How do I supply an initial value to a text field?
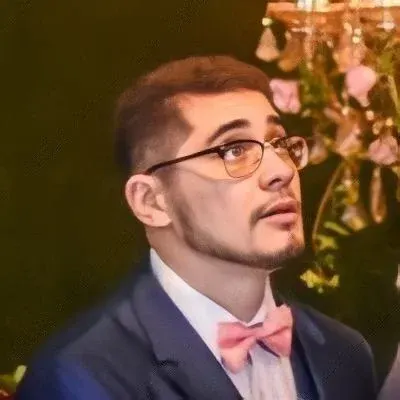
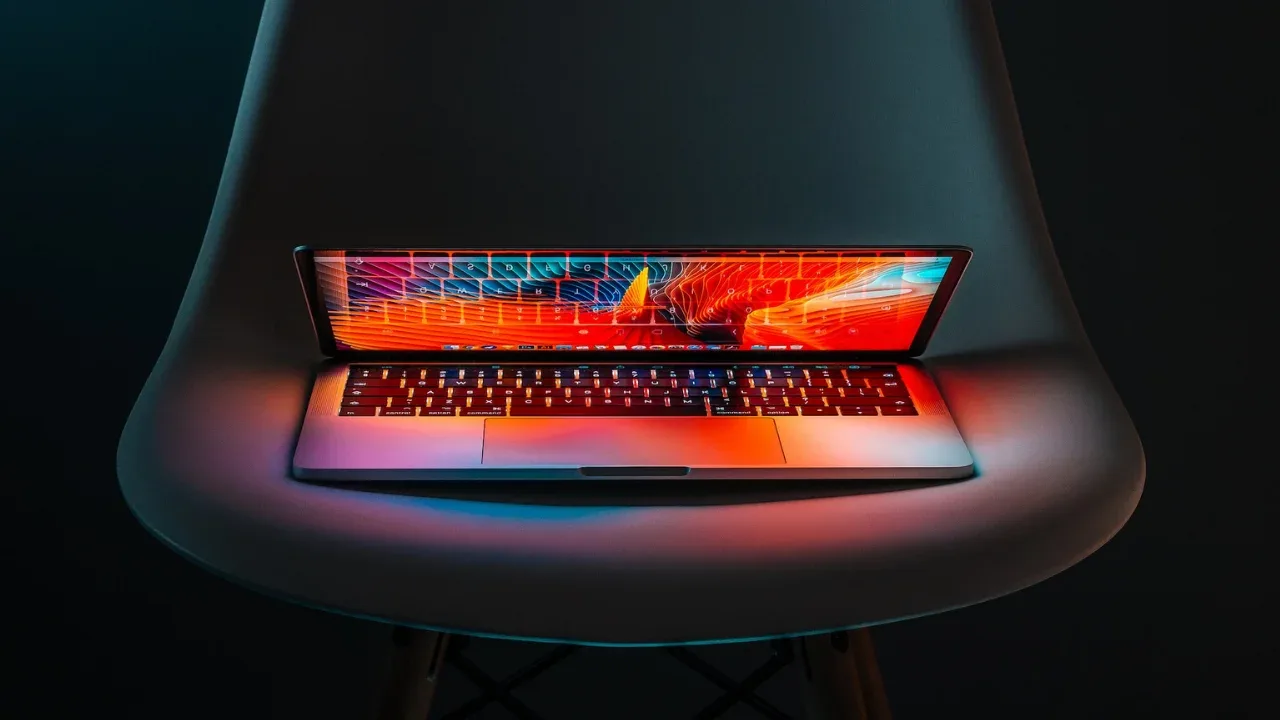
š Tech Blog Post: How to Supply an Initial Value to a Text Field in Flutter
š¤ Are you struggling with setting an initial value to a text field in Flutter? Don't worry, we've got you covered! In this blog post, we'll explore common issues and provide easy solutions to help you get that initial value right. Let's dive in! š»
Understanding the Problem
The goal is clear - you want to populate your text field with a pre-defined value and then have the ability to clear it by redrawing it with an empty value. However, figuring out the best approach can sometimes feel like wandering through a never-ending maze. But fear not, there are a few methods that can help you achieve this seamlessly.
Solution 1: Using the TextEditingController
One of the most common and straightforward ways to supply an initial value to a text field is by utilizing the TextEditingController
. Here's how you can do it:
TextEditingController _controller = TextEditingController(text: 'Your initial value');
Widget buildTextField() {
return TextField(
controller: _controller,
// Other properties...
);
}
void clearTextField() {
_controller.clear();
}
In this approach, we create a TextEditingController
and assign the pre-defined initial value to its text
property. Then, we pass this controller to the controller
parameter of the TextField
widget. This way, the text field gets populated with the initial value automatically.
To clear the text field, you can call the clear()
method on the controller.
Solution 2: Making Use of initialValue
If you prefer a more concise approach without using a controller, Flutter has an alternative. You can utilize the initialValue
property directly in the TextField
widget:
Widget buildTextField() {
return TextField(
initialValue: 'Your initial value',
// Other properties...
);
}
By setting the initialValue
property to your desired initial value, you achieve the same goal as with the first approach. However, keep in mind that this method does not provide a built-in way to clear the text field without manually changing the value yourself.
Conclusion
You don't have to get lost in the confusion of providing an initial value to a text field in Flutter. Whether you prefer using the TextEditingController
or the initialValue
property, our solutions will help you set that initial value effortlessly.
Now it's your turn! Give these methods a try and let us know which one worked best for you. If you have any other tips or tricks, feel free to share them in the comments below. Happy coding! ššØāš»
Remember, coding is all about experimentation, so don't be afraid to test out different approaches and find the one that suits your needs the best. Happy Fluttering! š
š„ Don't forget to subscribe to our newsletter to receive more helpful Flutter tips and tricks directly in your inbox! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
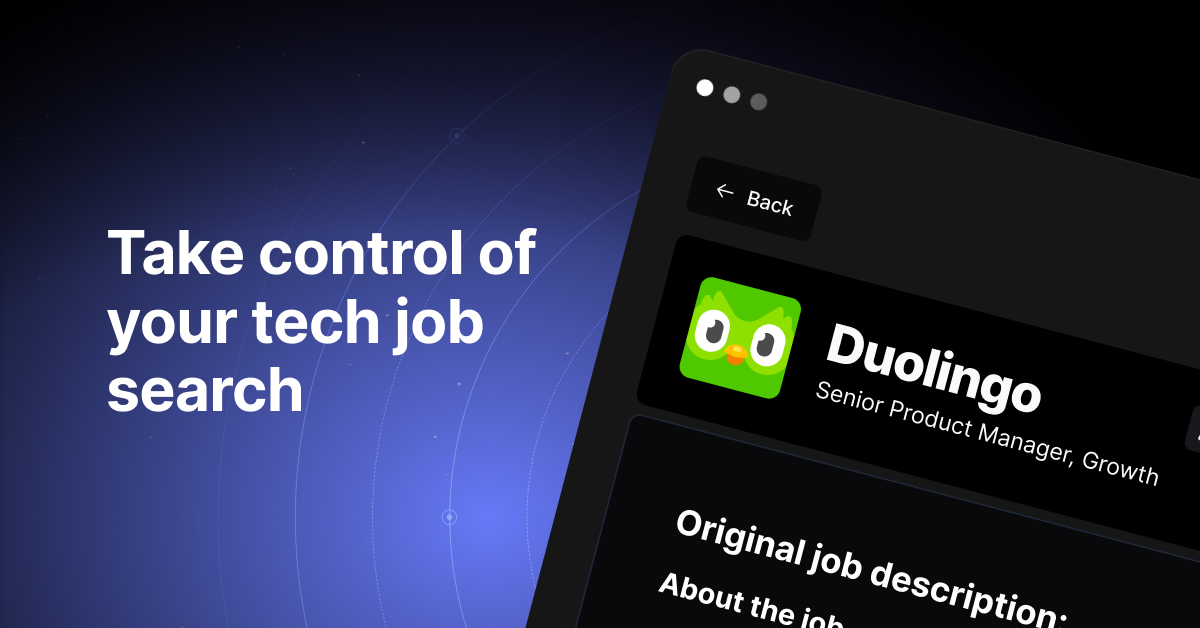