How to deal with unwanted widget build?
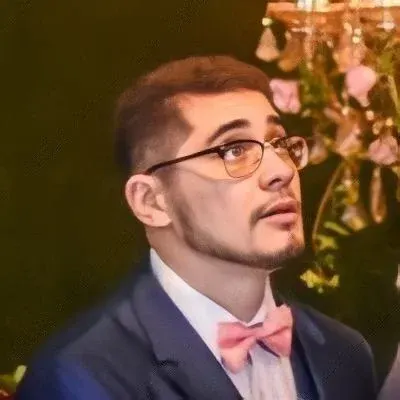
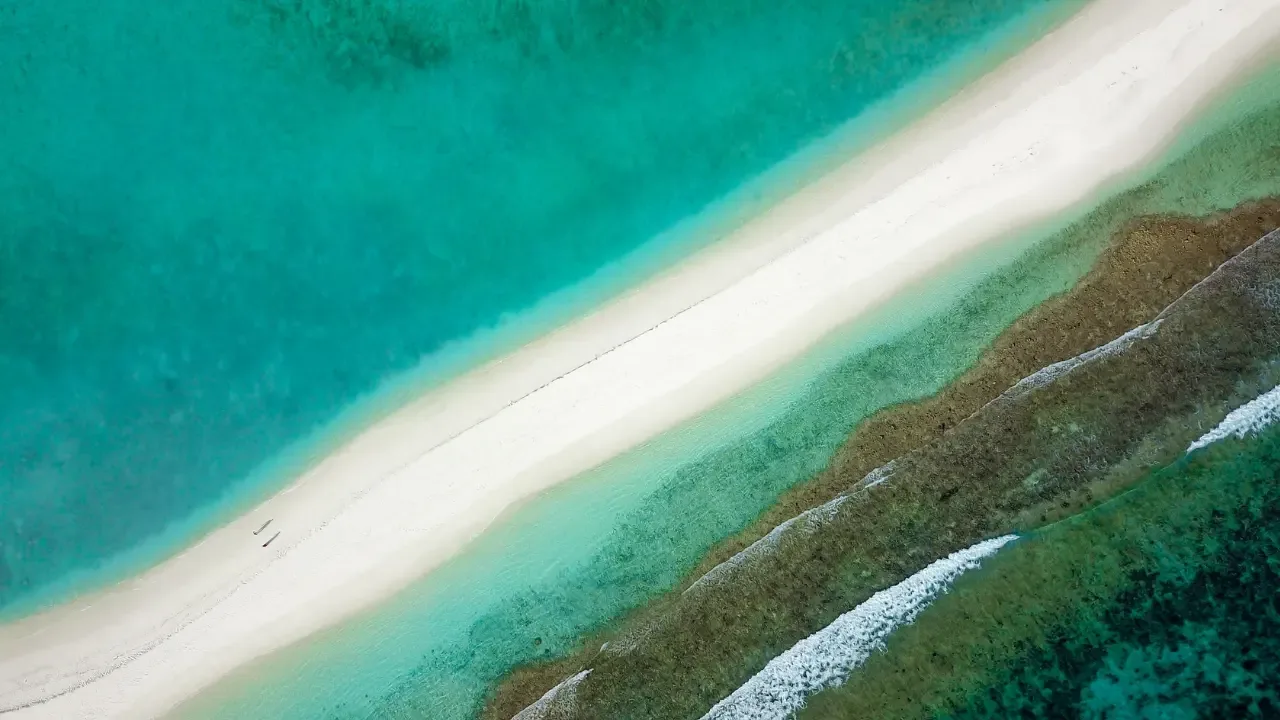
How to Deal with Unwanted Widget Build 👷♂️
Do you sometimes find yourself in a situation where the build
method of your widgets is called again for reasons beyond your control? 🤔 It can be frustrating, especially when it leads to undesired effects in your app. But worry not, we've got you covered with some easy solutions to deal with unwanted widget builds! 💪
Understanding the Cause 🕵️♂️
Firstly, it's important to understand why this is happening. Typically, the build
method is called when a widget's state changes or when a widget's parent is updated. In the context of your question, it seems that the undesired build is triggered by a parent update.
Example Scenario: Unwanted Build with FutureBuilder
🔄
Let's dive into a specific example to better understand the issue. Consider the following code snippet that uses FutureBuilder
:
@override
Widget build(BuildContext context) {
return FutureBuilder(
future: httpCall(),
builder: (context, snapshot) {
// create some layout here
},
);
}
In this example, if the build
method were to be called again, it would trigger another HTTP request. This is certainly not desired as it can lead to multiple redundant requests and potential performance issues. 😓
Solution: Preventing the Unwanted Build 🚧
Thankfully, there are a few strategies you can employ to prevent the unwanted build in situations like this. Let's explore them:
1. Using Builder
Widget ✅
One way to avoid the unwanted build is by wrapping the widget that causes the undesired rebuild with a Builder
widget. The Builder
widget creates a new BuildContext
and ensures that only the wrapped portion is rebuilt when its state changes.
@override
Widget build(BuildContext context) {
return Builder(
builder: (context) {
return FutureBuilder(
future: httpCall(),
builder: (context, snapshot) {
// create some layout here
},
);
},
);
}
By doing this, the parent widget's state won't affect the Builder
widget and the undesired rebuild will be prevented.
2. Leveraging AutomaticKeepAliveClientMixin
♻️
If you need to preserve the state of your widget even when it gets rebuilt, you can use the AutomaticKeepAliveClientMixin
. This mixin allows your widget to keep its state across rebuilds, effectively preventing the unwanted build.
To use the AutomaticKeepAliveClientMixin
, you'll need to make your widget a subclass of StatefulWidget
and include the mixin in its state class:
class MyWidget extends StatefulWidget {
@override
_MyWidgetState createState() => _MyWidgetState();
}
class _MyWidgetState extends State<MyWidget> with AutomaticKeepAliveClientMixin {
// your code here
@override
Widget build(BuildContext context) {
super.build(context); // call super.build to activate the mixin
return FutureBuilder(
future: httpCall(),
builder: (context, snapshot) {
// create some layout here
},
);
}
@override
bool get wantKeepAlive => true;
}
With this approach, your widget will retain its state even when the parent updates, effectively preventing any undesired builds.
Conclusion and Action Time ✌️
Dealing with unwanted widget builds can be a real headache, but with the right approach, you can overcome this challenge! Try out the solutions mentioned above and prevent those undesired builds in your Flutter apps.
Remember, understanding the cause of the issue, leveraging tools like Builder
widget and AutomaticKeepAliveClientMixin
, are key steps towards resolving this problem.
Now it's your turn! Have you encountered unwanted builds in your Flutter journey? How did you deal with them? Share your experiences and tips in the comments below! 👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
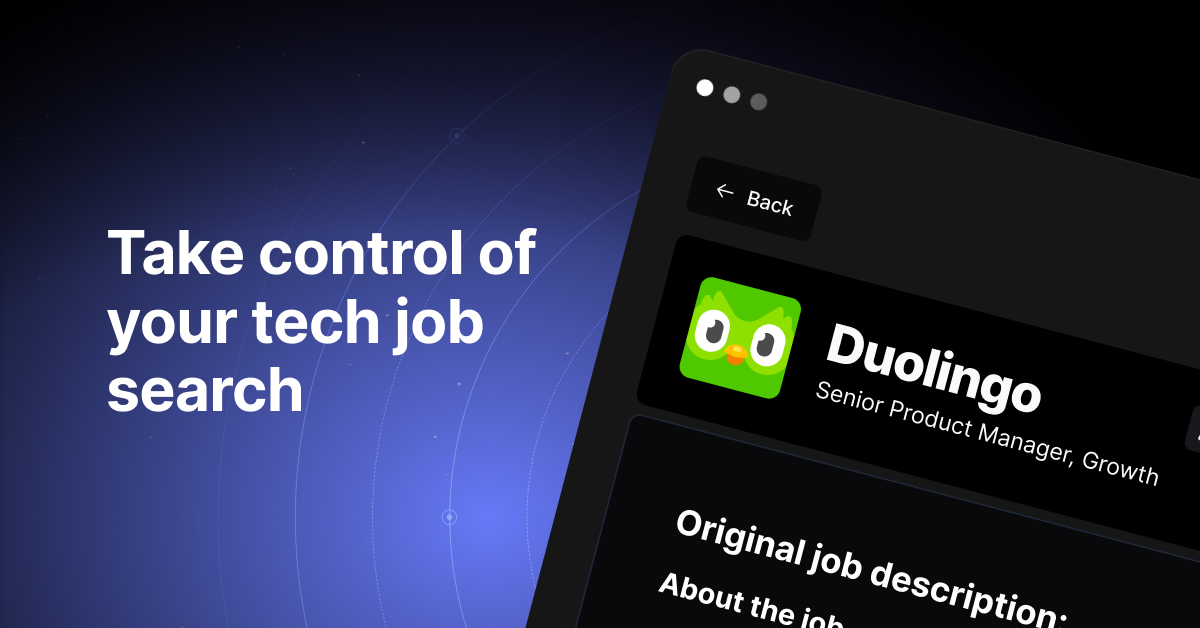