Flutter: How to correctly use an Inherited Widget?
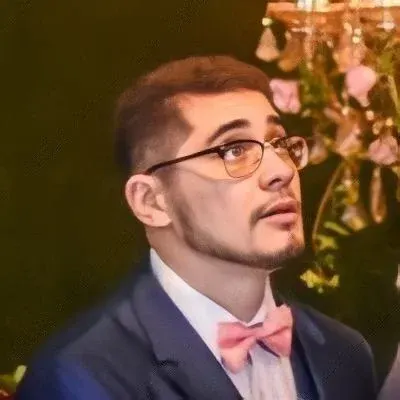
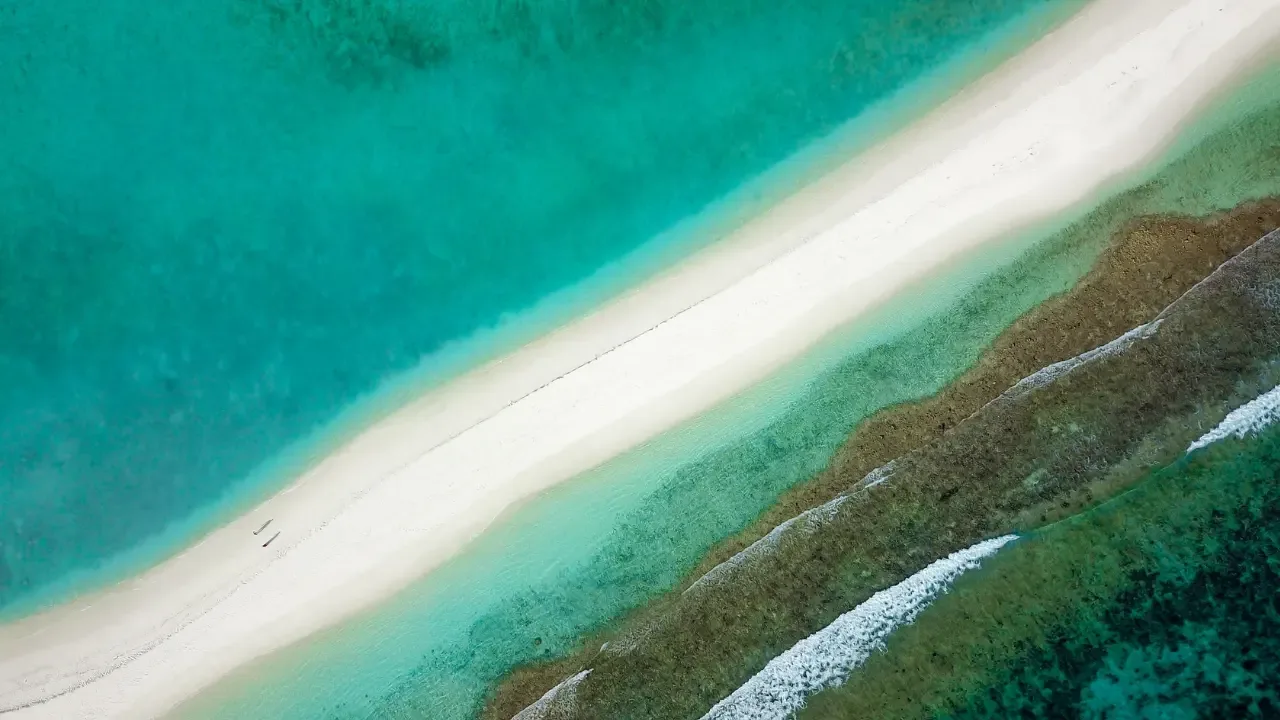
Flutter: How to correctly use an Inherited Widget? 😃
If you're new to Flutter or struggling to understand how to correctly use an InheritedWidget, you're not alone. The concept of InheritedWidget can be a bit confusing at first, especially when it comes to updating the widget and triggering rebuilds. But fear not, we're here to demystify it for you! 🚀
Understanding the purpose of InheritedWidget 🤔
InheritedWidget is an essential part of Flutter's state management system. Its main purpose is to allow you to propagate data down the widget tree without resorting to global variables or singletons. By using InheritedWidget, you can provide data to multiple widgets in your app without explicitly passing it through the constructors of each widget.
The immutability dilemma 🌚
One of the common questions that arises when using InheritedWidget is: "How can I update it if it's immutable?" Good question! 🤔 InheritedWidget is indeed immutable, but fear not, there's a solution. The key lies in understanding that InheritedWidget is the container for the data, but the state that contains the data can be mutable.
Updating the InheritedWidget state 🔄
To update the state of an InheritedWidget, you need to wrap it in a StatefulWidget. This way, you can mutate the state of the StatefulWidget and pass this updated data down to the InheritedWidget, which, in turn, hands the data down to all of its children widgets.
But here's the catch: When you update the state of the StatefulWidget, it will trigger a rebuild of the entire subtree underneath it, not just the widgets that reference the InheritedWidget. 🌳 This means that all the widgets underneath the StatefulWidget will rebuild, even if they don't reference the InheritedWidget.
updateShouldNotify to the rescue 🛡️
To avoid unnecessary rebuilds, Flutter provides the updateShouldNotify
method. This method allows you to specify whether a widget and its subtree should be rebuilt when the state of the InheritedWidget changes. By default, updateShouldNotify
returns true, which means that any change in the InheritedWidget's state will trigger a rebuild of the associated widgets. However, you can provide custom logic in updateShouldNotify
to skip the rebuild if the data hasn't actually changed.
An example to bring it all together 🌟
Let's take a look at an example to solidify our understanding. Imagine you have a CounterInheritedWidget that holds a count value, and you want to increment this count in a button press event:
class CounterInheritedWidget extends InheritedWidget {
CounterInheritedWidget({
Widget? child,
required this.count,
}) : super(child: child);
final int count;
static CounterInheritedWidget of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<CounterInheritedWidget>()!;
}
@override
bool updateShouldNotify(CounterInheritedWidget oldWidget) {
return oldWidget.count != count;
}
}
class CounterWidget extends StatefulWidget {
@override
_CounterWidgetState createState() => _CounterWidgetState();
}
class _CounterWidgetState extends State<CounterWidget> {
int count = 0;
void incrementCount() {
setState(() {
count++;
});
}
@override
Widget build(BuildContext context) {
return CounterInheritedWidget(
count: count,
child: ElevatedButton(
onPressed: incrementCount,
child: Text('Increment Count'),
),
);
}
}
In this example, we define a CounterInheritedWidget that holds the count value. The CounterWidget, which is a StatefulWidget, increments the count whenever the button is pressed. The CounterWidget also wraps itself in the CounterInheritedWidget, passing the current count value. This allows any child widget that depends on CounterInheritedWidget to access and use the count value without explicitly passing it.
Conclusion and your next steps 🏁
InheritedWidget can be a powerful tool in managing and sharing data within your Flutter app. While it may have its nuances, understanding how to correctly use InheritedWidget will greatly improve your app's state management. So go ahead, give it a try, and don't hesitate to ask if you have any questions along the way! 💪
Have you used InheritedWidget in your Flutter projects? What challenges did you encounter, and how did you overcome them? Share your experiences and thoughts in the comments below. Let's learn and grow together! 👇
Happy coding! 😉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
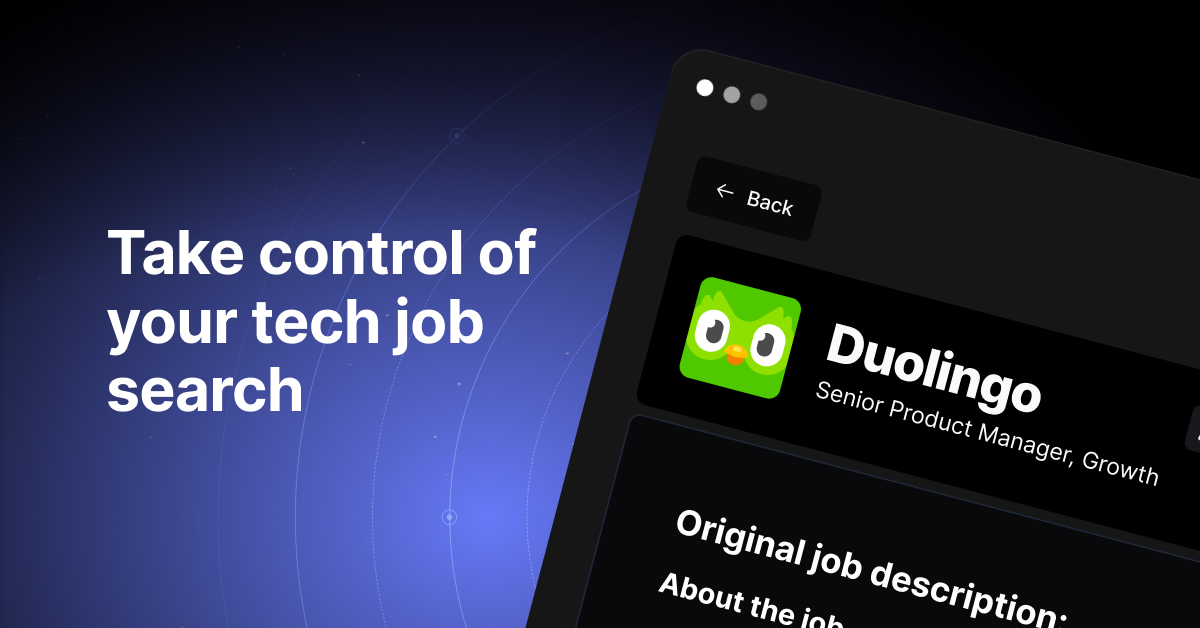