How to solve Flutter CERTIFICATE_VERIFY_FAILED error while performing a POST request?
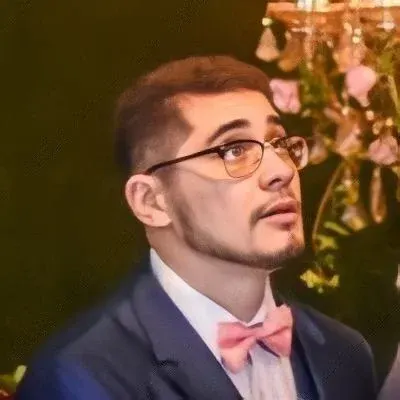
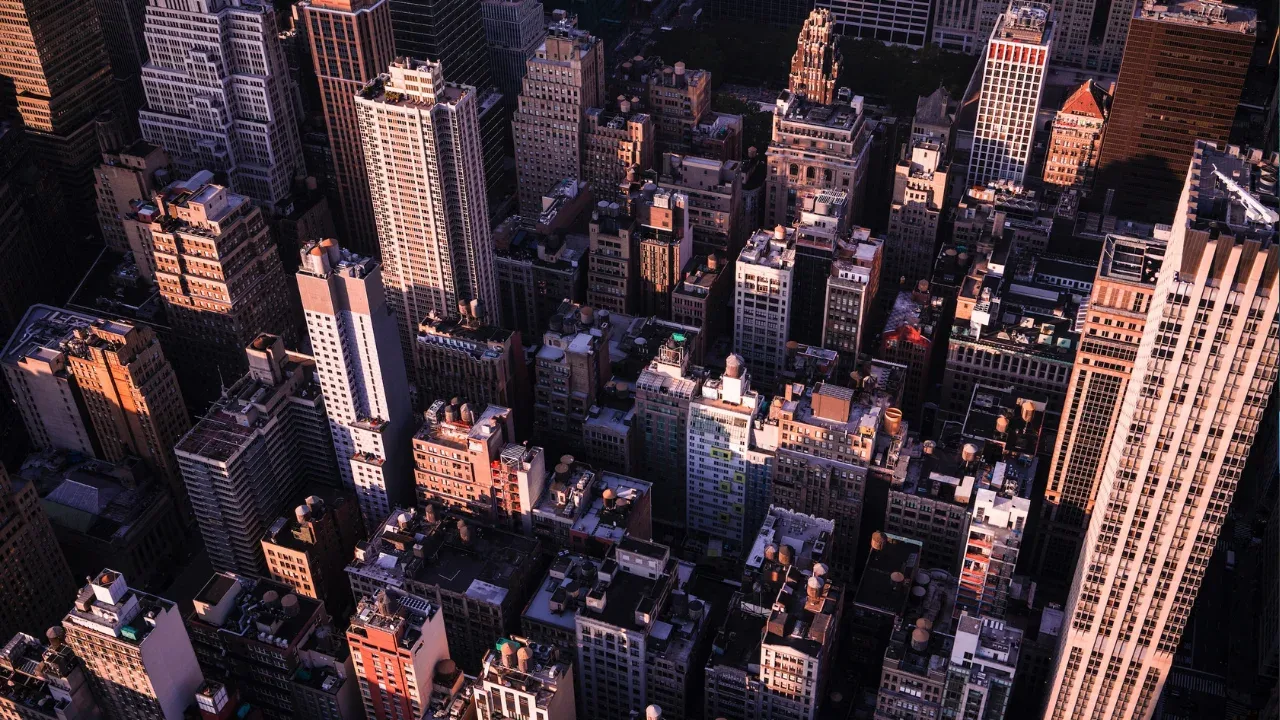
👋 Hey there, Flutter enthusiast! 😄 Are you experiencing the dreaded CERTIFICATE_VERIFY_FAILED
error while trying to perform a POST request in your Flutter app? Don't fret! 🙌 This blog post is here to help you solve this issue in a jiffy. Let's dive right in, shall we? 💪
Understanding the Problem
The CERTIFICATE_VERIFY_FAILED
error occurs when the SSL/TLS certificate presented by the server during the handshake process cannot be verified by the client. This often happens when the client is unable to find the necessary root certificates to establish a secure connection.
Common Causes
There are a few common causes for this error:
Missing Root Certificates: The client may not have the required root certificates installed to verify the server's certificate.
Invalid or Self-Signed Certificates: The server may be using an invalid or self-signed certificate that is not trusted by the client by default.
Hostname Mismatch: The hostname in the certificate does not match the actual hostname of the server.
Easy Solutions
Now that we know what might be causing the error, here are three easy solutions to fix it:
Solution 1: Bypass SSL Verification (Not recommended for production)
If you are using Flutter for development purposes, you can opt to bypass SSL verification to establish a connection without validating the server's certificate. However, keep in mind that this approach is not suitable for production environments, as it undermines the security of the connection.
To bypass SSL verification, you can make use of the http
package's IOClient
and HttpClient
classes as follows:
import 'dart:io';
import 'package:http/http.dart' as http;
void main() {
// Bypass SSL verification
HttpClient httpClient = HttpClient()
..badCertificateCallback = ((X509Certificate cert, String host, int port) => true);
final ioClient = http.IOClient(httpClient);
// Use ioClient for your requests
}
Solution 2: Install Root Certificates
If the error is due to missing root certificates, you can manually add them to your Flutter app. Here's how:
Visit the website of the server you are trying to connect to in your browser.
View the certificate details (usually found in the browser's security options).
Download the root certificate (usually in PEM or CRT format).
Create a
certs
folder in your Flutter project's root directory.Move the downloaded root certificate file into the
certs
folder.Update your
pubspec.yaml
file to include the certificate in your app's assets:
flutter:
assets:
- certs/
In your Dart code, use the
rootBundle
to load the certificate and provide it to thehttp
package:
import 'dart:io';
import 'package:http/http.dart' as http;
import 'package:flutter/services.dart' show rootBundle;
void main() async {
// Load the certificate
final certFileData = await rootBundle.load('certs/my_root_certificate.crt');
SecurityContext securityContext = SecurityContext.defaultContext;
securityContext.setTrustedCertificatesBytes(certFileData.buffer.asUint8List());
final httpClient = http.Client()
..forceUgrade = true
..badCertificateCallback = ((X509Certificate cert, String host, int port) => true)
..context = securityContext;
// Use httpClient for your requests
}
Solution 3: Use a SafeHTTP Package
Alternatively, you can use a safe HTTP package that handles certificate validation for you and simplifies the process. One such package is the flutter_secure_http
package.
To use flutter_secure_http
, add it to your pubspec.yaml
file:
dependencies:
flutter_secure_http: ^3.0.0
Here's an example of how to use it:
import 'package:flutter_secure_http/flutter_secure_http.dart';
void main() {
final http = FlutterSecureHttp();
// Use http for your requests
}
Wrapping It Up
And there you have it! Three easy solutions to help you resolve the CERTIFICATE_VERIFY_FAILED
error in Flutter. You can choose the approach that best suits your requirements and get back to coding awesomeness in no time! 💥
If you found this blog post helpful, don't forget to share it with other Flutter developers facing the same challenge. 😊 And if you have any questions or other tricks up your sleeve, share them in the comments below. Let's solve problems and build amazing Flutter apps together! 🚀✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
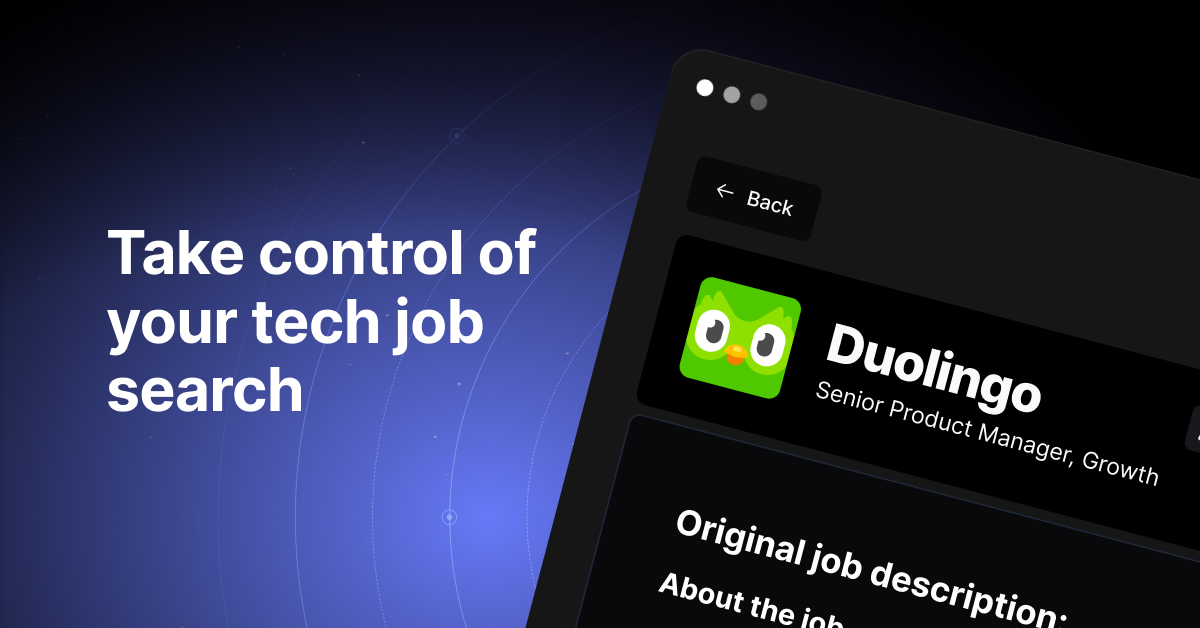