How To Override the “Back” button in Flutter?
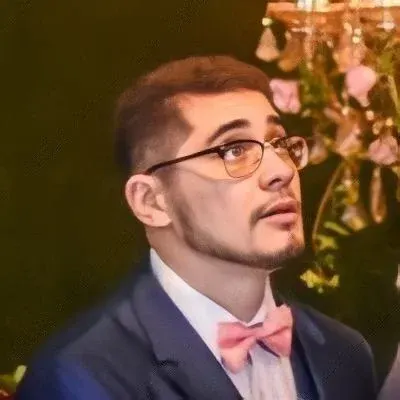
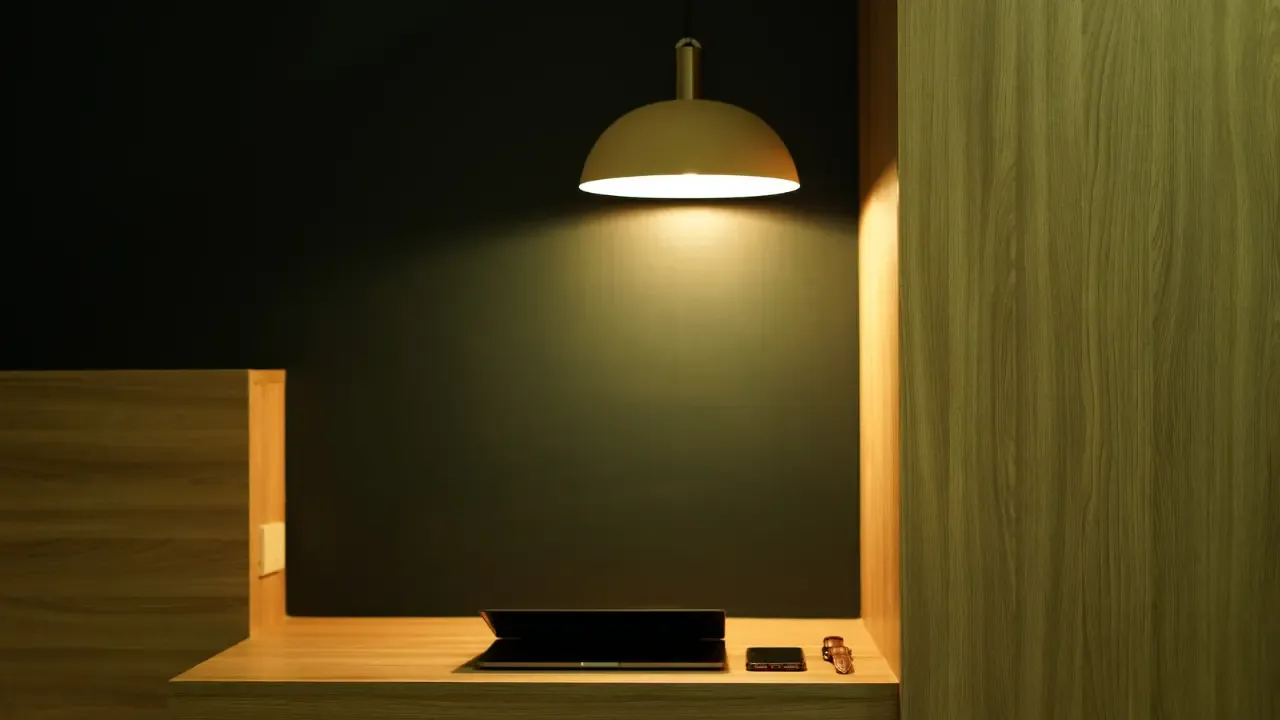
How to Override the "Back" Button in Flutter? 📱⏪
Are you struggling to handle the system back button in your Flutter app? 🤔 Don't worry, we've got you covered! In this guide, we'll walk you through the process of overriding the "Back" button and providing a confirmation dialog in your app when the user taps on it. Let's dive in! 💪
The Common Issue: Handling the System Back Button 😫⏪
Many app developers face the problem of wanting to customize the behavior of the system back button in their Flutter apps. By default, when the user taps the back button, the app simply navigates to the previous screen or exits the app if there are no screens left in the stack. However, in certain cases, you may want to show a confirmation dialog or perform a specific action before exiting the app, just like the user in this scenario. 🧐🔙
Easy Solution: Using the WillPopScope Widget 🛠️🔀
To override the system back button and provide a confirmation dialog, Flutter provides the WillPopScope
widget. This widget allows you to intercept the back button press and handle it according to your desired logic. Here's a step-by-step guide to implementing this solution:
Wrap your Home widget or the widget where you want to handle the back button press with the
WillPopScope
widget.
return WillPopScope(
onWillPop: () async {
// Your code for showing the confirmation dialog and handling the back button press goes here
return false; // Return true if you want to allow the back button press, false otherwise
},
child: YourWidget(), // Replace YourWidget with the actual name of your widget
);
Inside the
onWillPop
callback, you can show your confirmation dialog using theshowDialog
method. This is where you can ask the user if they really want to exit the app or perform any other desired action.
onWillPop: () async {
bool exit = await showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
title: Text("Exit App"),
content: Text("Do you want to exit the app?"),
actions: [
TextButton(
child: Text("No"),
onPressed: () {
Navigator.of(context).pop(false);
},
),
TextButton(
child: Text("Yes"),
onPressed: () {
Navigator.of(context).pop(true);
},
),
],
);
},
);
return exit ?? false;
},
Finally, return
true
orfalse
based on whether you want to allow the back button press or prevent it, respectively. Returningtrue
will allow the default back button behavior, while returningfalse
will prevent the back button press from exiting the app.
return exit ?? false;
Call-to-Action: Level up your Flutter app! ✨📲
Congratulations! 🎉 You've successfully learned how to handle and override the system back button in Flutter using the WillPopScope
widget. By customizing this behavior, you can provide a better user experience and ensure your app functions as desired. Don't stop here! Explore more Flutter techniques and enhance your app-building skills. 🚀💪
If you found this guide helpful, don't forget to share it with your fellow Flutter developers! Let us know in the comments below if you have any questions or other topics you'd like us to cover. Happy coding! 😄👨💻👩💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
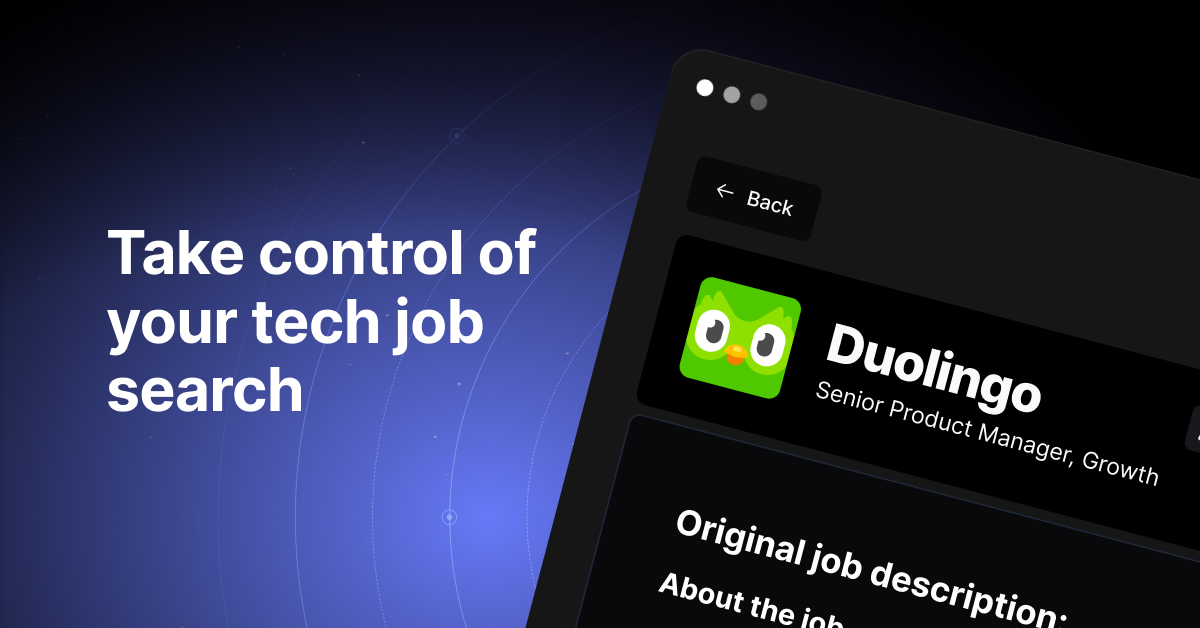