Check OS version in Swift?
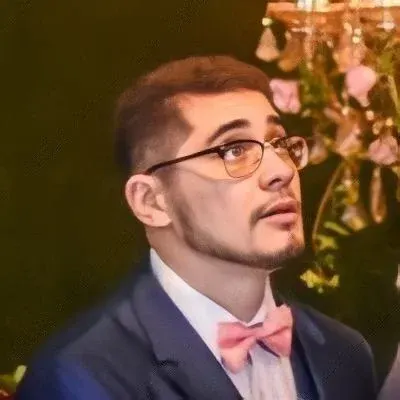
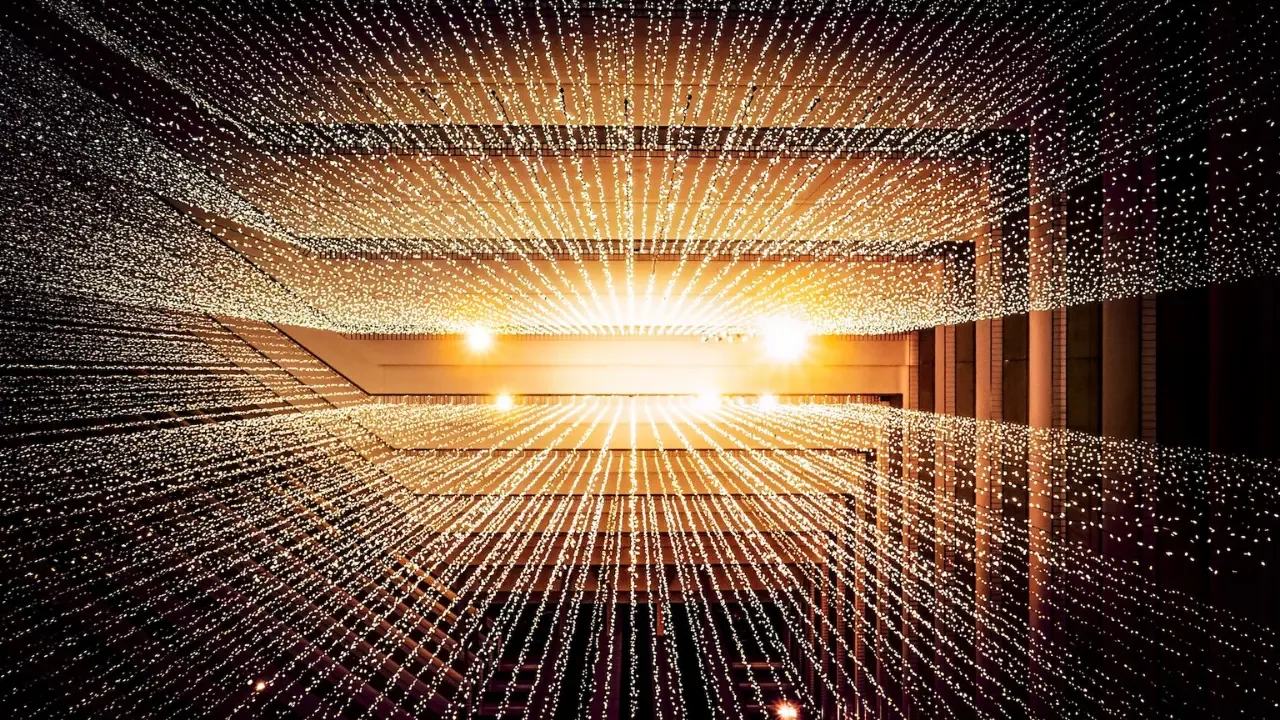
Checking the OS Version in Swift: A Complete Guide
š±š»
Are you looking to check the operating system version in Swift? š¤ You've come to the right place! In this blog post, we'll address common issues and provide easy solutions to help you get the information you need. So let's dive in! šŖš¼
The Code Snippet
First, let's take a look at the code snippet you mentioned:
var sysData: CMutablePointer<utsname> = nil
let retVal: CInt = uname(sysData)
This code is using the uname
function to retrieve system information. However, there are a couple of problems that you encountered. Let's tackle them one by one. š
Problem 1: sysData's Initial Value
In the given code snippet, sysData
is initialized as nil
. This leads to a value of -1 in retVal
, which indicates an error. To fix this, we need to allocate memory for sysData
before passing it to the uname
function.
Here's an updated code snippet that solves this problem:
var sysData = utsname()
let retVal: CInt = uname(&sysData)
By initializing sysData
as utsname()
without CMutablePointer
, we create an instance of utsname
and pass its memory address to the uname
function using the &
symbol.
Problem 2: Reading Information from sysData
Now that we have successfully obtained the system information, let's explore how to read it. The utsname
structure contains various fields such as sysname
, nodename
, release
, version
, and machine
. These fields represent the operating system's name, node name, release level, version, and hardware identifier, respectively.
Here's an example that demonstrates how to access and print each field:
let systemInfo = sysData
let sysName = String(bytes: &systemInfo.sysname, encoding: .utf8)?.trimmingCharacters(in: .controlCharacters)
let nodeName = String(bytes: &systemInfo.nodename, encoding: .utf8)?.trimmingCharacters(in: .controlCharacters)
let release = String(bytes: &systemInfo.release, encoding: .utf8)?.trimmingCharacters(in: .controlCharacters)
let version = String(bytes: &systemInfo.version, encoding: .utf8)?.trimmingCharacters(in: .controlCharacters)
let machine = String(bytes: &systemInfo.machine, encoding: .utf8)?.trimmingCharacters(in: .controlCharacters)
print("Operating System: \(sysName ?? "Unknown")")
print("Node Name: \(nodeName ?? "Unknown")")
print("Release Level: \(release ?? "Unknown")")
print("Version: \(version ?? "Unknown")")
print("Hardware Identifier: \(machine ?? "Unknown")")
In the above code snippet, we convert each field from a byte array to a string using the String(bytes:encoding:)
initializer in Swift. We also trim any control characters from the resulting strings using trimmingCharacters(in:)
method. Finally, we print out the information we retrieved.
Call to Action
Now that you have a complete understanding of how to check the OS version in Swift, why not try it out yourself? š Feel free to experiment, integrate it into your projects, and share your experience with us in the comments section below. We're excited to hear from you! šš¬
That's it for today's blog post. Don't forget to subscribe to our newsletter š© so you never miss an update and follow us on social media to stay connected. š„ Happy coding! š»š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
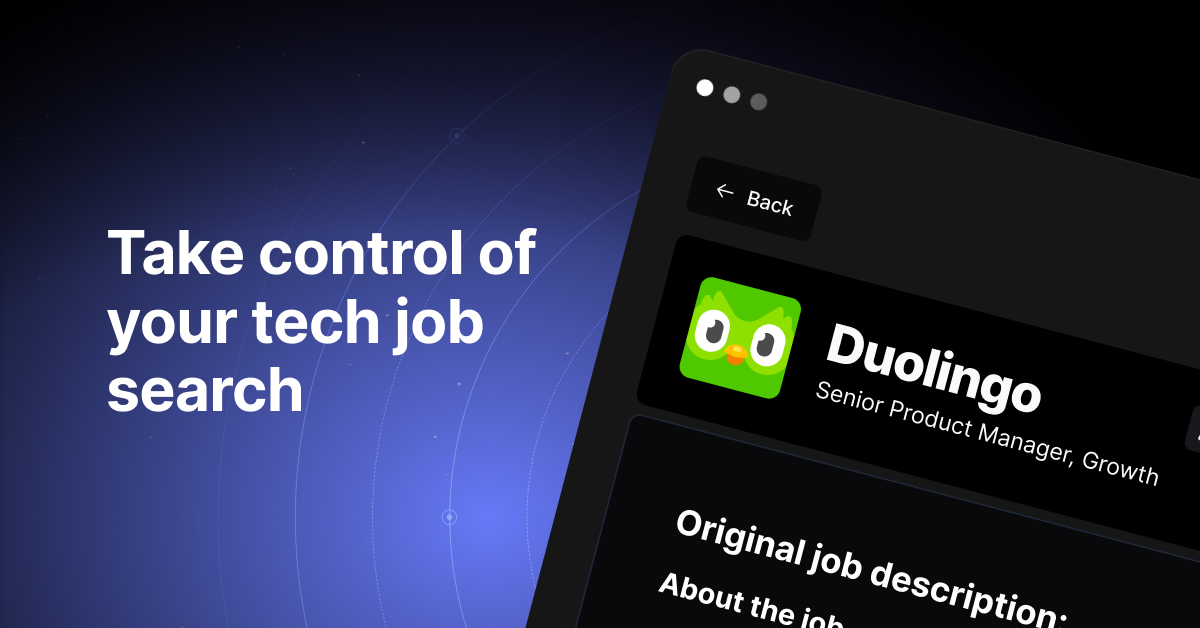