type "List<dynamic>" is not a subtype of type "List<Widget>"
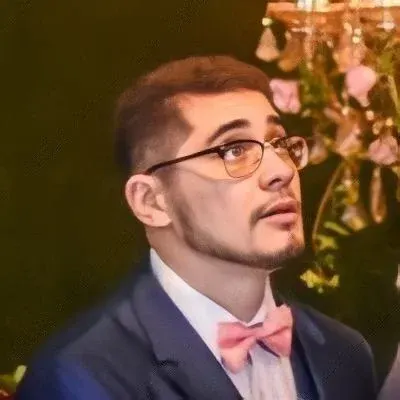
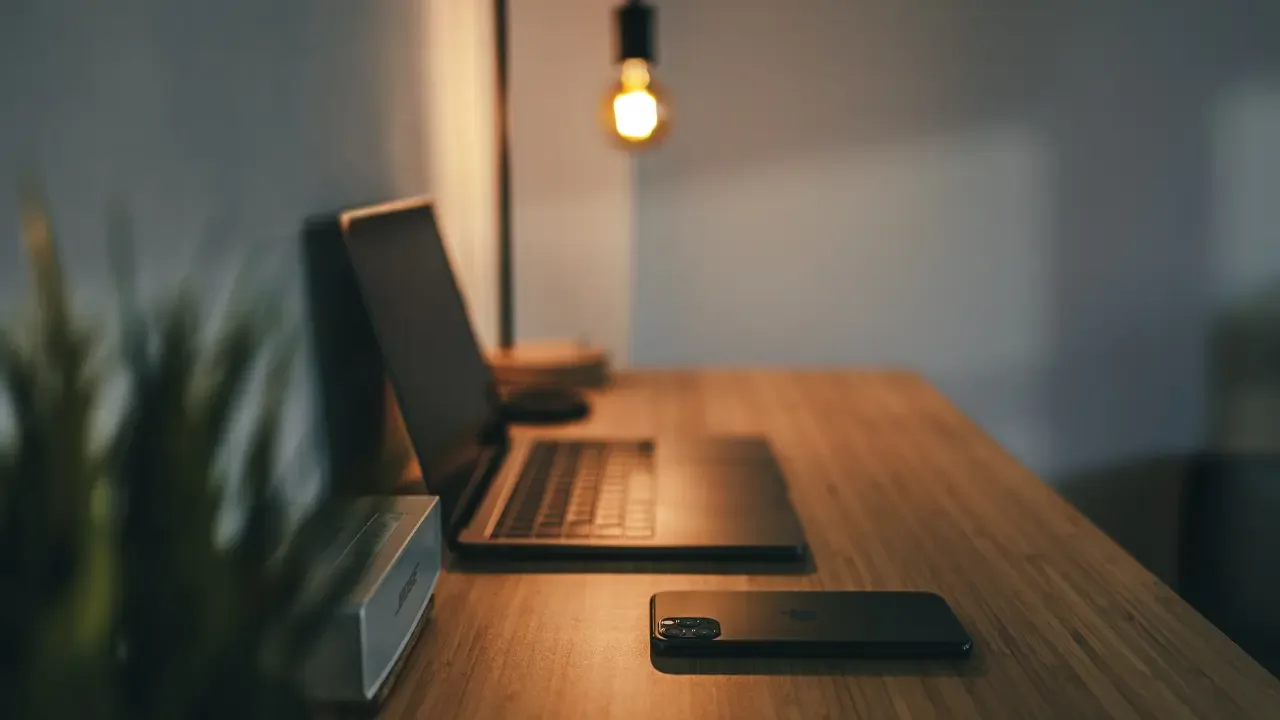
Understanding the Error: type 'List<dynamic>' is not a subtype of type 'List<Widget>'
So, you've got some code that you copied and pasted from a Firestore example and you're encountering an error that says: type 'List<dynamic>' is not a subtype of type 'List<Widget>'
. 🤔
This error typically occurs when there's a mismatch between the expected type and the actual type being used. In this case, it seems that you're trying to assign a list of dynamic objects (List<dynamic>
) to a property or variable expecting a list of Widget
objects (List<Widget>
).
Let's break it down and find a solution! 💪
Understanding the Code
First, let's take a closer look at the code snippet you provided:
Widget _buildBody(BuildContext context) {
return new StreamBuilder(
stream: _getEventStream(),
builder: (context, snapshot) {
if (!snapshot.hasData) return new Text('Loading...');
return new ListView(
children: snapshot.data.documents.map((document) {
return new ListTile(
title: new Text(document['name']),
subtitle: new Text("Class"),
);
}).toList(),
);
},
);
}
In this code, you're using a StreamBuilder
to build a widget that listens to a stream (_getEventStream()
) and rebuilds itself whenever new data arrives. Inside the builder, you're checking whether the snapshot
has data and returning either a loading indicator (Text('Loading...')
) or a ListView
containing a list of ListTile
widgets.
Identifying the Problem
The error you're encountering indicates that the children
property of the ListView
is expecting a List<Widget>
, but you're providing a List<dynamic>
. This suggests that the documents.map()
function is returning a list of dynamic objects instead of widgets.
Finding the Solution
To resolve this issue, you need to ensure that the map()
function returns a list of widgets. In this case, you can explicitly convert each document
into a ListTile
widget using the toList()
function.
Here's the modified code:
Widget _buildBody(BuildContext context) {
return StreamBuilder(
stream: _getEventStream(),
builder: (context, snapshot) {
if (!snapshot.hasData) return Text('Loading...');
return ListView(
children: snapshot.data.documents.map<Widget>((document) {
return ListTile(
title: Text(document['name']),
subtitle: Text("Class"),
);
}).toList(),
);
},
);
}
By specifying <Widget>
as the generic type argument for the map()
function (map<Widget>((document) {...
) and using the toList()
function, you ensure that the children
property of the ListView
receives a List<Widget>
, thus resolving the error.
The Call-to-Action: Let's Engage!
I hope this solution helped you resolve the type 'List<dynamic>' is not a subtype of type 'List<Widget>'
error. 💡
If you found this blog post helpful, don't forget to share it with your fellow developers who might encounter a similar issue. 😄❤️
Feel free to leave a comment below if you have any questions or if there's anything else I can assist you with. Happy coding! 🚀👨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
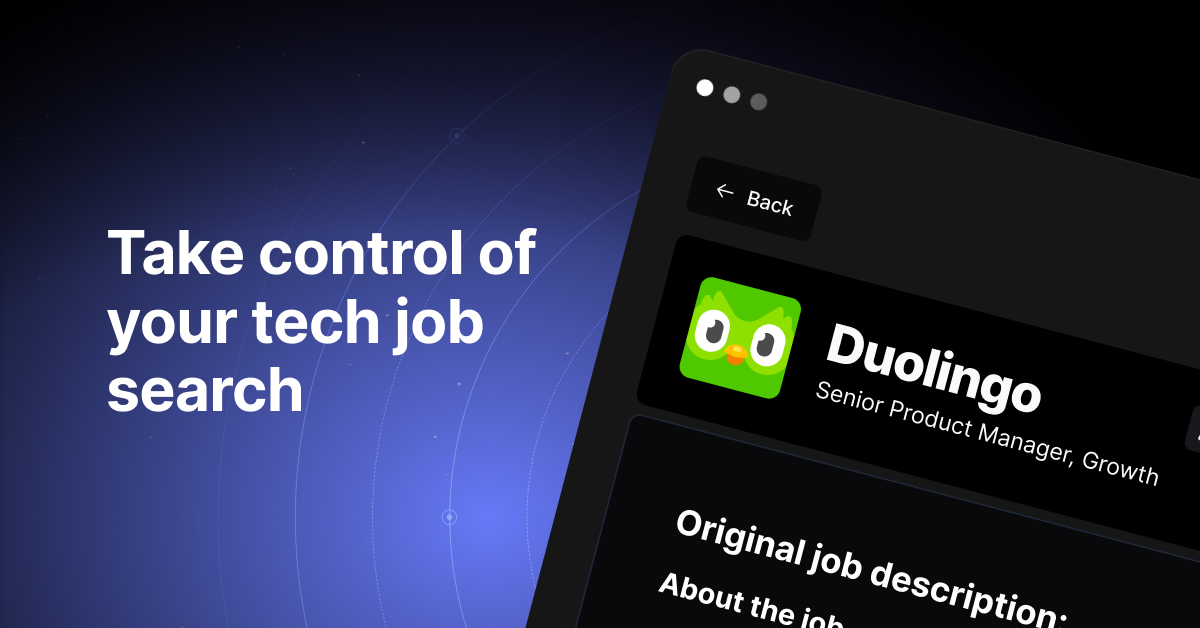