String.equals versus ==
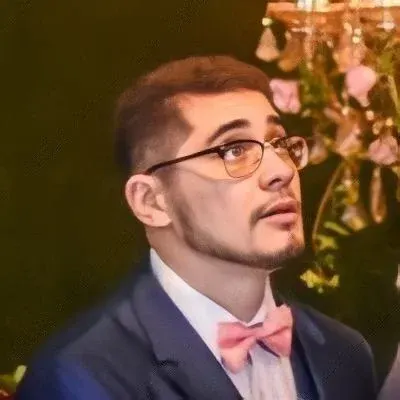
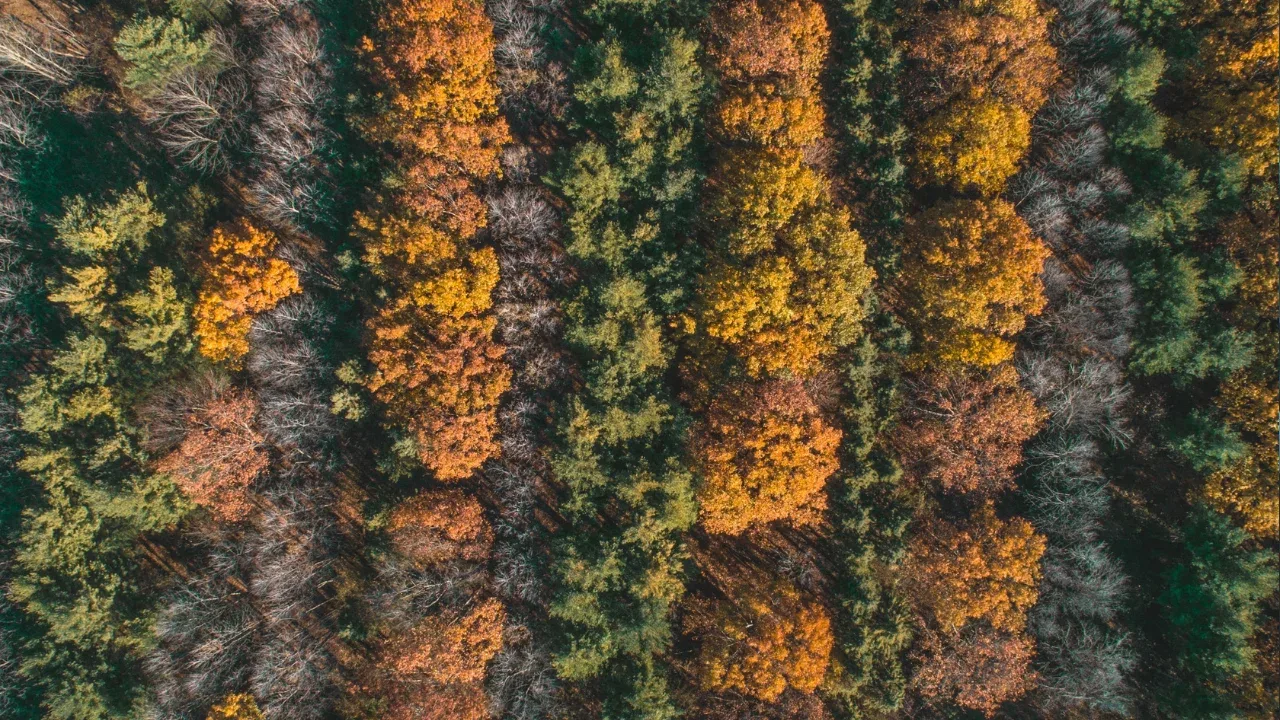
String.equals versus ==: Understand the Difference and Avoid Common Pitfalls
Have you ever encountered a scenario where your string comparison code doesn't work as expected? 🤔 Maybe you've come across the confusion between using the String.equals()
method and the ==
operator. Understanding the difference between these two can help you avoid common pitfalls and ensure your code functions correctly.
Let's dive into the details and explore this question in more depth. 💡
The Problem: Comparing Strings in Java
Consider the code snippet below:
public static void main(String...aArguments) throws IOException {
String usuario = "Jorman";
String password = "14988611";
String strDatos = "Jorman 14988611";
StringTokenizer tokens = new StringTokenizer(strDatos, " ");
int nDatos = tokens.countTokens();
String[] datos = new String[nDatos];
int i = 0;
while (tokens.hasMoreTokens()) {
String str = tokens.nextToken();
datos[i] = str;
i++;
}
if ((datos[0] == usuario)) {
System.out.println("WORKING");
}
}
The intent of this code is to compare datos[0]
(the first element in the array) with usuario
. If they match, it should print "WORKING". However, when you run this code, you may notice that "WORKING" is not printed, even if datos[0]
and usuario
have the same value.
The Explanation: String.equals() versus == Operator
In Java, strings are objects, not primitive types. This means that when comparing strings, you should treat them as objects and use the equals()
method instead of the ==
operator.
The equals()
method compares the content of the strings, whereas the ==
operator compares the references. When using ==
, you are checking if two string variables refer to the same memory address. On the other hand, equals()
compares the actual characters in the strings.
So, in our code snippet, using ==
to compare datos[0]
and usuario
will not work as expected, since they are different objects in memory, even if they have the same value.
The Solution: Using String.equals() for String Comparison
To fix the problem and ensure accurate string comparison, you should replace ==
with the equals()
method. Here's an updated version of the code:
if (datos[0].equals(usuario)) {
System.out.println("WORKING");
}
By using equals()
, you are comparing the actual characters in the strings, ensuring accurate results.
Conclusion and Call-to-Action
String comparison can be tricky, but understanding the difference between String.equals()
and the ==
operator is crucial. Remember to use equals()
when you want to compare the content of strings, as it compares characters, not just memory addresses.
Next time you encounter a string comparison issue, ask yourself if you're using the correct approach. If not, adjust your code accordingly.
Do you have any tips or tricks for handling string comparison in Java? Let us know in the comments below! 😄💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
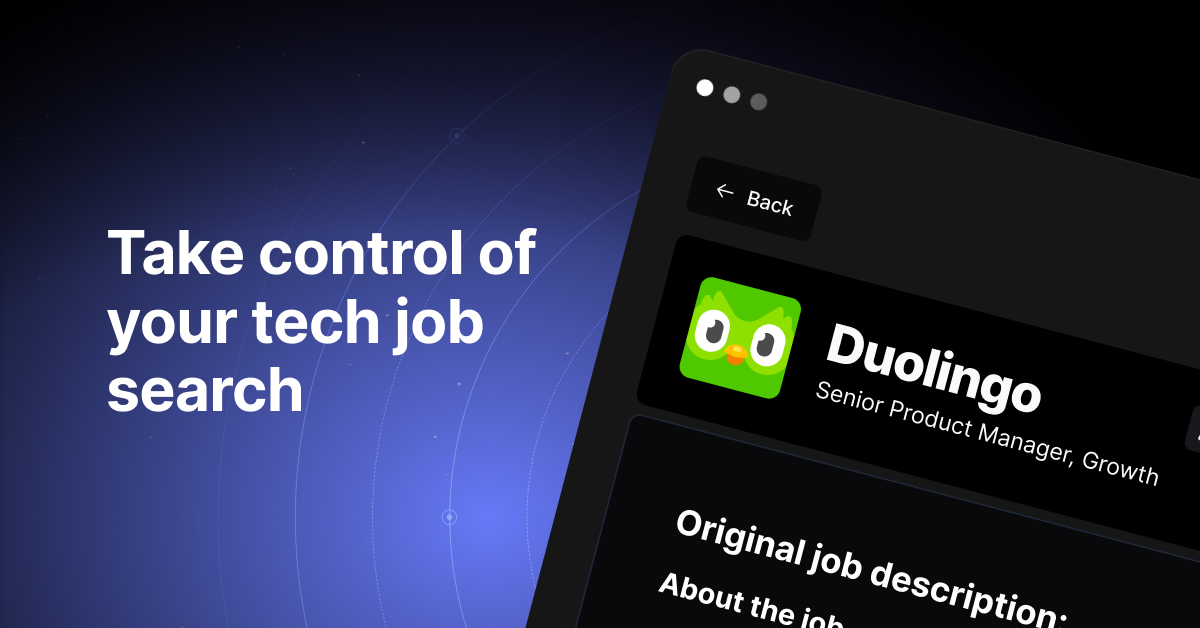