How to make flutter app responsive according to different screen size?
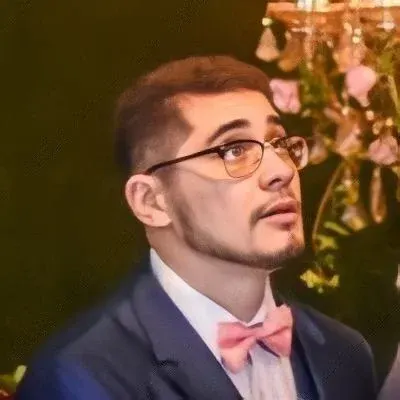

How to Make a Flutter App Responsive According to Different Screen Sizes
Are you struggling to make your Flutter app responsive across different screen sizes? Don't worry, we've got you covered! In this blog post, we'll address common issues and provide easy solutions to make your app look great on any device. 📱💻🖥️
The Problem
One of our readers recently asked us for help with making their Flutter app responsive to various screen sizes. They shared a code snippet from their build
method, which looked like this:
override
Widget build(BuildContext context) {
return new Container(
// Container properties
);
}
The code provided a simple container without any responsiveness logic.
The Solution
To make your Flutter app responsive, we need to utilize the available screen space efficiently. Here are a few steps you can take to achieve a responsive design:
Step 1: Use a LayoutBuilder
Wrap your Container
widget with a LayoutBuilder
widget. This will provide you with the current constraints of the parent widget, which you can use to build a responsive layout. Here's how you can implement it:
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return Container(
// Container properties
);
},
)
Step 2: Utilize Responsive Widget Properties
Use responsive widget properties like MediaQuery.of(context).size
and MediaQuery.of(context).orientation
to make your app responsive. These properties provide information about the current screen size and orientation, which can be used to adjust your layout dynamically.
For example, you can calculate the width and height ratios based on the screen size and adjust your widget sizes accordingly. Here's an updated version of the code snippet provided:
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
final double screenWidth = constraints.maxWidth;
final double screenHeight = constraints.maxHeight;
return Container(
width: screenWidth * 0.8,
height: screenHeight * 0.6,
// Container properties
);
},
)
Step 3: Use Responsive Design Patterns
Consider using responsive design patterns like Flex
, Wrap
, or GridView
to layout your widgets dynamically. These widgets adjust their child widgets based on available space, making them perfect for responsive layouts.
For example, you can use the Wrap
widget to wrap your child widgets and ensure they adjust to different screen sizes smoothly:
LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
return Wrap(
spacing: 10.0,
runSpacing: 10.0,
children: [
// Child widgets
],
);
},
)
Step 4: Test on Different Devices
Finally, it's crucial to test your app on various devices to ensure its responsiveness. Emulators and simulators can be used to simulate different screen sizes and orientations during the development process.
Wrapping Up
Making your Flutter app responsive doesn't have to be a daunting task. By using the provided steps and techniques, you can easily create a responsive layout that adapts to different screen sizes. Remember to test your app on various devices to ensure a smooth user experience.
If you found this guide helpful, feel free to share it with fellow Flutter developers who might be facing similar issues. 😊
Let us know in the comments if you have any questions or additional tips for building responsive Flutter apps. Happy coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
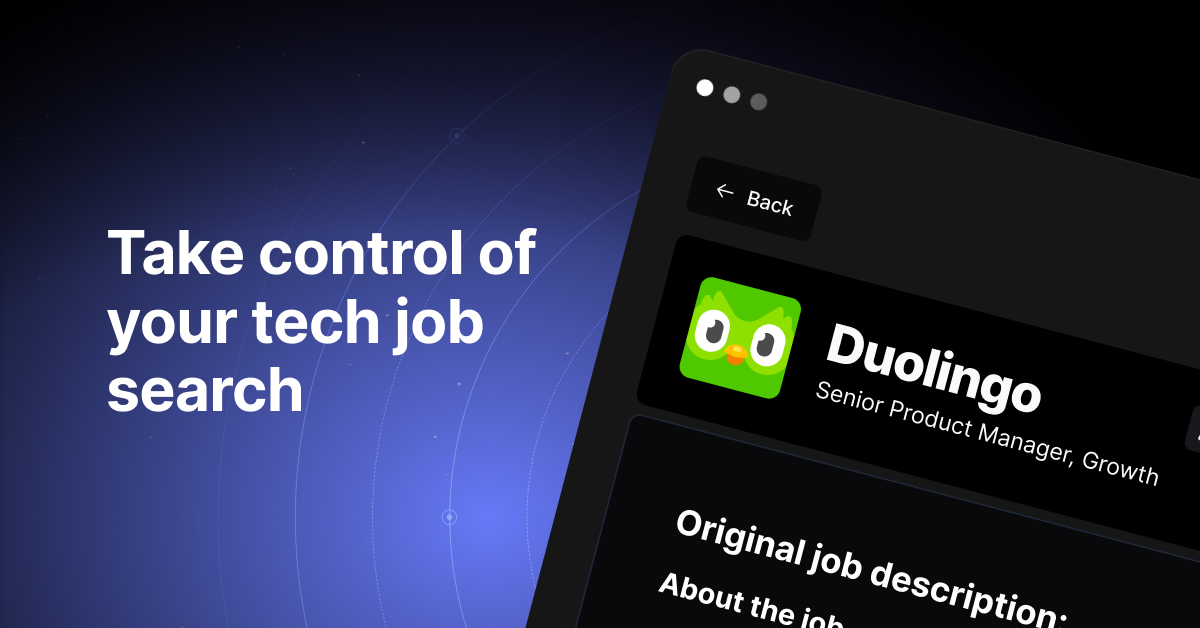