How to programmatically send SMS on the iPhone?
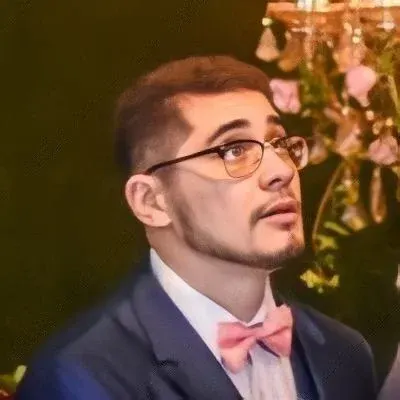
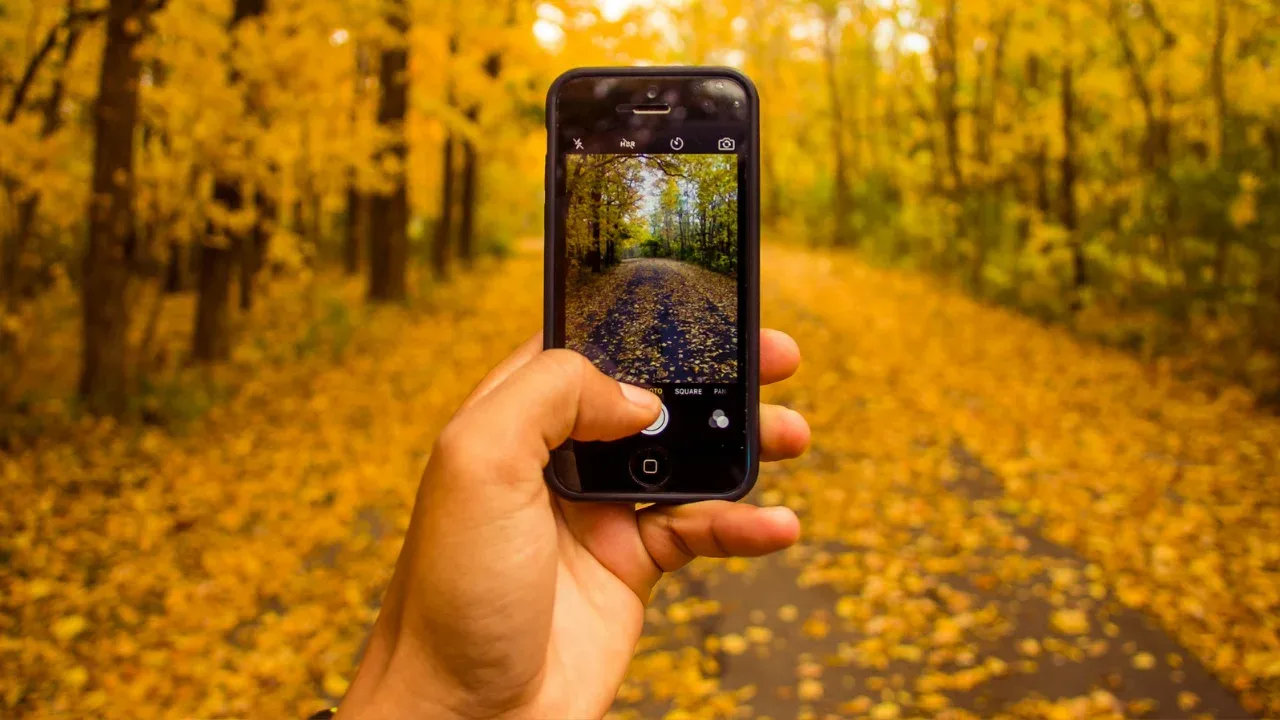
📱📨 Sending SMS on the iPhone: A Complete Guide 📝🚀
Have you ever wondered if you can programmatically send an SMS on your iPhone? 🤔 Whether you want to automate messaging for your app or develop a messaging feature within your application, this guide will help you navigate through the process smoothly. 📲💨
So, let's dive into the world of iOS development and learn how to programmatically send SMS on the iPhone using the official SDK and Cocoa Touch! 🎉💻
Understanding the Problem
First things first: Yes, it is indeed possible to send an SMS programmatically on the iPhone. 🙌 However, there are a few things you need to keep in mind before diving deep into the coding realm.
Apple's official SDK (Software Development Kit) and Cocoa Touch framework provide developers with the necessary tools to interact with the iPhone's messaging capabilities.
You need to have the user's consent to send SMS messages. Respect their privacy and request permission before sending any messages programmatically.
Easy Solutions Await 🎁
Now that we have a clear understanding of the prerequisites, let's explore a couple of easy solutions to help you accomplish your task.
Solution 1: MFMessageComposeViewController
One of the simplest ways to send an SMS programmatically on the iPhone is by utilizing the power of MFMessageComposeViewController
. This framework provides a pre-configured messaging interface that enables users to compose and send SMS messages without leaving your app's context.
Here's an example of how you can use MFMessageComposeViewController
:
import MessageUI
func sendSMS() {
if MFMessageComposeViewController.canSendText() {
let composeVC = MFMessageComposeViewController()
composeVC.messageComposeDelegate = self
composeVC.recipients = ["1234567890"]
composeVC.body = "Hello, friend! 📱 Have a great day!"
present(composeVC, animated: true, completion: nil)
}
}
extension YourViewController: MFMessageComposeViewControllerDelegate {
func messageComposeViewController(_ controller: MFMessageComposeViewController, didFinishWith result: MessageComposeResult) {
dismiss(animated: true, completion: nil)
}
}
Remember to add the necessary import statement for MessageUI
and declare conformance to the MFMessageComposeViewControllerDelegate
. This will ensure that you can handle the delegate methods when the user finishes composing the message.
Solution 2: Utilize SMS Gateways or APIs
In some cases, you might need more control or customization over the SMS messaging process. In such scenarios, you can consider integrating SMS gateways or APIs into your app.
SMS gateways and APIs allow you to send SMS messages programmatically by utilizing their services. They often provide you with an interface to make HTTP requests to their servers, allowing you to send messages with specific parameters.
Examples of popular SMS gateways and APIs include Twilio, Nexmo, and Plivo. Each has its own documentation and usage guidelines, so make sure to explore their resources and choose one that fits your requirements.
Empowering the Developer Community 💪🌐
By following the solutions mentioned above, you will be well-equipped to send SMS messages programmatically on your iPhone. 🚀
Share your experiences, insights, and any struggles you encountered along the way in the comments section below. Let's empower and learn from each other! 🤝
Remember, always respect user privacy and seek permission before sending any SMS messages programmatically.
Happy coding! 🎉💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
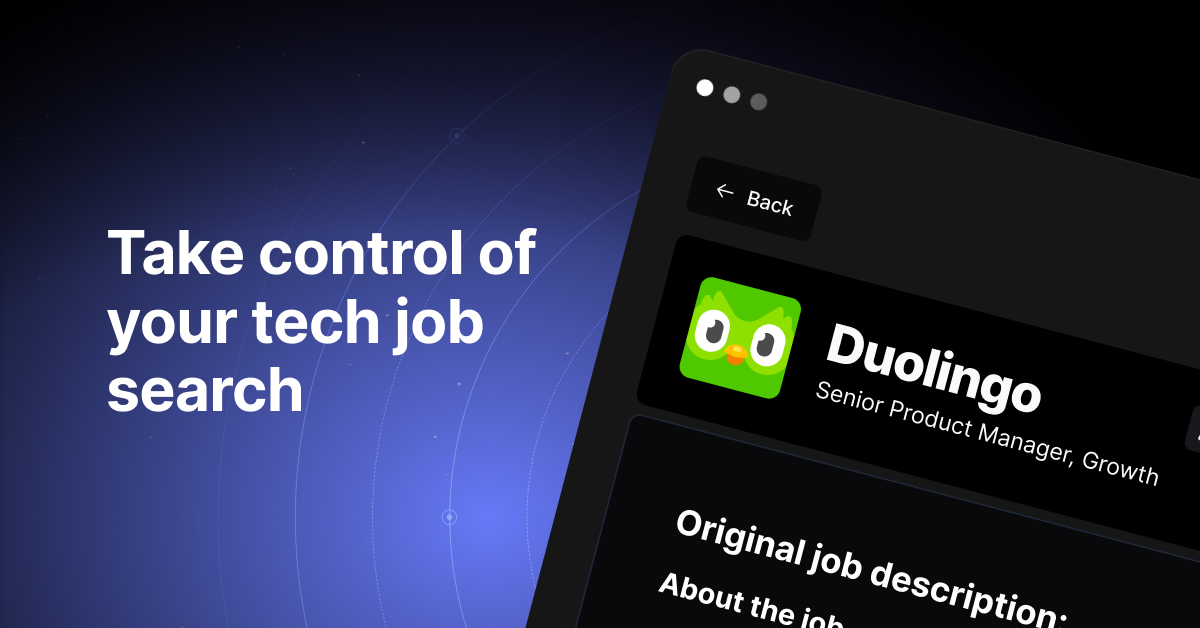