@try - catch block in Objective-C
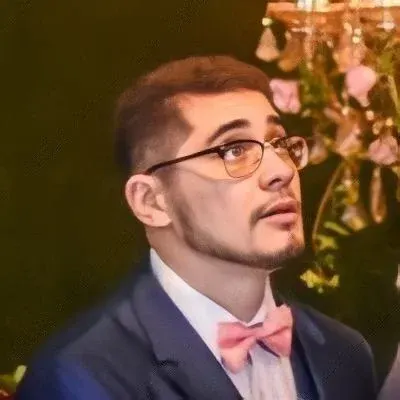
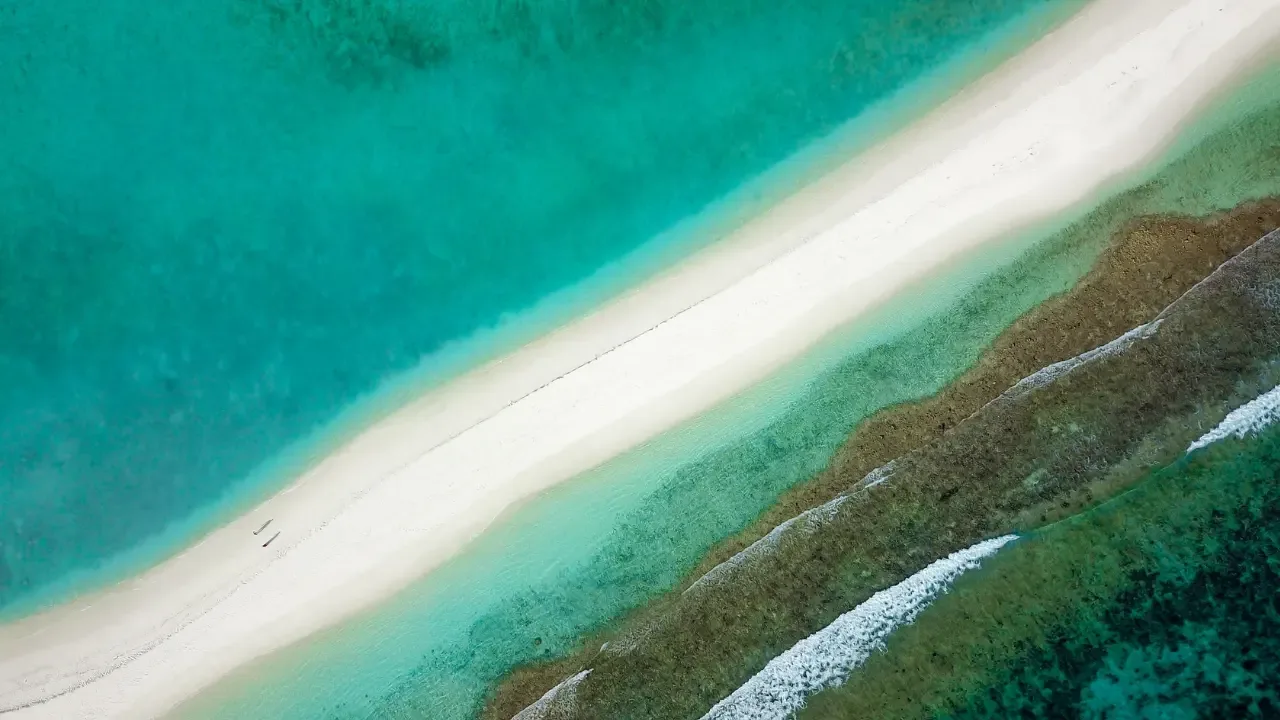
Understanding @try-catch block in Objective-C
š Hello tech enthusiasts! Today, let's dive deep into the mysterious world of @try-catch blocks in Objective-C. We'll address a common issue faced by developers and provide you with easy solutions. So buckle up and let's get started! š
The Problem: @try block not catching exceptions
š Have you ever encountered a situation where an exception is thrown in your Objective-C code, but it doesn't get caught by the @try block as expected? š± The code snippet given above might have resonated with your experience.
š A common misconception is that the @try block should catch any exceptions thrown within its scope. However, there are scenarios where the app crashes despite having an @try block in place. Let's demystify this, shall we?
The Explanation: Exceptions and Uncaught Exceptions
š In Objective-C, exceptions are a way to handle runtime errors or exceptional conditions that occur during the execution of your code. Exceptions are thrown when something unexpected happens and need to be dealt with appropriately.
𤯠The crucial point to note is that not all exceptions in Objective-C are meant to be caught. Some exceptions are intended to crash the app, signaling a severe error that cannot be recovered from. These are called "uncaught exceptions."
1ļøā£ In the code snippet provided, characterAtIndex:
is used to access a character at an index that is out of range. This action throws an exception of type NSRangeException
.
2ļøā£ Since NSRangeException
is an uncaught exception, it is not handled by the @try block, resulting in a crash.
3ļøā£ The @catch block is designed to catch expected exceptions that you explicitly handle, typically those derived from NSException
.
The Solution: Catching Expected Exceptions
ā To handle exceptions thrown by Objective-C code effectively, it's crucial to differentiate between expected exceptions that you should catch and uncaught exceptions that are meant to crash the app.
1ļøā£ Replace the @catch block's parameter type from NSException
to NSError
or NSException
subclasses you expect to catch. In this case, we can replace NSException
with NSRangeException
.
@try {
[test characterAtIndex:6];
}
@catch (NSRangeException *exception) {
NSLog(@"Caught Exception: %@", exception);
}
@finally {
NSLog(@"Finally block");
}
2ļøā£ By catching the expected NSRangeException
, the app won't crash, and you'll be able to handle the exceptional condition gracefully.
The Call-to-Action: Engage and Level Up Your Objective-C Skills!
š Congratulations, you now have a solid understanding of how @try-catch blocks work in Objective-C! šŖ It's time to put your new knowledge into practice.
š Experiment with different exception types and try-catch block configurations in your code. Understand when to catch specific exceptions and when to let uncaught exceptions crash the app.
š Share your experiences and any additional tips you have for working with @try-catch blocks in Objective-C in the comments below. Let's learn and grow together! š±
š¢ If you found this blog post helpful, don't forget to share it with your fellow developers. Let's spread the knowledge and make coding in Objective-C a seamless experience for everyone! š¤©
Happy coding! āØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
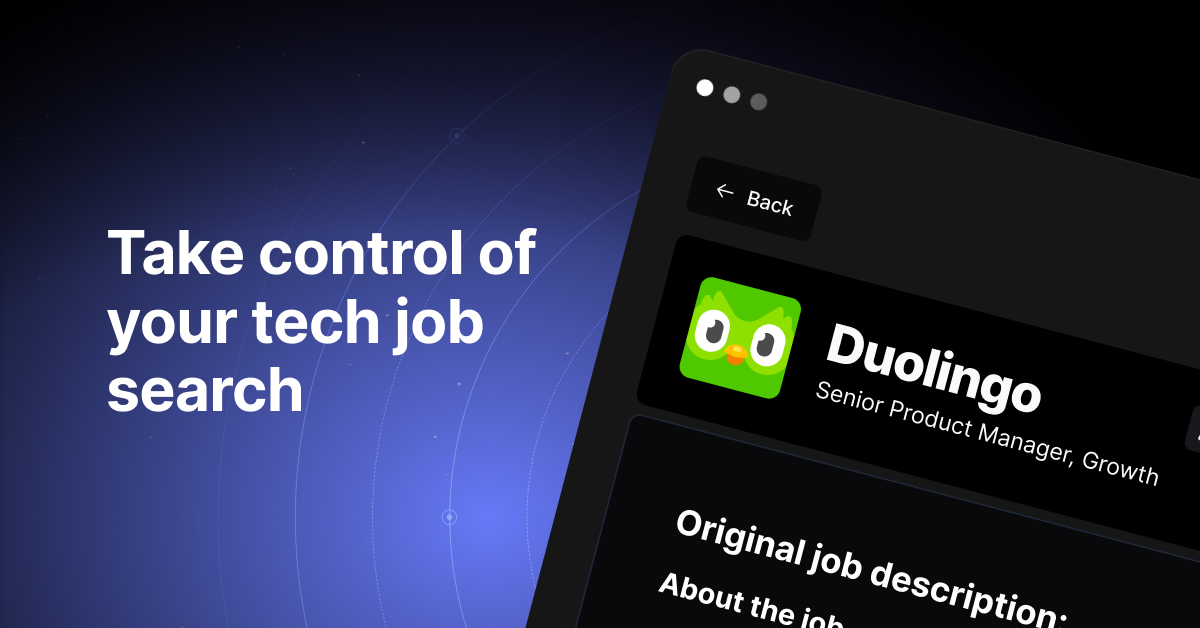