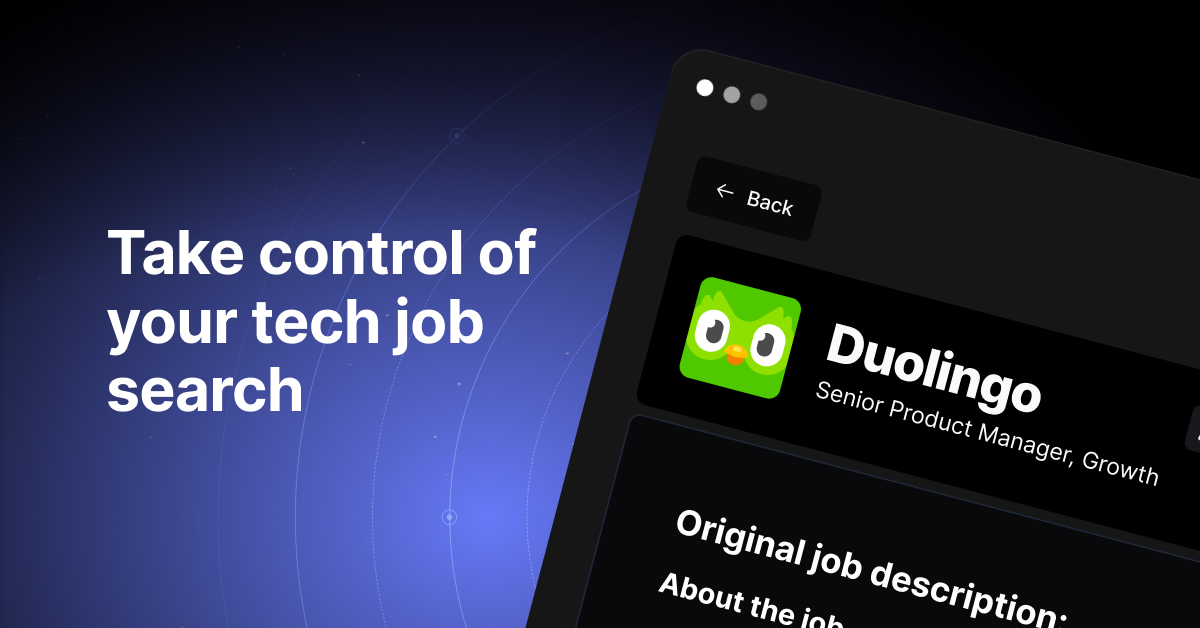