How to dynamically resize text in Flutter?
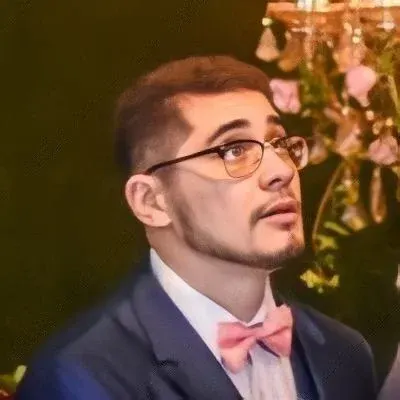
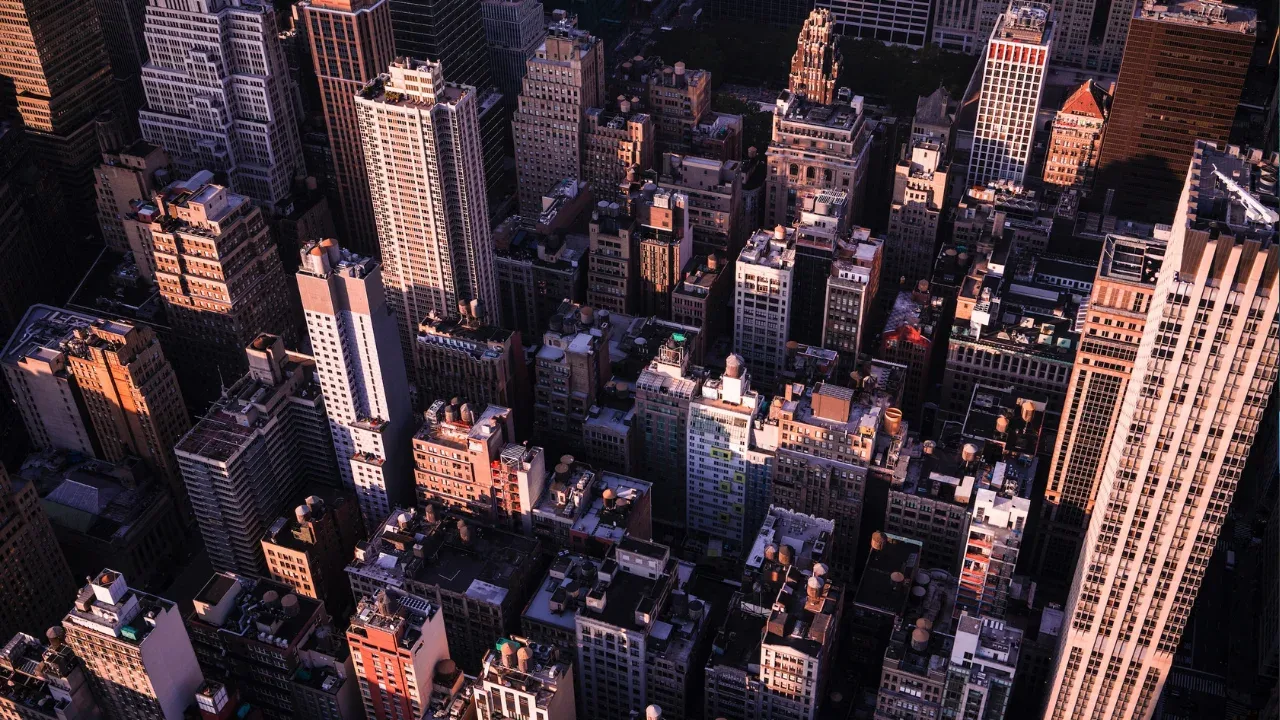
How to Dynamically Resize Text in Flutter 📏
Have you ever encountered the challenge of fitting a varying text length into a fixed space in your Flutter app? Maybe you retrieve text from an API, and it sometimes overflows the container, requiring you to manually adjust the font size. 😫
Fear not! In this guide, I will show you two dynamic approaches to resize text in Flutter based on the available space. By the end, you'll be able to effortlessly adjust the font size to fit any container while keeping your UI looking sleek and professional. 🎉
The ConstrainedBox + LayoutBuilder Strategy 📦🔧
One effective way to dynamically resize text is by combining the power of ConstrainedBox
and LayoutBuilder
. Let's dive into the implementation:
Container(
child: ConstrainedBox(
constraints: BoxConstraints(
minWidth: 300.0,
maxWidth: 300.0,
minHeight: 30.0,
maxHeight: 100.0,
),
child: LayoutBuilder(
builder: (BuildContext context, BoxConstraints constraints) {
if (/* height is larger than 100.0? height is over the constraints? */) {
return textWithSize24();
}
return textWithSize30();
},
),
),
),
With this approach, the ConstrainedBox
establishes the maximum dimensions for the text container, while the LayoutBuilder
helps you calculate the available space dynamically. The conditional statement checks if the current height exceeds the allowed constraints, enabling you to choose the appropriate font size for your text. 📐
Determining the Text Height and MaxLines 📏🧭
To calculate the text's height, we can use the TextPainter
class provided by the Flutter framework. Here's an example of how you can determine the text height based on the font size:
final text = 'Sample text';
final fontSize = 30.0;
final textPainter = TextPainter(
text: TextSpan(text: text, style: TextStyle(fontSize: fontSize)),
maxLines: 1,
textDirection: TextDirection.ltr,
);
textPainter.layout();
final textHeight = textPainter.height;
By setting the maxLines
property to 1
, we ensure that the text won't wrap to multiple lines. Now, you have the flexibility to compare textHeight
against your container's allowed maxHeight and adjust the font size accordingly. 📐🔍
The Manual Character Count Strategy 🔤✍️
If determining the text height dynamically feels complex for your specific use case, an alternative approach is to consider the number of characters in your text. While it may feel a bit more manual, it can work effectively depending on your requirements. Here's a simplified example:
final text = 'Sample text';
final maxCharacters = 50;
Widget determineTextSize(String text) {
return text.length > maxCharacters ? textWithSize24() : textWithSize30();
}
Here, we set a maximum character threshold (maxCharacters
), and if the text exceeds this limit, we choose a smaller font size. This approach works well if you have a good understanding of the expected text length and can adjust the value accordingly. 🔢✅
Engage and Share Your Experience! 💬🤝
Now that you have learned two powerful strategies for dynamically resizing text in Flutter, I encourage you to experiment with these methods and share your experience with the Flutter community. 🙌
Have you come across any other techniques or challenges related to text resizing in Flutter? Let's collaborate and help each other out! Feel free to leave a comment below and let's start the conversation. 💬👇
Happy coding, Flutter enthusiasts! Keep creating awesome apps with beautiful text that automatically adapts to any space. 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
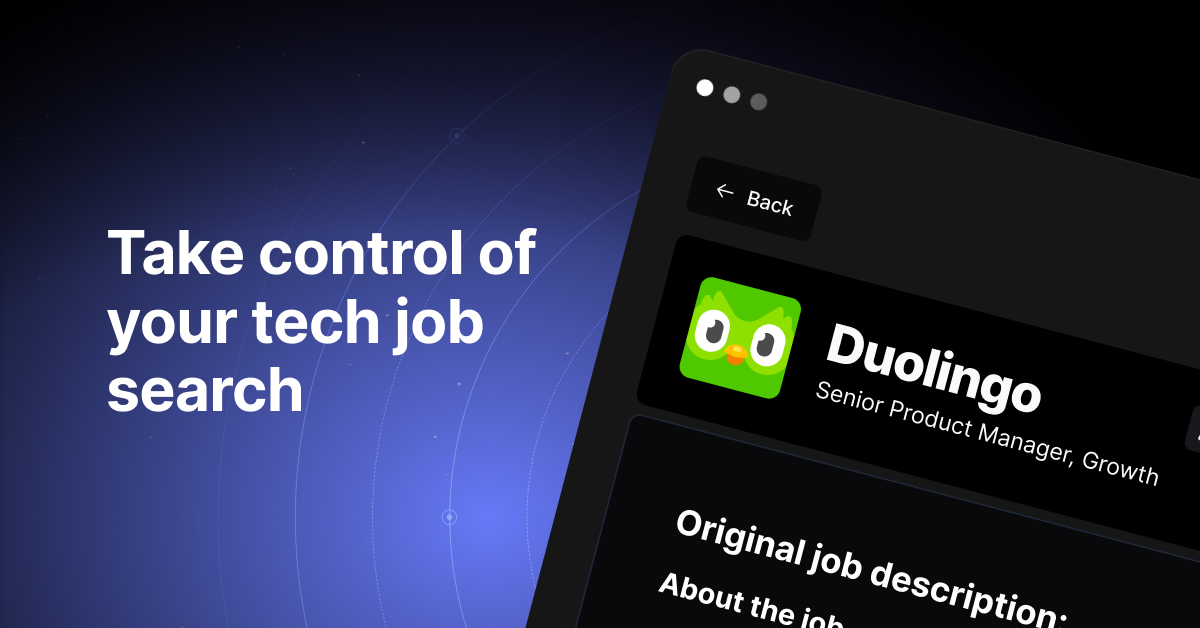