How to create an Excel File with Nodejs?
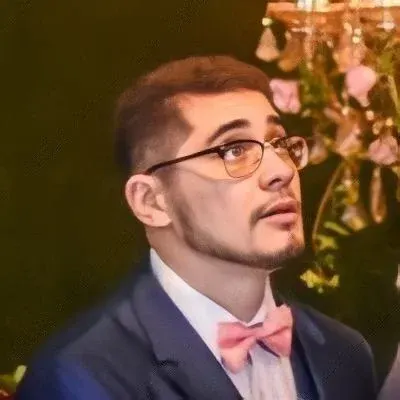
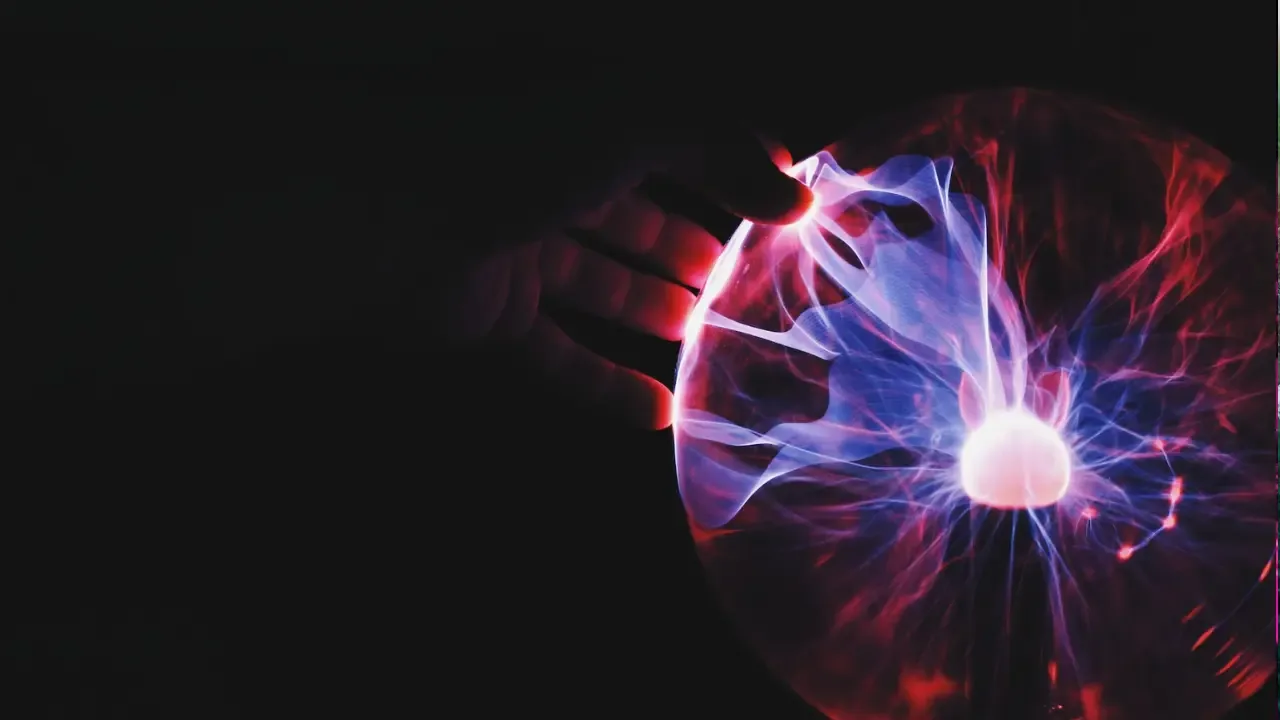
How to Create an Excel File with Node.js 📂
So you're a Node.js programmer, with a table of data ready to be saved in Excel file format. You've come to the right place! In this blog post, we'll explore some common issues faced in creating Excel files using Node.js, provide easy solutions, and guide you through the process step-by-step. Let's get started! 🚀
The Challenge: Finding the Right Node.js Library 📚
As mentioned, finding a suitable library for creating Excel files in Node.js can be tricky. While there are plenty of Excel parsing libraries available, the options for Excel writing are relatively limited. And if you're using a Linux server, you need a library that's compatible with your environment. Fear not! We've got you covered.
Solution 1: Excel4node 📊
One excellent library that meets our requirements is Excel4node. It's easy to use, comprehensive, well-documented, and runs smoothly on Linux servers. This library allows you to generate an Excel file directly from your Node.js code.
To install Excel4node, simply run the following command in your terminal:
npm install excel4node
Once installed, you can start integrating it into your project immediately. Here's an example to demonstrate how easy it is to create an Excel file using Excel4node:
const Excel = require('excel4node');
const workbook = new Excel.Workbook();
const worksheet = workbook.addWorksheet('Sheet 1');
worksheet.cell(1, 1).string('Hello, World!');
workbook.write('output.xlsx');
In this example, we create a new workbook, add a worksheet named 'Sheet 1', write a string value to cell A1, and finally save the workbook as 'output.xlsx'. It's that simple! 🙌
Solution 2: Converting a CSV File to an Excel File 🔄
Alternatively, if you already have your data in a CSV file and want to convert it to an XLS file programmatically, csvtoexcel is a great solution. csvtoexcel is another powerful Node.js library that runs perfectly fine on Linux servers.
To install csvtoexcel, run the following command:
npm install csvtoexcel
Here's an example of how you can convert a CSV file to an Excel file using csvtoexcel:
const csv = require('csvtoexcel');
csv()
.fromFile('input.csv')
.then((excelFile) => {
console.log('Conversion complete!', excelFile);
})
.catch((err) => {
console.error('Conversion failed:', err);
});
In this example, we use the .fromFile()
method to specify the CSV file we want to convert, and the .then()
method will log the completion of the conversion, along with the path to the new Excel file. If any errors occur during the conversion process, they will be caught and logged using the .catch()
method.
Your Turn! Engage and Share with Us! 💬📢
Now that you have the tools to create Excel files with Node.js, it's time to put your skills to action! Whether you choose to go with Excel4node or csvtoexcel, we'd love to hear about your experiences and how these libraries worked for you.
Do you have other Node.js libraries or techniques you'd like to share for creating Excel files? Any challenges you faced along the way? Let us know in the comments below! Let's help each other excel (pun intended 😉) in Node.js file creation!
Keep exploring, learning, and coding! ✨💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
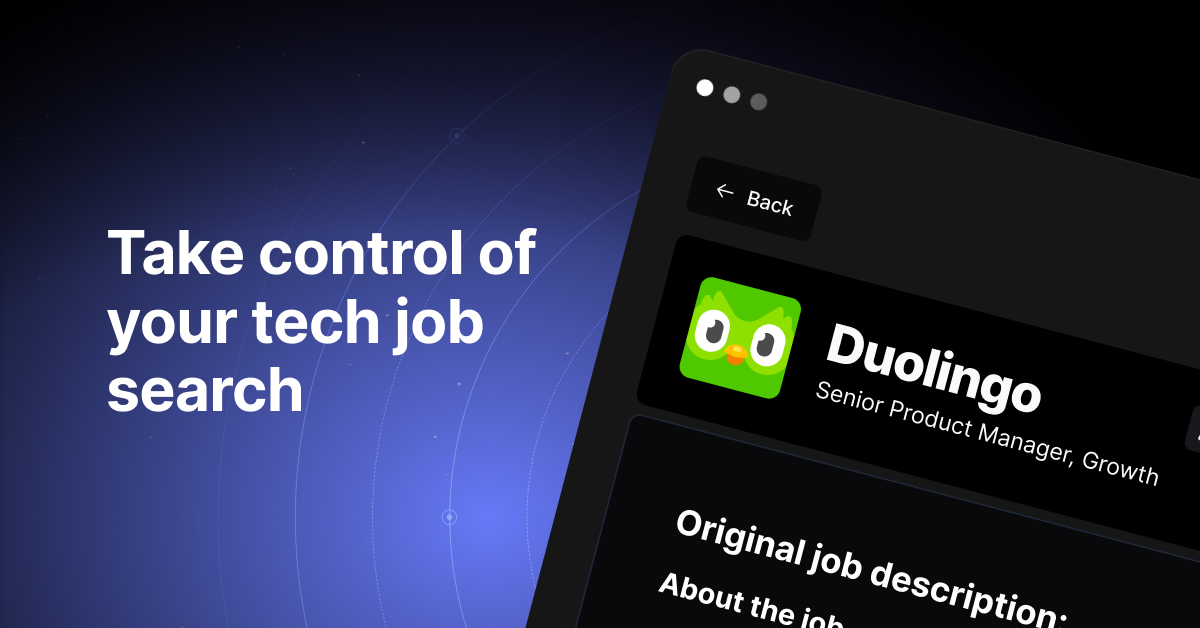