Sizing elements to percentage of screen width/height
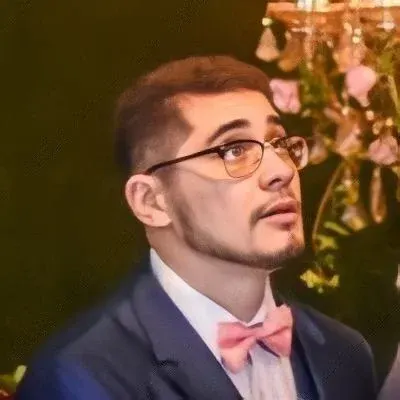
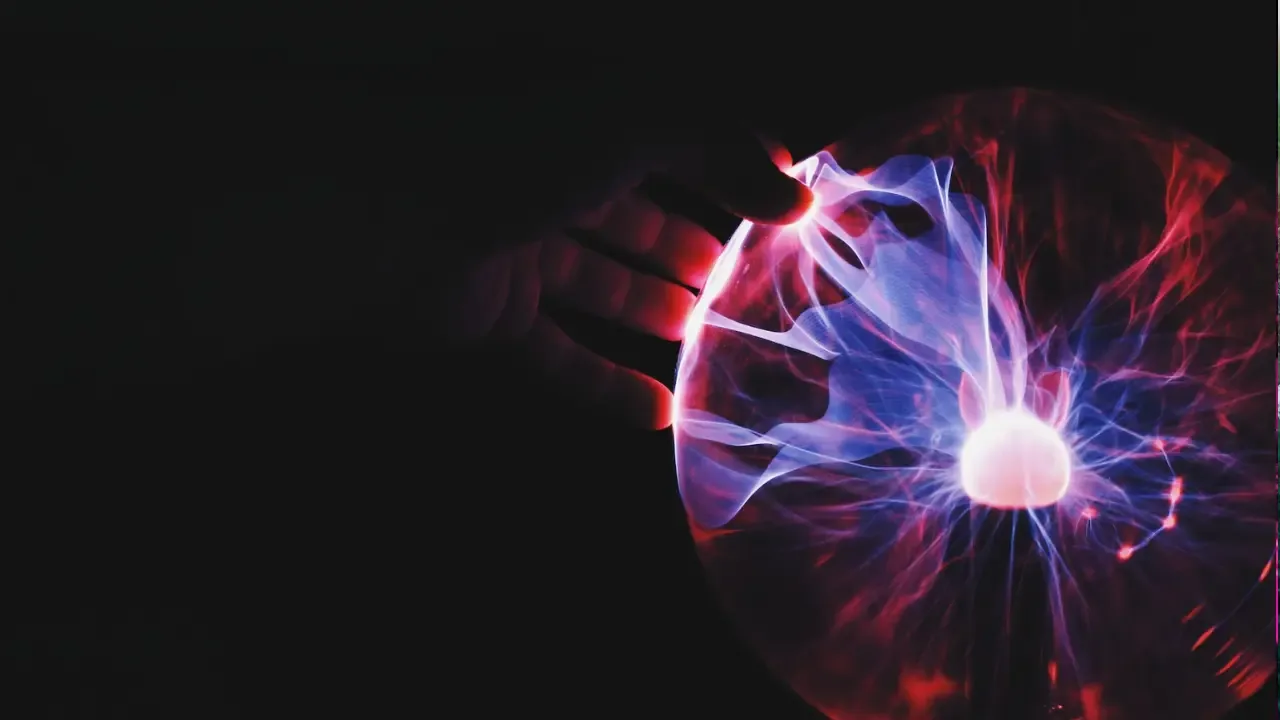
Sizing Elements to Percentage of Screen Width/Height: A Simple Guide 💻📏
Have you ever wondered how to size an element on your app's screen relative to the screen size itself? 🤔 Maybe you want that CardView to take up a specific percentage of the screen width, making it look just right. Well, you're in luck! In this blog post, we'll explore an easy solution to this common problem without relying on LayoutBuilder. 🙌
The Challenge: Sizing an Element Relative to Screen Size
In the given scenario, we want to set the width of a CardView to be 65% of the screen width. Easy, right? But here's the catch: we can't directly do it inside the build
method because the screen dimensions aren't known until the layout is complete. So, how do we tackle this challenge? 🤷♀️
The Solution: Deferred Sizing After the Build Process
To size an element relative to the screen size, we need to wait until after the build process to access the screen dimensions. Here's a simple, non-LayoutBuilder approach you can follow:
Wrap your CardView inside a LayoutBuilder widget:
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
// Here, we have access to the screen dimensions
double screenWidth = constraints.maxWidth;
double desiredWidth = screenWidth * 0.65;
return CardView(
width: desiredWidth,
// Other properties...
);
},
);
Inside the
builder
function, extract the maximum width of the LayoutBuilder's constraints. This value represents the current screen width.Calculate the desired width for your CardView by multiplying the screen width by the desired percentage (in this case, 65%).
Finally, pass the calculated
desiredWidth
to the CardView'swidth
property.
Voila! 🎉 Your CardView is now sized to be 65% of the screen width, regardless of the device's dimensions. Pretty neat, huh? 😉
Where to Put the Logic?
Now for the million-dollar question: where should you put this logic? While there are different approaches, a preferred place to handle this is inside the build
method of a StatefulWidget. You can encapsulate the LayoutBuilder and CardView inside your own custom widget, making it reusable and easy to maintain.
Let's Get Coding! 🚀
Feeling inspired to try it out yourself? Fire up your favorite IDE and start implementing this technique for sizing elements relative to the screen size. Don't forget to share your creations in the comments below! Let's see those beautifully proportioned components! 😍
If you have any questions or encounter any issues, feel free to reach out. We're here to help you navigate your way through coding challenges! Happy coding! 💻❤️
Are you struggling with sizing elements? Share your experiences and let's solve these challenges together!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
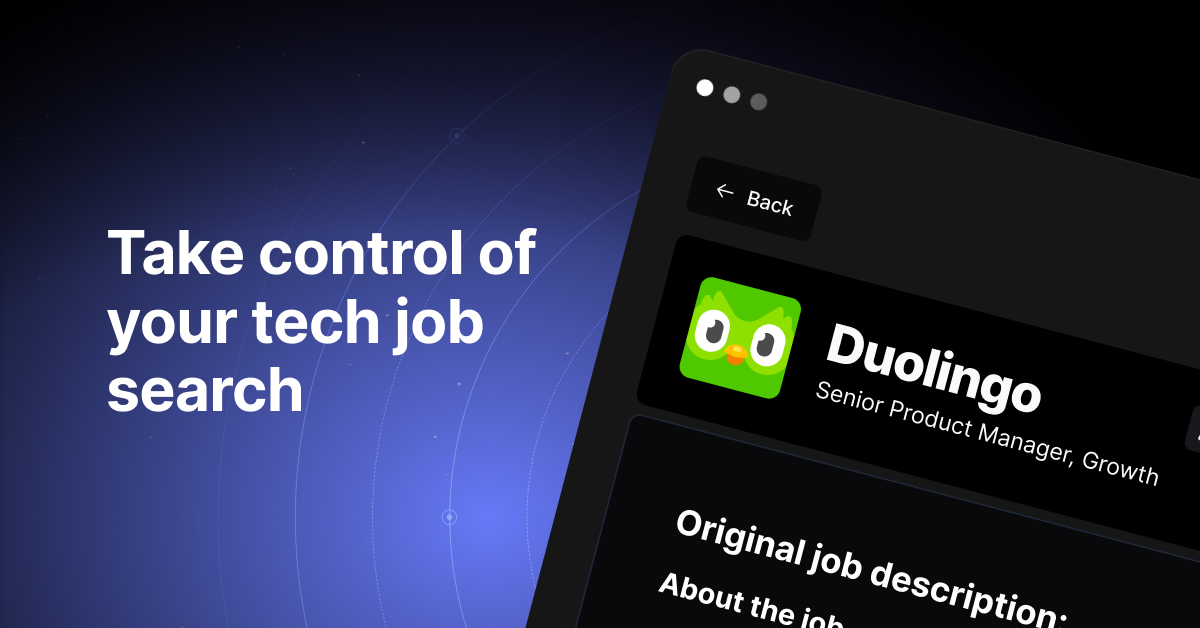