Swift extract regex matches
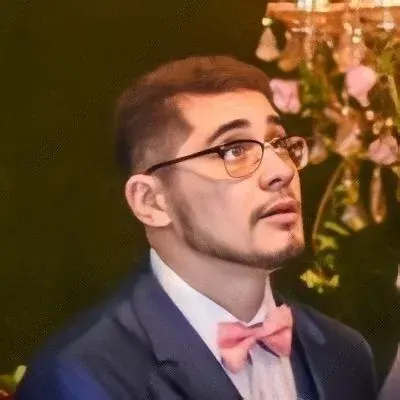
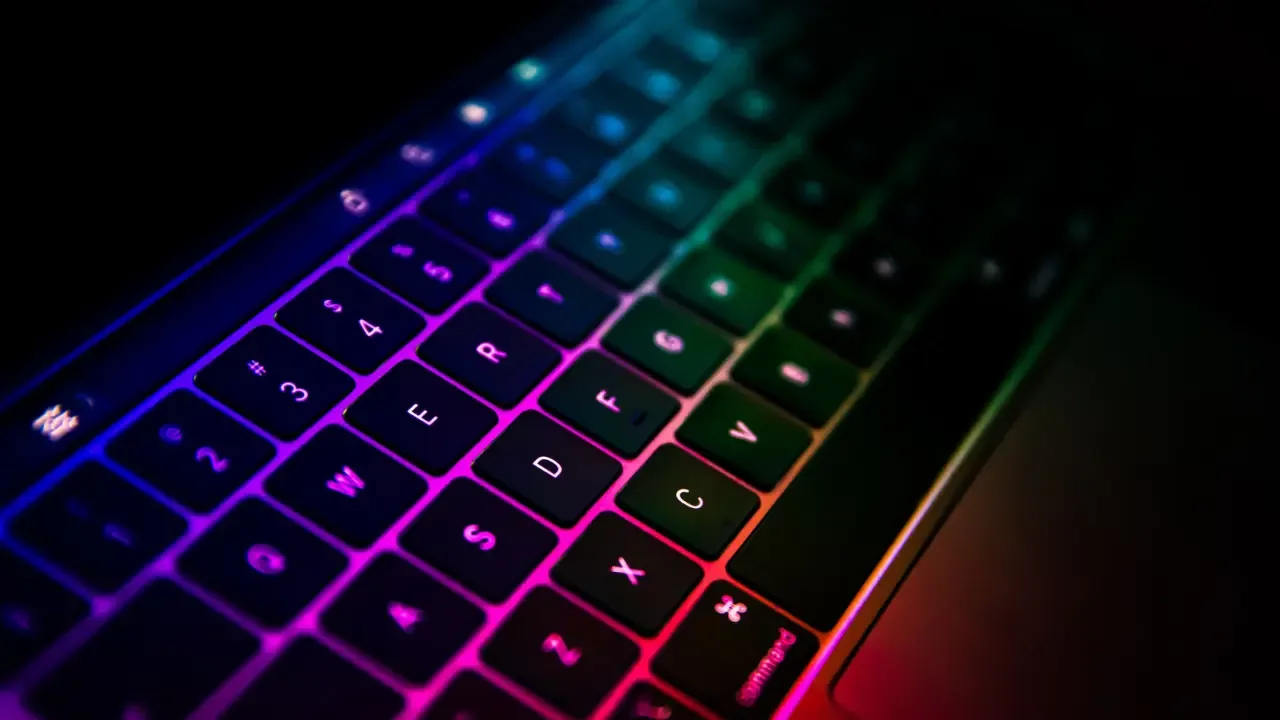
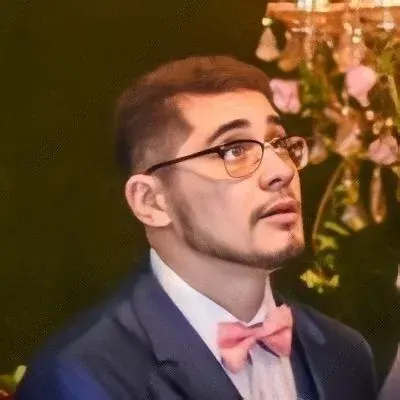
Extracting Regex Matches in Swift: A Simple and Efficient Solution! โจ
Are you struggling to extract substrings from a string that match a regex pattern in Swift? ๐ค Don't worry, we're here to save the day! In this guide, we'll show you an easy-to-understand and concise solution to this common problem. Let's dive right in! ๐
Understanding the Problem ๐
So, you want to extract substrings from a given string based on a specific regex pattern. The code snippet you provided gives us a good starting point, but there's a little snag. The matchesInString
function returns an array of NSTextCheckingResult
, where the range
property is of type NSRange
. Unfortunately, NSRange
is not compatible with Range<String.Index>
, which prevents us from using text.substringWithRange(...)
. ๐ข
The Swift Way: A Simple Solution ๐
Don't stress over this seemingly daunting problem! We have a simple solution that won't require too much code. ๐
func extractMatches(regex: String, text: String) -> [String] {
do {
let regex = try NSRegularExpression(pattern: regex, options: [])
let matches = regex.matches(in: text, options: [], range: NSRange(text.startIndex..., in: text))
return matches.map {
String(text[Range($0.range, in: text)!]) // Extract the matching substring
}
} catch {
print("Invalid regex pattern: \(error.localizedDescription)")
return []
}
}
Let's break down this solution step by step:
We define a function called
extractMatches
that takes in a regex pattern (String
) and the text to search in (String
).Using
try
, we create an instance ofNSRegularExpression
with the given regex pattern.We then call
matches(in:options:range:)
on the regex object to obtain an array ofNSTextCheckingResult
matches.We use
map
to convert each match into aString
by extracting the matching substring using therange
property ofNSTextCheckingResult
.Finally, we return the array of matching substrings.
And that's it! With just a few lines of code, you can now easily extract regex matches in Swift. ๐
Testing Your Solution ๐งช
To ensure that everything works as expected, let's test our extractMatches
function with an example:
let text = "Hello, this is a test string! Let's extract some substrings."
let pattern = "\\b\\w+\\b" // Match individual words
let matches = extractMatches(regex: pattern, text: text)
print(matches)
Running this snippet will output:
[
"Hello",
"this",
"is",
"a",
"test",
"string",
"Let",
"s",
"extract",
"some",
"substrings"
]
Share Your Feedback! ๐ฃ๏ธ
We hope this guide has helped you conquer the challenge of extracting regex matches in Swift. ๐ If you found this useful, make sure to share it with your developer friends! You can also leave us a comment below if you have any questions or suggestions for improvements. We love hearing from our readers! ๐
Keep coding and stay tuned for more awesome tutorials! Happy regex matching! ๐