performSelector may cause a leak because its selector is unknown
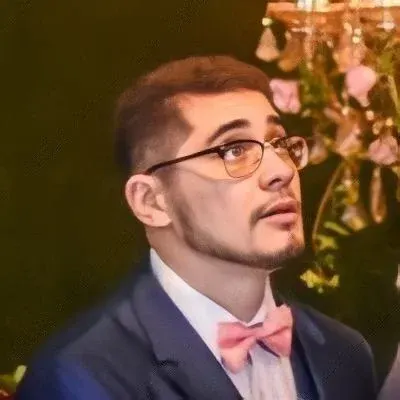

📝🌟Blog Post: Why performSelector may cause a leak and how to fix it! 🛠️✨
Hey there, tech enthusiasts! 👋 Are you eager to understand the mysterious warning message from the ARC compiler that says "performSelector may cause a leak because its selector is unknown"? 🤔 Fear not! In this blog post, we'll unravel the mystery behind this warning, explore common issues, provide easy solutions, and empower you to fix it. Let's dive in! 🚀
Understanding the Warning ⚠️
So, you're encountering this warning message, huh? That's no fun! Let's figure out why it's happening and how it relates to memory leaks. 🤔
By using the performSelector
method like this:
[_controller performSelector:NSSelectorFromString(@"someMethod")];
you're invoking a method dynamically at runtime using a selector that is not known to the compiler. The issue arises because the compiler can't verify if the selector exists or not. This uncertainty can potentially lead to memory leaks if not handled properly. 😱
Why can it cause a leak? 💦
When you use performSelector
, the compiler can't perform its usual static analysis to optimize memory management. As a result, the compiler doesn't know whether the invocation creates a strong reference cycle or not, thus the warning. 😓
Imagine a scenario where the selector triggers a method that creates a strong reference to self
. If self
also strongly references the object that triggers the selector (e.g., a timer), a retain cycle is formed, leading to memory leaks. 💔
Simple Solutions to Tackle the Warning 🛠️
Now that we understand the problem, it's time to look at some simple solutions to prevent memory leaks and get rid of the warning! 🎉
1. User Interaction or Timer
If you're using performSelector
in response to user interaction or via a timer, switch to a safer alternative:
// Instead of
[_controller performSelector:selector];
// Use
[_controller performSelector:selector withObject:nil afterDelay:0];
By using a delay of 0
, the invoked method will be executed on the next run loop iteration after the control flow has been released. This avoids the creation of potential retain cycles. 👍
2. Delegate Pattern
If you're using performSelector
to invoke a delegate method, update your code to use the delegate pattern. For example:
// Instead of
[_controller performSelector:selector withObject:parameter];
// Use
if ([_delegate respondsToSelector:selector]) {
[_delegate performSelector:selector withObject:parameter];
}
By explicitly checking if the delegate responds to the selector before invoking it, you ensure that the method is only called when implemented by the delegate. 🙌
3. Blocks or Invocations
Another approach to avoid the warning is by utilizing blocks or NSInvocation. This allows you to encapsulate the logic and safely pass around code blocks.
Blocks example:
// Instead of
[_controller performSelector:selector];
// Use
((void (^)(id))selector)(_controller);
NSInvocation example:
// Instead of
[_controller performSelector:selector];
// Use
NSMethodSignature *methodSignature = [_controller methodSignatureForSelector:selector];
NSInvocation *invocation = [NSInvocation invocationWithMethodSignature:methodSignature];
[invocation setSelector:selector];
[invocation invokeWithTarget:_controller];
Both options provide a safer way to execute dynamic methods while avoiding the warning. It's a win-win! 💪
Take Action! Engage and Share! 💬📢
Congrats, my friend! You've made it to the end of this exciting blog post. We've explored why performSelector
can cause a leak, learned its relationship with memory management, and most importantly, equipped ourselves with easy solutions. Awesome! 🎉
Now, it's your turn to take action! Try implementing the suggested solutions in your code and let us know how it goes in the comments below. Have you encountered different approaches to tackle this problem? Share your thoughts!
Remember, knowledge is meant to be shared. If you found this blog post helpful, spread the word by sharing it with your fellow developers. Together, we can conquer these coding challenges! 🌍💻
Thanks for reading, and until next time, happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
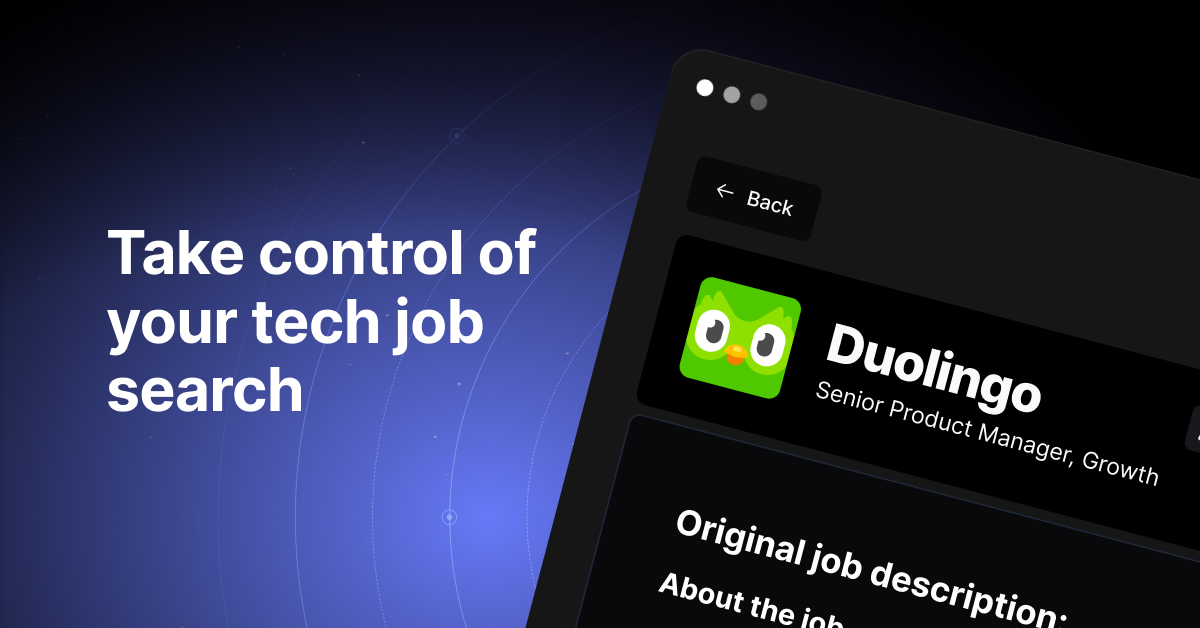