How to add a ListView to a Column in Flutter?
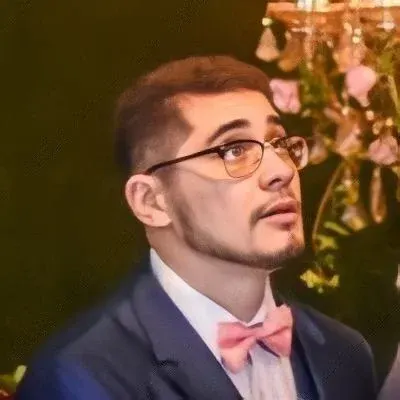

How to Add a ListView to a Column in Flutter 😎
If you're building a Flutter app and want to add a horizontal ListView within a Column, you might have encountered a problem where your elements disappear. But fear not! In this easy guide, we'll show you how to correctly add a ListView to a Column in Flutter so your elements stay visible. Let's get started! 🚀
Understanding the Problem 💡
Here's the code snippet where the issue lies:
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Login / Signup"),
),
body: Container(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
// Existing TextFields and Buttons...
ListView(
scrollDirection: Axis.horizontal,
children: <Widget>[
RaisedButton(
onPressed: null,
child: Text("Facebook"),
),
RaisedButton(
onPressed: null,
child: Text("Google"),
)
],
),
],
),
),
margin: EdgeInsets.all(15.00),
),
),
);
When you run this code, the ListView elements disappear. However, if you remove the ListView, everything works fine again. The problem lies in how the ListView is added to the Column.
The Solution 🛠️
To ensure that the ListView remains visible within the Column, you need to wrap the ListView and the existing Column elements with a SingleChildScrollView. Here's the modified code:
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text("Login / Signup"),
),
body: Container(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
// Existing TextFields and Buttons...
SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Row(
children: <Widget>[
RaisedButton(
onPressed: null,
child: Text("Facebook"),
),
SizedBox(width: 5.00),
RaisedButton(
onPressed: null,
child: Text("Google"),
)
],
),
),
],
),
),
margin: EdgeInsets.all(15.00),
),
),
);
By wrapping the ListView and the existing Column elements inside a SingleChildScrollView, you allow the ListView to scroll horizontally without affecting the visibility of other elements. The Row widget is used inside the SingleChildScrollView to display the ListView elements horizontally.
That's it! 🎉 Now your ListView will peacefully coexist with the other elements in your Column.
Conclusion and Call-to-Action 🚀
Adding a ListView to a Column in Flutter requires an understanding of how widgets interact with each other. With the solution provided in this guide, you can now confidently add horizontal ListViews to your Columns, ensuring that your elements stay visible.
If you found this guide helpful, feel free to share it with your fellow Flutter developers or leave a comment below. Happy coding! 😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
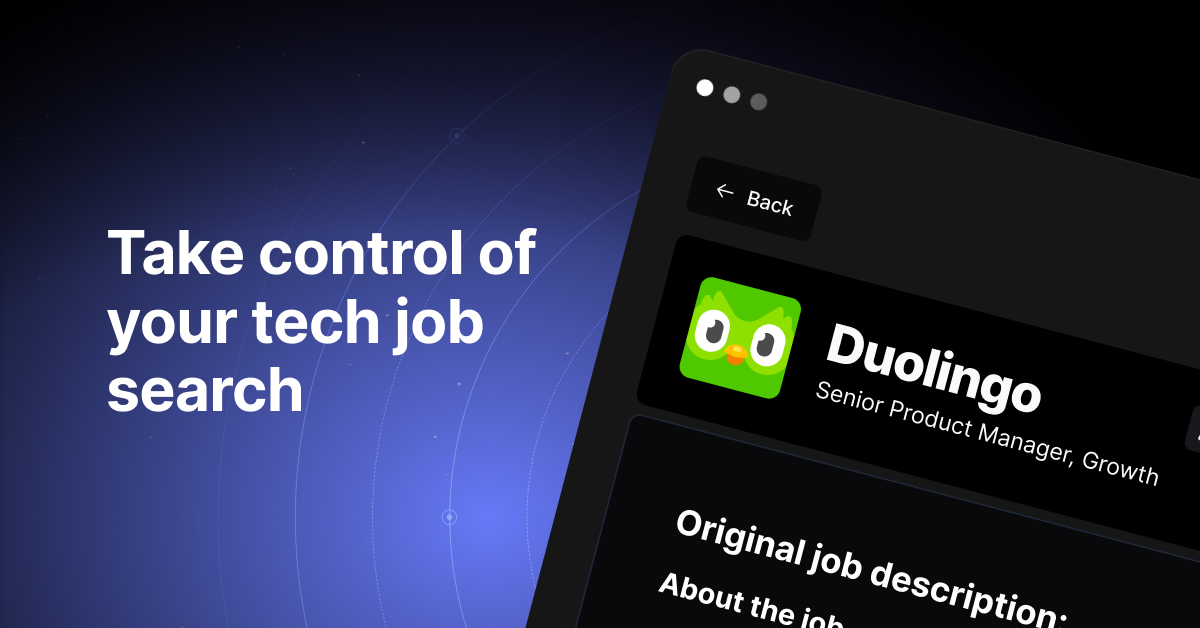