There are multiple heroes that share the same tag within a subtree
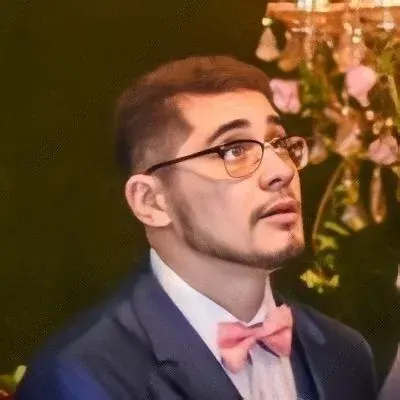
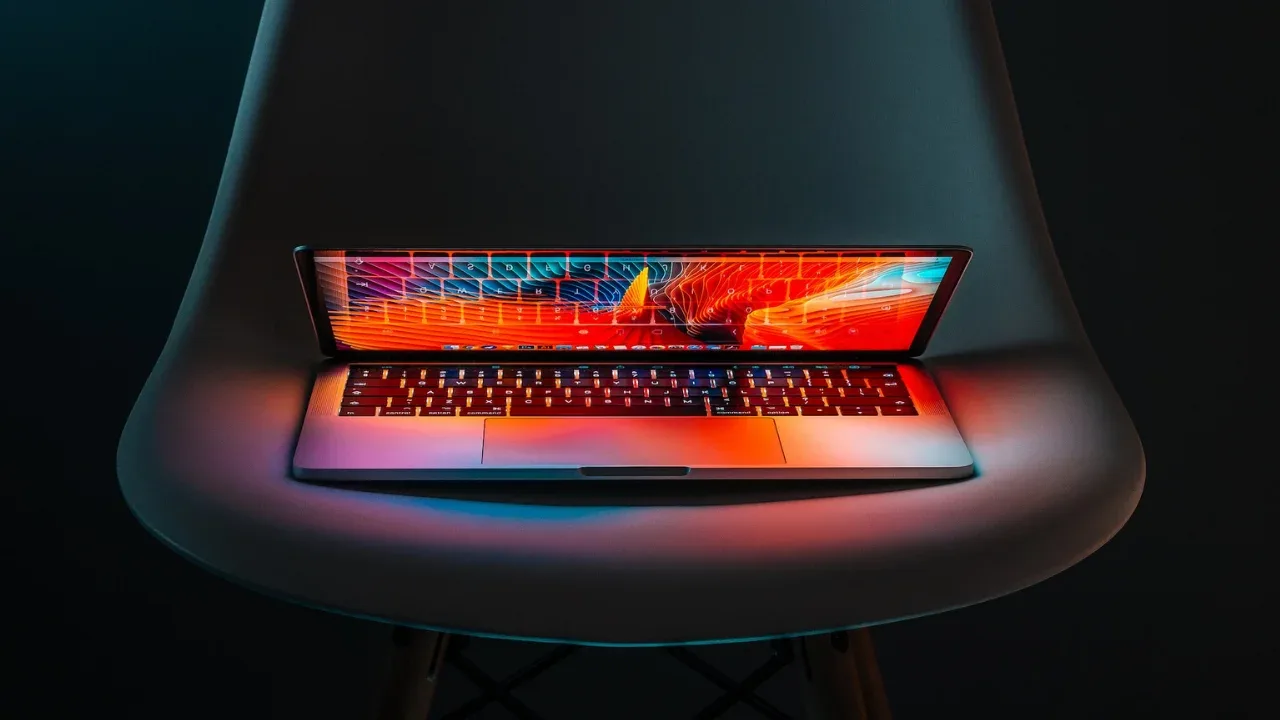
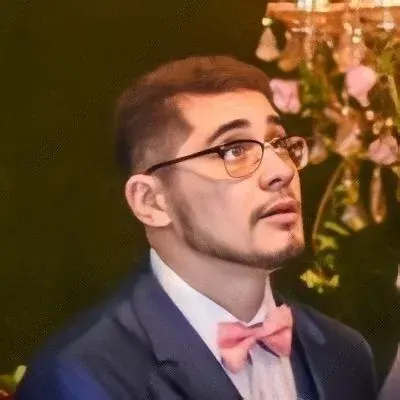
🔥📝 Fixing the "Multiple Heroes" Error in Flutter Routes 🔥📝
So, you're trying to navigate from one screen to another in your Flutter app using routes. However, when you hit the button to move to the specified route, you encounter the dreaded error:
Another exception was thrown: There are multiple heroes that share the same tag within a subtree.
Yikes! Don't worry, though. I'm here to help you understand and fix this issue. Let's break it down step by step.
🤔 Understanding the Error
The error message you see is pointing out that there are multiple heroes (typically widgets that are being animated) with the same tag within a subtree. In this case, the error specifically mentions a FloatingActionButton
tag.
A hero's tag needs to be unique within a subtree, meaning no two heroes can have the same tag. When you try to navigate to a new route, Flutter detects that there are multiple heroes with the same tag and throws an error to prevent conflicts during the animation.
💡 Quick Solution
To fix this issue, all you need to do is ensure that each hero in your app has a unique and non-null tag within its subtree. Let's take a look at your code to see where the problem might lie.
In the provided code snippet, there's a FloatingActionButton
in the NavigatorOne
class. Since this is the only FloatingActionButton
present, we can assume that its tag is causing the conflict.
To solve it, you can assign a unique tag to the FloatingActionButton
by using the Hero
widget's tag
property. Here's an example:
FloatingActionButton(
heroTag: 'uniqueTag',
onPressed: () {
Navigator.of(context).pushNamed('/second');
},
child: Text(' one 1'),
),
With this change, each FloatingActionButton
will have a unique tag within its subtree, preventing any conflicts when navigating between routes.
🚀 Supercharge Your Solution
While the quick solution works perfectly, it's always a good idea to take your coding game to the next level! Instead of hardcoding a static unique tag, you can make use of Flutter's UniqueKey
class to generate a truly unique tag for each hero.
Here's an improved example using UniqueKey
:
// Import the UniqueKey class
import 'package:flutter/foundation.dart';
//...
FloatingActionButton(
heroTag: UniqueKey(),
onPressed: () {
Navigator.of(context).pushNamed('/second');
},
child: Text(' one 1'),
),
By utilizing UniqueKey
, you can ensure that each FloatingActionButton
will always have a different and unique tag within its subtree. This approach is particularly useful when dealing with dynamic widget creation.
📢 Take Action
Now that you understand the issue and have the solution at hand, it's time to implement the fix in your code and get rid of the "Multiple Heroes" error!
Give it a try, and I'm confident you'll successfully navigate from one screen to another using routes without encountering any conflicts with heroes sharing the same tag.
If you have any questions or want to share your success, don't hesitate to leave a comment below. I'm here to help you out! Happy coding! 😄👨💻