How do you create a Swift Date object?
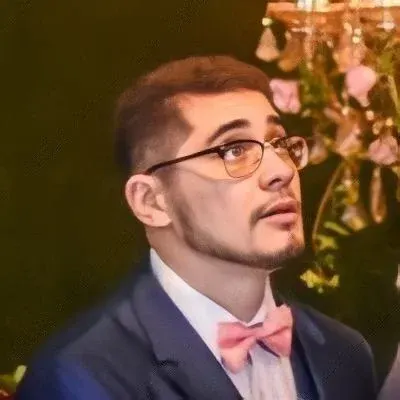
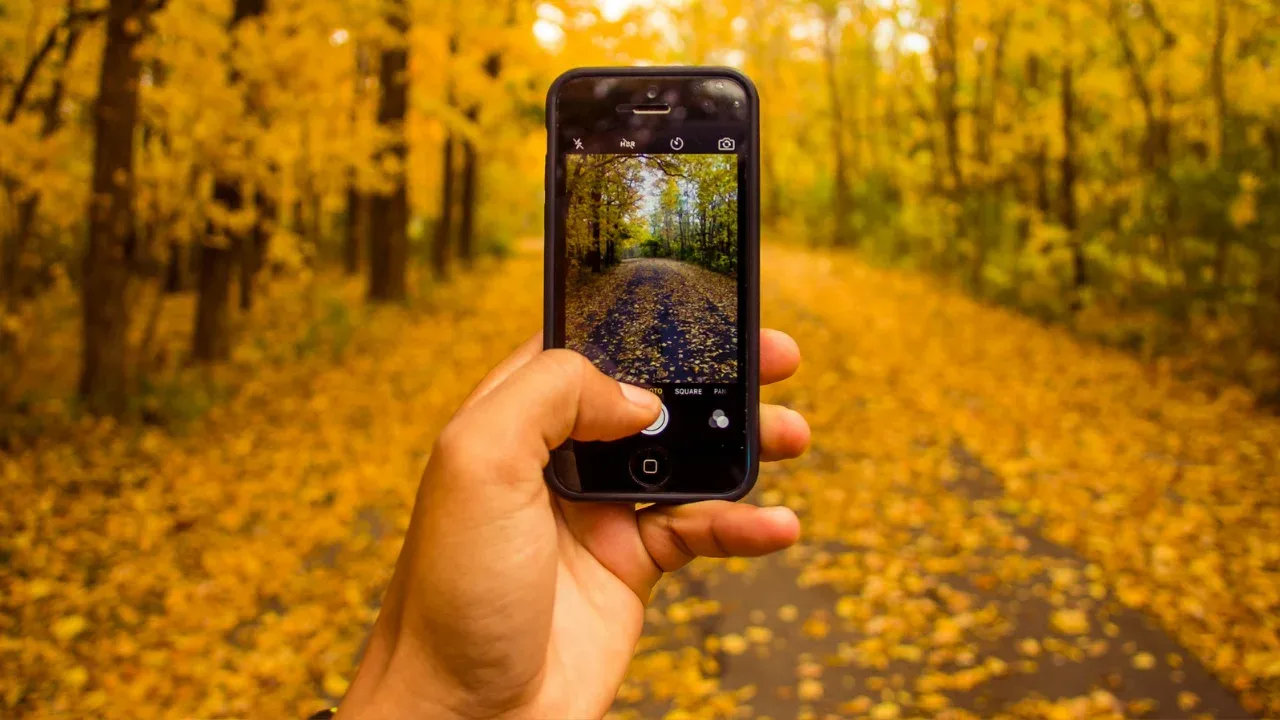
📅 Creating a Swift Date Object: A Handy Guide for Swift Developers
If you're a Swift developer trying to create a Date object from a date string in Xcode, you may have found yourself scratching your head. Well, worry no more! In this blog post, we'll dive deep into this topic and provide you with easy-to-follow solutions to create a Swift Date object. 💪
The Challenge: Creating a Swift Date Object
Let's say you have a date string like 2014-05-20
and you want to transform it into a Date object. In JavaScript, you could simply use new Date('2014-05-20')
. But in Swift, things work a bit differently.
Solution 1: Using DateFormatter
Swift provides us with a handy class called DateFormatter
that allows us to parse date strings and convert them into Swift Date objects. Here's how you can do it:
let dateString = "2014-05-20"
let dateFormatter = DateFormatter()
dateFormatter.dateFormat = "yyyy-MM-dd"
if let date = dateFormatter.date(from: dateString) {
// Now you have your Swift Date object!
} else {
// Date parsing failed. Handle the error gracefully.
}
In this solution, we create a DateFormatter
and set its dateFormat
property to match the format of our input date string. In our case, it's "yyyy-MM-dd"
. We then use the date(from: String)
method to parse the date string and obtain a Swift Date object. Simple, right? 😄
Solution 2: Using ISO8601DateFormatter
Starting from Swift 4, we have an even easier way to create Date objects from ISO 8601 strings. The ISO8601DateFormatter
class is designed specifically for parsing and formatting dates following the ISO 8601 standard.
let dateString = "2014-05-20"
let dateFormatter = ISO8601DateFormatter()
if let date = dateFormatter.date(from: dateString) {
// Voila! Your Swift Date object is ready.
} else {
// Oops! Date parsing failed. Handle the error gracefully.
}
With ISO8601DateFormatter
, you don't need to specify the date format explicitly. Swift will automatically recognize the ISO 8601 format and convert it into a Date object. 👍
Call-To-Action: Share Your Experience
Creating a Swift Date object doesn't have to be a headache anymore! Give these solutions a try, and let us know your thoughts in the comments below. Have you encountered any other challenges while working with date objects in Swift? Share your experiences and solutions with the community. We'd love to hear from you! 🗣️
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
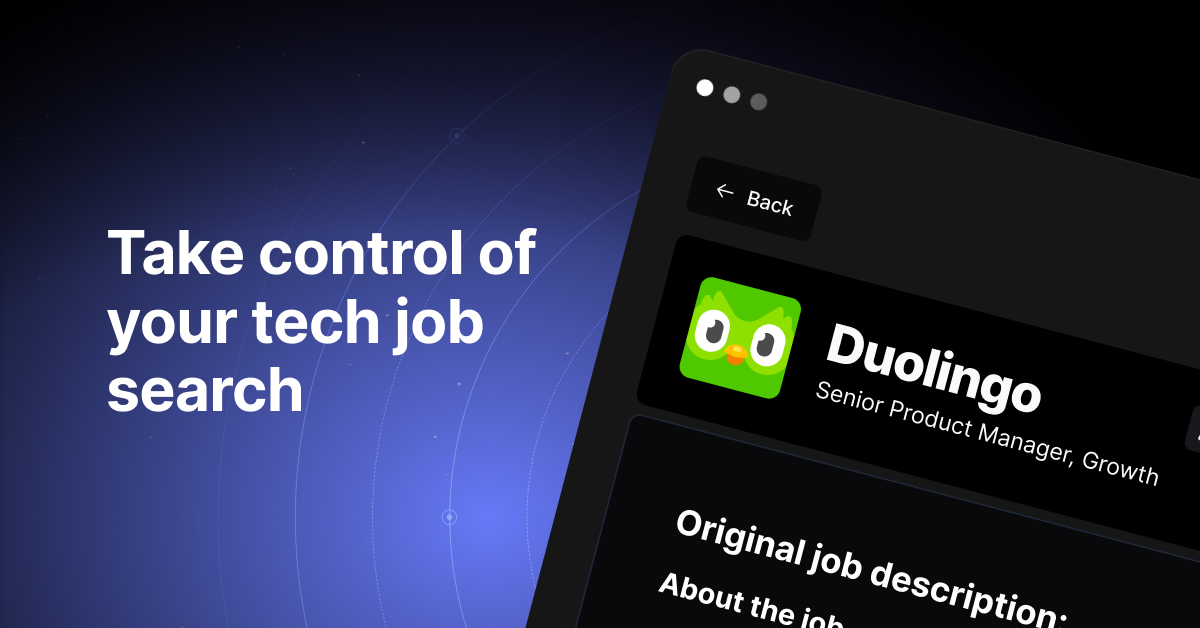