How to make an AlertDialog in Flutter?
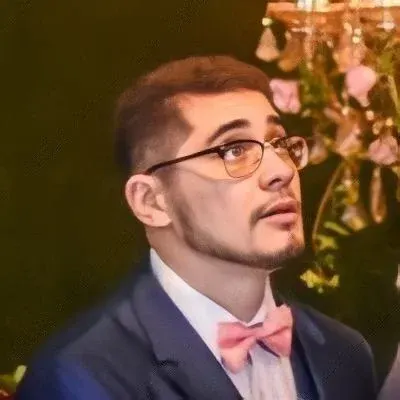
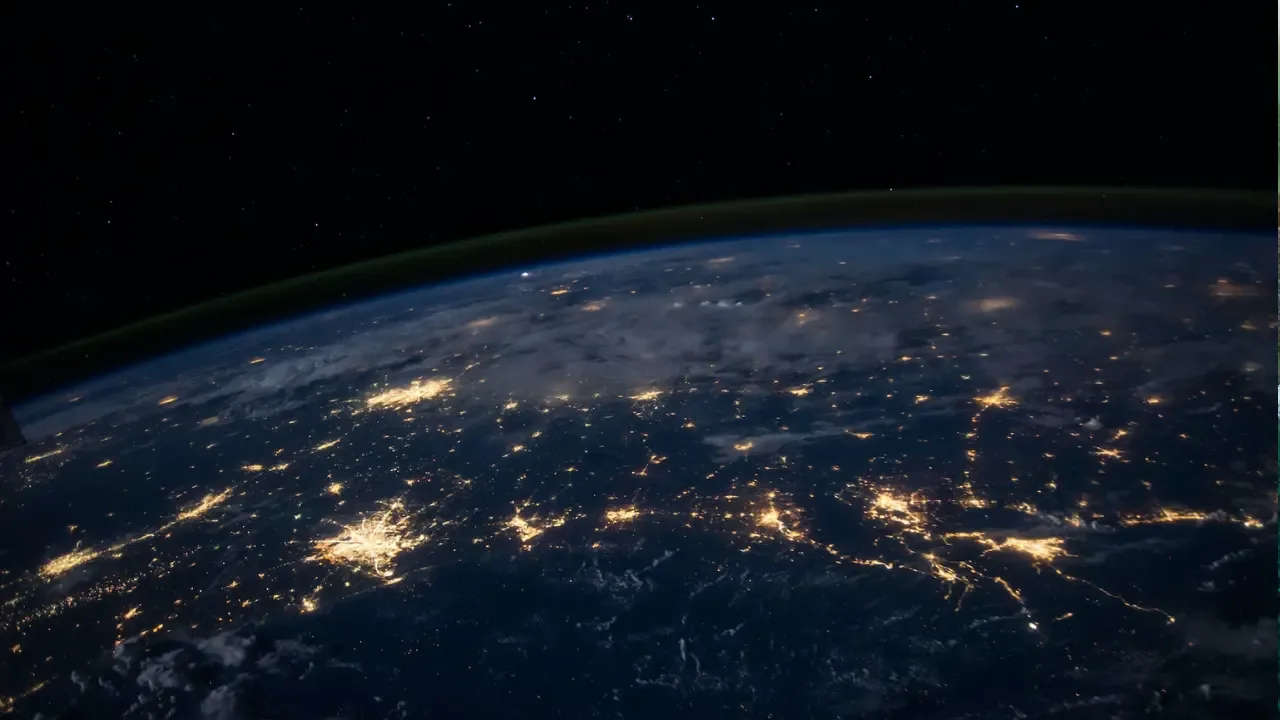
How to Make an AlertDialog in Flutter?
Alert dialogs are essential components in mobile app development, as they help provide important information, confirmations, or prompts to users. If you're learning to build apps in Flutter and wondering how to create an alert dialog, you've come to the right place! In this blog post, we'll walk you through the process of making an AlertDialog in Flutter and tackle some common issues along the way. Let's get started! 🚀📲
Setting up the Environment 🛠️
Before creating an AlertDialog, make sure you have Flutter and the necessary dependencies installed on your machine. If you haven't yet set up your Flutter environment, check out the official Flutter installation guide to get started.
Creating an AlertDialog
To create an AlertDialog in Flutter, follow these steps:
Import the required packages:
import 'package:flutter/material.dart';
Create a function to show the AlertDialog:
Future<void> _showAlertDialog(BuildContext context) async {
return showDialog<void>(
context: context,
barrierDismissible: false, // dialog is not dismissible by clicking outside
builder: (BuildContext context) {
return AlertDialog(
title: Text('My Alert Dialog'),
content: SingleChildScrollView(
child: ListBody(
children: <Widget>[
Text('This is a sample alert dialog.'),
Text('You can add more content here.'),
],
),
),
actions: <Widget>[
TextButton(
child: Text('OK'),
onPressed: () {
Navigator.of(context).pop(); // close the dialog
},
),
],
);
},
);
}
Trigger the AlertDialog:
_showAlertDialog(context);
Handling Common Issues 🛠️🐛
Customizing Actions in an AlertDialog 🎨✍️
To customize the actions in your AlertDialog, such as styling or adding additional buttons, you can modify the actions
property of the AlertDialog widget. For example:
actions: <Widget>[
ElevatedButton(
child: Text('Custom Action'),
onPressed: () {
// Handle custom action
},
),
OutlinedButton(
child: Text('Cancel'),
onPressed: () {
Navigator.of(context).pop(); // close the dialog
},
),
],
Adding a Dropdown Menu in an AlertDialog 🌐📝
To add a dropdown menu to your AlertDialog, you can use the DropdownButton
widget as the content of the dialog. For example:
content: Column(
children: <Widget>[
Text('Select an option:'),
DropdownButton<String>(
value: dropdownValue,
onChanged: (String newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: <String>['Option 1', 'Option 2', 'Option 3', 'Option 4']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
],
),
Displaying an AlertDialog Automatically on App Launch ⚡🖥️
To show an AlertDialog automatically when the app loads, you can call the _showAlertDialog
function in the initState
method of your app's initial screen. For example:
@override
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((_) {
_showAlertDialog(context);
});
}
Styling an AlertDialog with Rounded Corners 🎨🔘
To style an AlertDialog with rounded corners, you can wrap it in a Container
widget and apply a custom border radius. For example:
builder: (BuildContext context) {
return Container(
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(16.0),
),
child: AlertDialog(
// ...
),
);
},
Conclusion 🎉🚀
Congratulations! You've learned how to make an AlertDialog in Flutter and handle common issues along the way. AlertDialogs are powerful tools to engage your app users, provide necessary information, and collect user input. Now it's time to incorporate this knowledge into your own Flutter projects! If you have any questions or further tips to share, feel free to leave a comment below. Happy coding! 😄💻
References:
Feel free to share this blog post with your friends on social media using the buttons below. Happy Fluttering! 🙌📱
💬💡🚀📲🛠️📩😲🔘🌐📝⚡️✍️🎨🎉😄💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
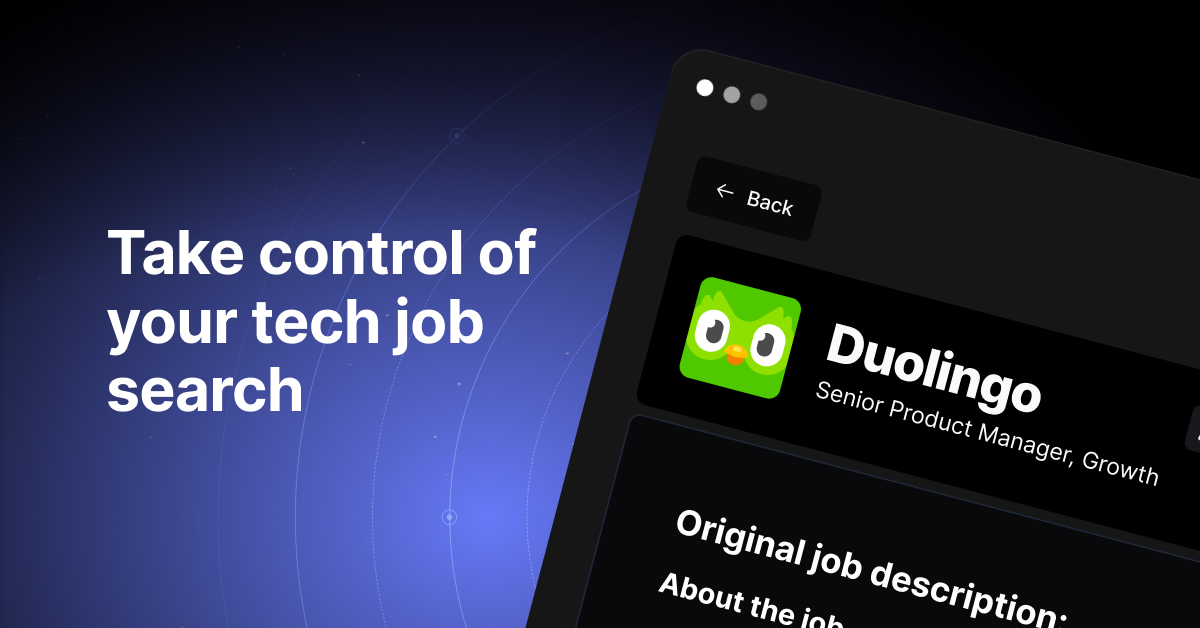