Simplest way to throw an error/exception with a custom message in Swift?
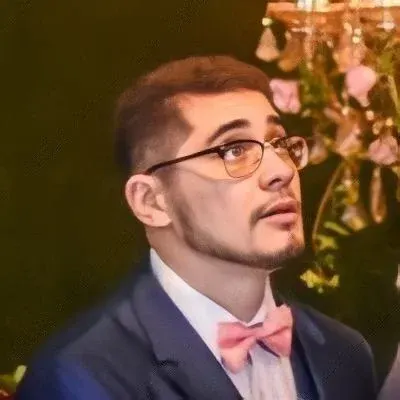
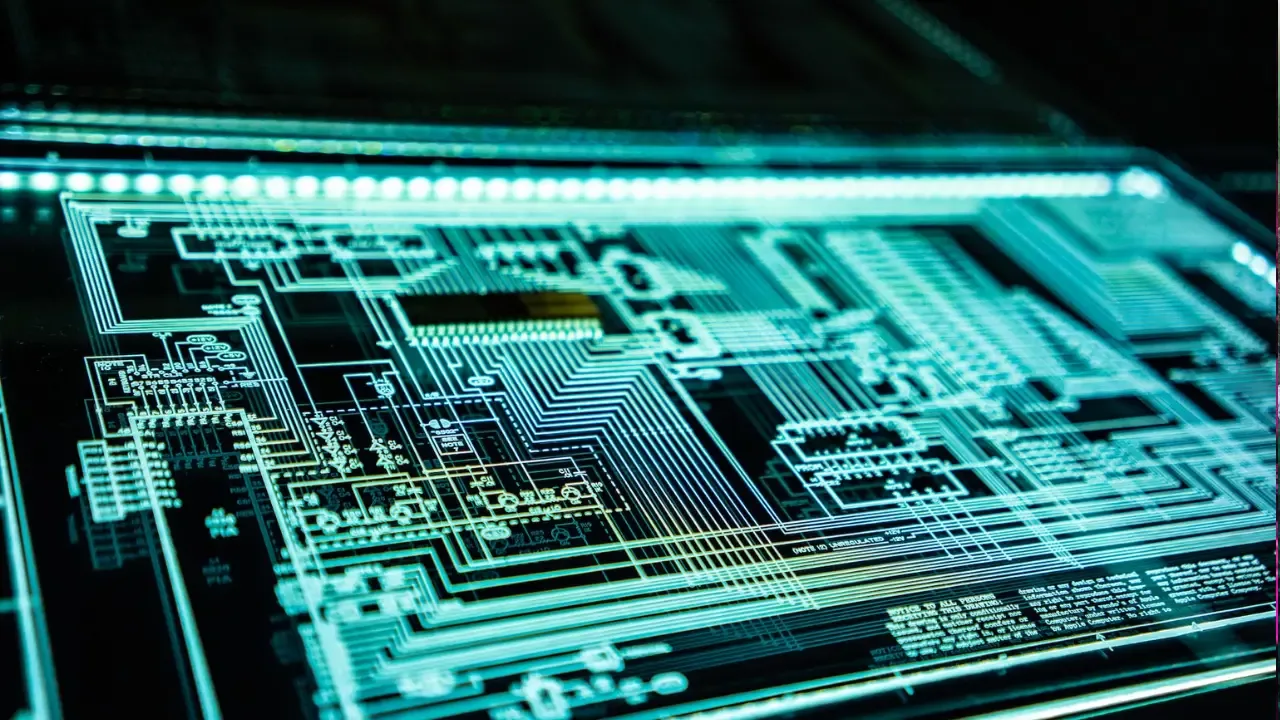
Simplest way to throw an error/exception with a custom message in Swift? 😕
Are you a Swift developer struggling to find a way to throw a runtime exception with a custom message in your code? You're not alone! Many developers coming from other programming languages are familiar with this feature and want to replicate it in Swift.
I understand your frustration. While Swift does not have the exact same functionality as Java, where you can simply throw a RuntimeException with a custom message, there are alternative solutions that can achieve a similar outcome. Let's explore some easy solutions to this problem! 🚀
Solution 1: Use the NSException
class
One option is to leverage the power of Objective-C interoperability in Swift by using the NSException
class. This class allows you to create and throw exceptions with custom messages. Here's an example of how you can use it:
// Import the Foundation framework to access the NSException class
import Foundation
// Throw an exception with a custom message
NSException.raise(NSExceptionName(rawValue: "CustomException"), format: "A custom message here", arguments: getVaList([]))
While this approach achieves the desired outcome, it's important to note that throwing exceptions using NSException
is not considered idiomatic Swift. It's better suited for situations where you need to interoperate with Objective-C code or handle legacy codebases.
Solution 2: Utilize a Custom Error Type
Swift encourages the use of a more structured approach to error handling, using custom types that conform to the Error
protocol. Although you mentioned not wanting to define enums for every type of error you throw, this approach provides better type safety and future-proofing. Here's how you can do it:
// Define a custom error enum
enum CustomError: Error {
case runtimeException(String)
}
// Throw an error instance with a custom message
throw CustomError.runtimeException("A custom message here")
By defining a custom error enum, you gain more control over the types of errors in your codebase. You can easily switch on error cases, handle them specifically, or even propagate them up the call stack without any ambiguity.
🌟 Give it a try!
Now that you have learned two different approaches to throwing errors with custom messages, it's time to experiment with them in your own code! Test them out and see which one fits best with your coding style and project requirements.
Remember, error handling is an essential part of writing robust and reliable code. By embracing the Swift way of handling errors, your code will become more maintainable and easier to collaborate on.
If you have any questions or face any challenges during the implementation, feel free to reach out to us in the comments below. We're here to help! 😊
Happy coding! 👩💻👨💻
*[Swift]: A powerful and intuitive programming language for macOS, iOS, watchOS, and tvOS. *[enum]: An enumeration, a common data type in Swift capable of defining a group of related values. *[idiomatic]: Following the accepted conventions and best practices of a particular language or community.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
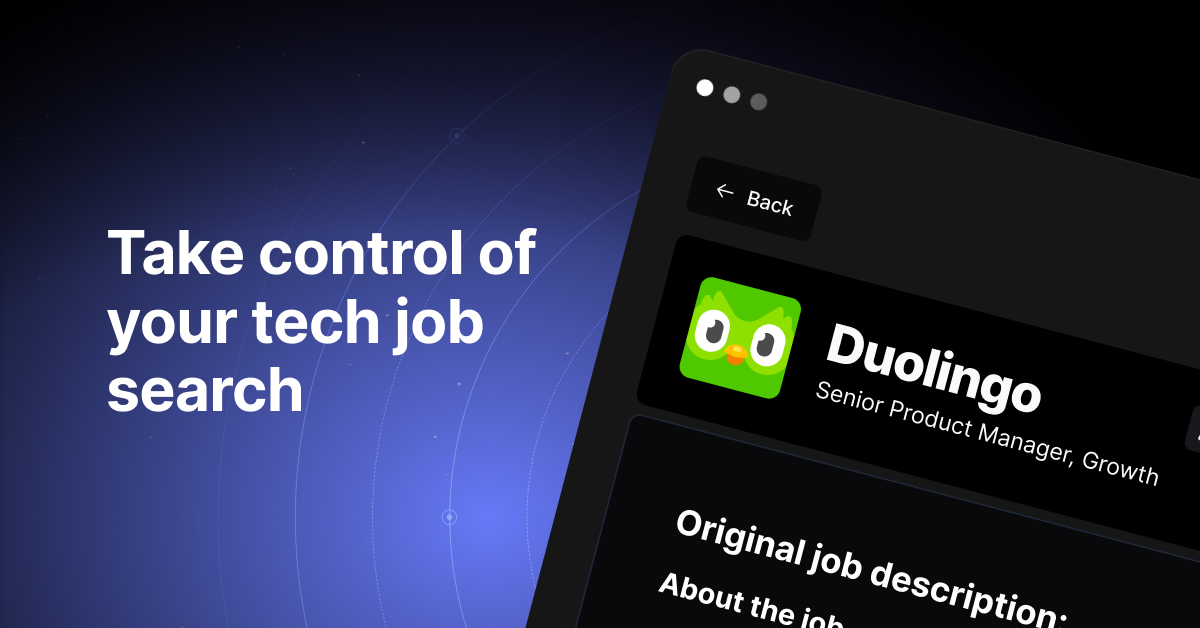