Flutter how to programmatically exit the app
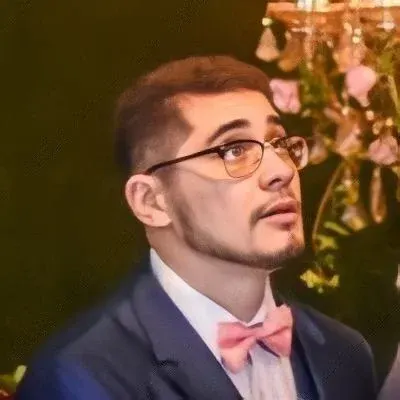
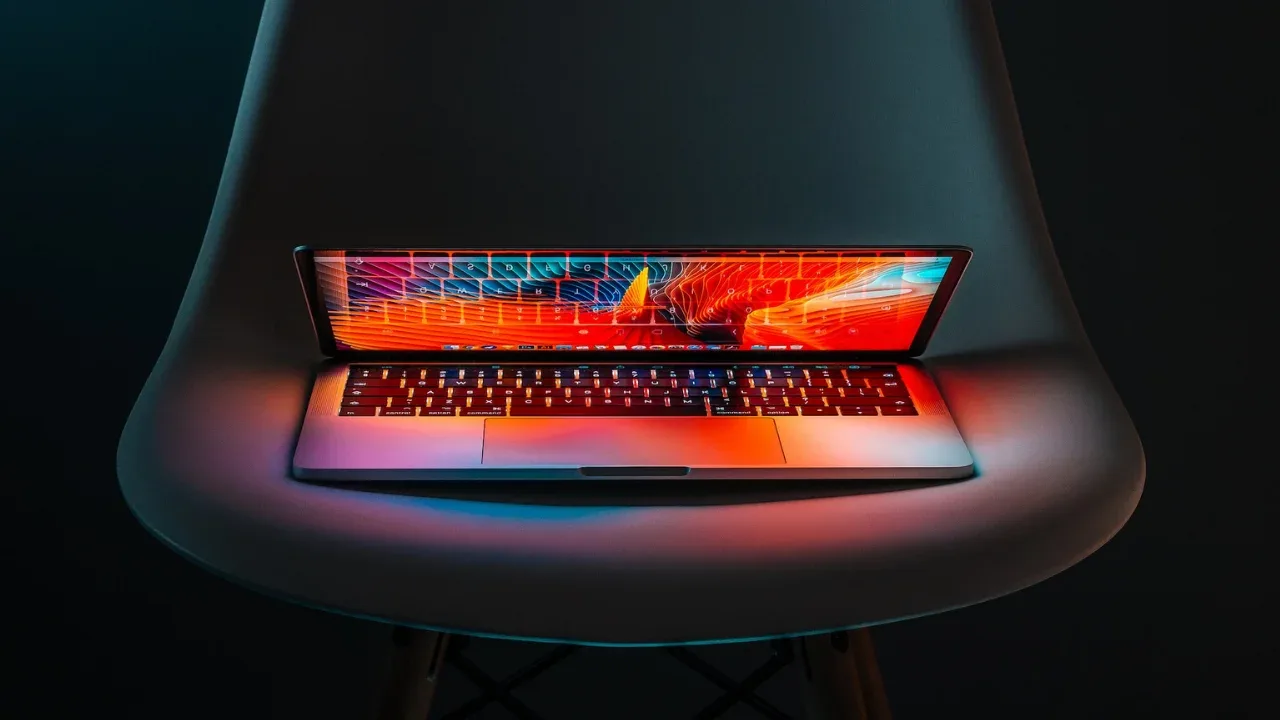
🚀 Exiting Flutter App Programmatically: Easy Solutions and Tips
Are you in a situation where you need to programmatically exit your Flutter app, but you find yourself facing a black screen? 😩 Don't worry, we've got your back! In this guide, we'll address the common issues you might encounter and provide easy solutions to help you overcome this challenge. Let's get started! 💪
The Challenge
The standard approach to exit a Flutter app is typically to use the Navigator
and call pop
to remove the current screen. However, if you try to pop the only screen, you may end up with an annoying black screen. This issue can be frustrating, but fortunately, there are alternative methods to solve this problem!
🌟 Solution 1: SystemChannels Approach
One solution is to use the SystemChannels
class provided by Flutter. This approach allows you to send a platform message directly to the Android or iOS system, gracefully closing the app. Let's see how it works:
import 'package:flutter/services.dart';
void exitApp() {
if (Platform.isAndroid) {
SystemChannels.platform.invokeMethod('SystemNavigator.pop');
} else if (Platform.isIOS) {
exit(0);
}
}
This code snippet shows the basic implementation of exiting the app programmatically using SystemChannels
and checking the platform to differentiate between Android and iOS devices. By invoking the SystemNavigator.pop
method or calling exit(0)
for Android and iOS respectively, you can safely exit your app.
🌟 Solution 2: Call exit(0)
Directly
Another workaround for this issue is to directly call the exit(0)
function. This method forcefully terminates your app, regardless of whether it's the recommended approach or not. While it may not be the most elegant solution, it works effectively for certain scenarios.
Here's an example of using exit(0)
, which you can place in your desired section of the code:
import 'dart:io';
void exitApp() {
exit(0);
}
While this solution may not be ideal for all situations due to potential side effects, it's worth considering when you need a quick and simple exit mechanism.
🌟 Solution 3: Use Flutter's Platform
Class
If you prefer a Flutter-specific solution instead of relying on system channels, you can utilize the Platform
class provided by the Flutter framework. Here's an example of how you can exit the app programmatically using Platform
:
import 'dart:io';
void exitApp() {
if (Platform.isAndroid || Platform.isIOS) {
exit(0);
}
}
By checking the current platform using Platform.isAndroid
or Platform.isIOS
, you can safely call exit(0)
within the respective platform conditional statement.
📣 Your Feedback Matters!
We hope these solutions help you overcome the black screen issue when trying to programmatically exit your Flutter app. Give them a try and let us know which one works best for you! If you have any other questions or face challenges in app development, feel free to leave a comment. We love hearing from you and are committed to helping you succeed! 🙌
Keep coding, keep Fluttering! 🚀💙
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
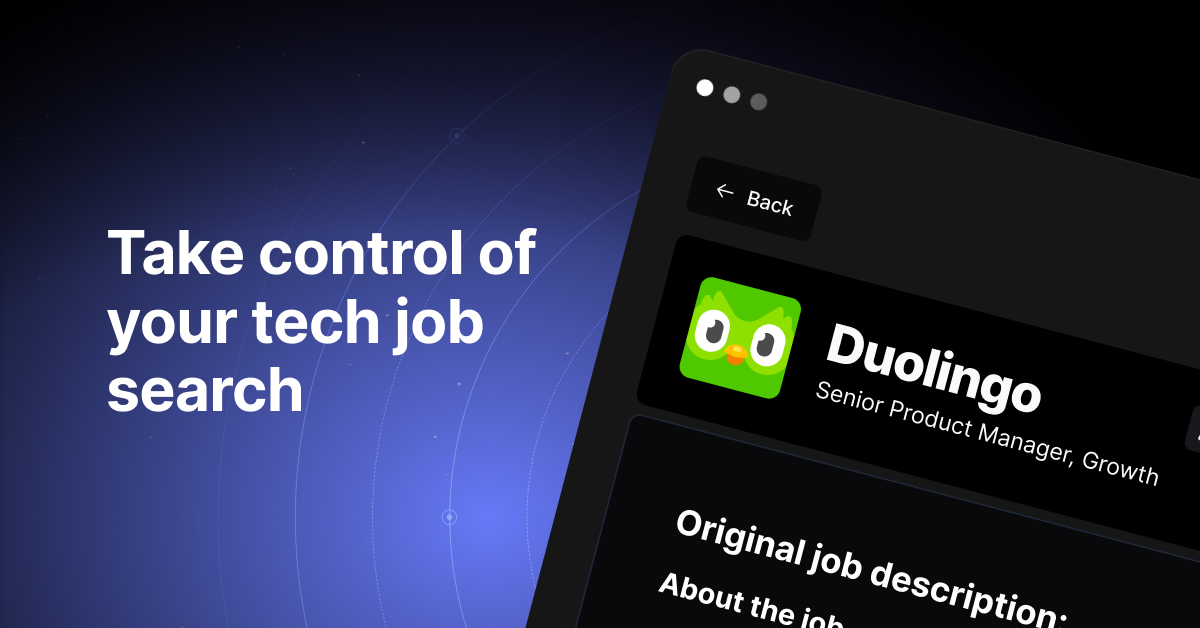