How to create number input field in Flutter?
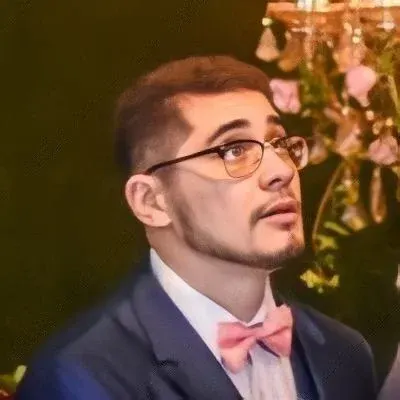
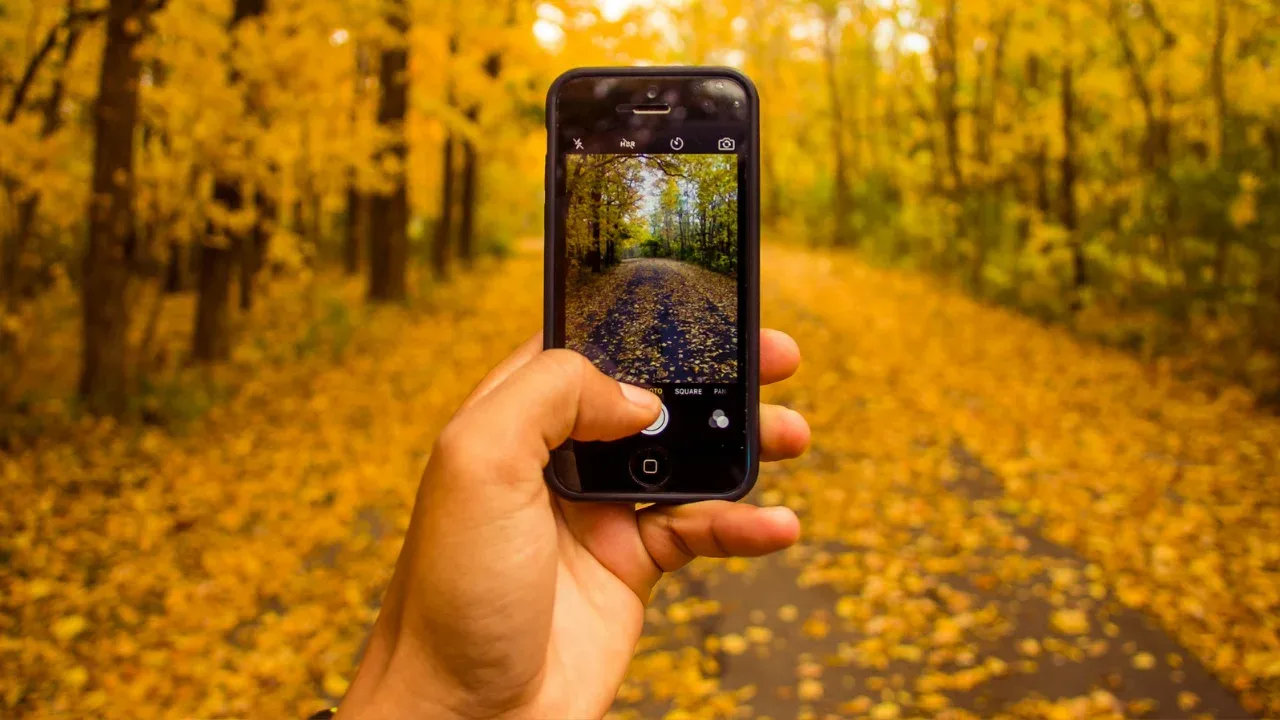
How to Create a Number Input Field in Flutter? 📱💯
So you want to create a number input field in Flutter, but you're struggling to figure out how. Don't worry, you're not alone! Many Flutter developers have had the same issue. In this blog post, we will not only address this common problem but also provide you with easy solutions and a compelling call-to-action. Let's get started!
Understanding the Problem 🤔
The challenge here is to create an input field in Flutter that opens up a numeric keyboard and only allows numeric input. While this feature may not be explicitly documented, it is indeed supported by Flutter material widgets.
Solution: Using the TextInputType Enum 🎉
Flutter provides the TextInputType
enum to specify the type of input for a text field. Luckily, it includes an option for numeric input.
To create a number input field, follow these step-by-step instructions:
Import the material library:
import 'package:flutter/material.dart';
Declare a
TextEditingController
to handle the input:final TextEditingController _controller = TextEditingController();
Use a
TextField
widget to create the input field:
TextField(
controller: _controller,
keyboardType: TextInputType.number,
)
By setting the keyboardType
property to TextInputType.number
, we ensure that the keyboard that appears when the field is tapped only includes numeric digits.
Example Code 🖥️💡
To help you visualize the implementation, here's an example of a complete Flutter widget that includes the number input field:
import 'package:flutter/material.dart';
class NumberInputScreen extends StatelessWidget {
final TextEditingController _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Number Input Field'),
),
body: Center(
child: Padding(
padding: EdgeInsets.all(16.0),
child: TextField(
controller: _controller,
keyboardType: TextInputType.number,
),
),
),
);
}
}
Feel free to customize the NumberInputScreen
widget as needed to fit into your app.
Call-to-Action: Share Your Thoughts! 📢💬
Now that you know how to create a number input field in Flutter, it's time to put this knowledge into action! Try implementing it in your own app and see how it works for you.
Have any questions, concerns or additional tips to share? We'd love to hear your thoughts in the comments below! Let's create an engaging community of Flutter developers who help each other grow. Don't hesitate to join the conversation and let us know how your number input field implementation went.
Happy Fluttering! 🚀✨
Conclusion 🎊
Creating a number input field in Flutter may seem like a daunting task at first, but with the right guidance, it becomes easy. By leveraging the TextInputType.number
option available through the keyboardType
property, you can provide users with a numeric keyboard and ensure that only numeric input is accepted.
Remember, Flutter has a vibrant and supportive community, so if you encounter any issues or have any questions, don't hesitate to reach out for help. Together, we can make the development journey smoother and more enjoyable for all Flutter developers!
Happy coding! 💻🎉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
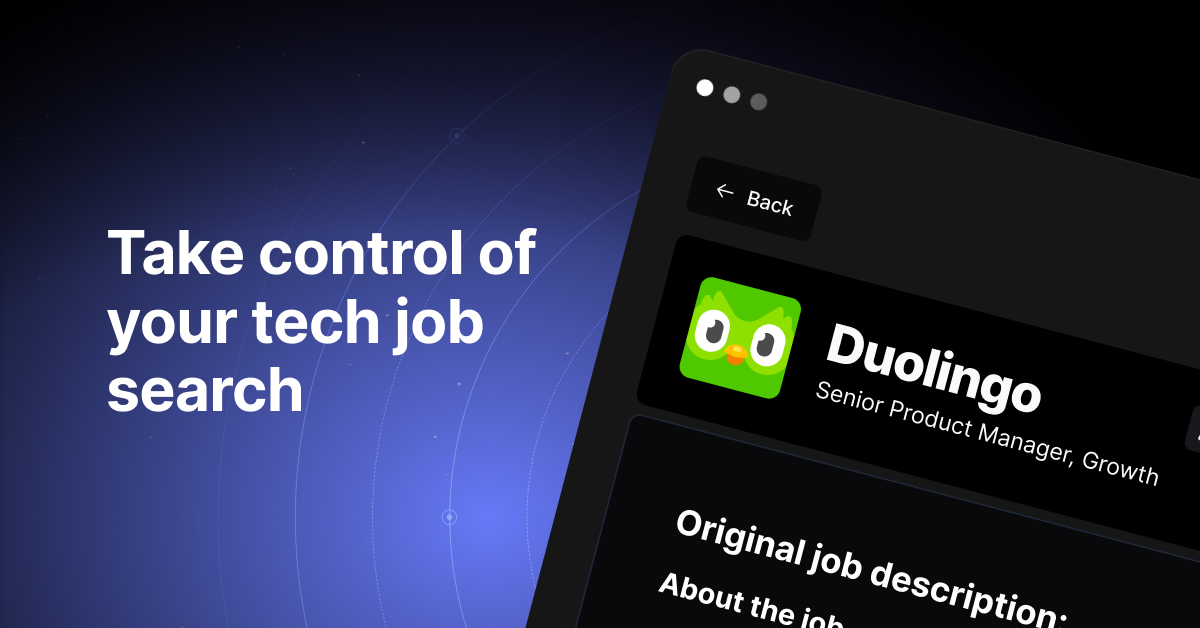