What REALLY happens when you don"t free after malloc before program termination?
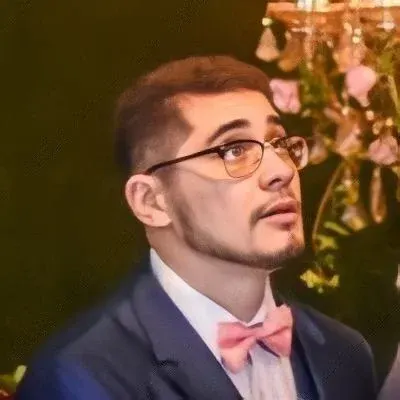
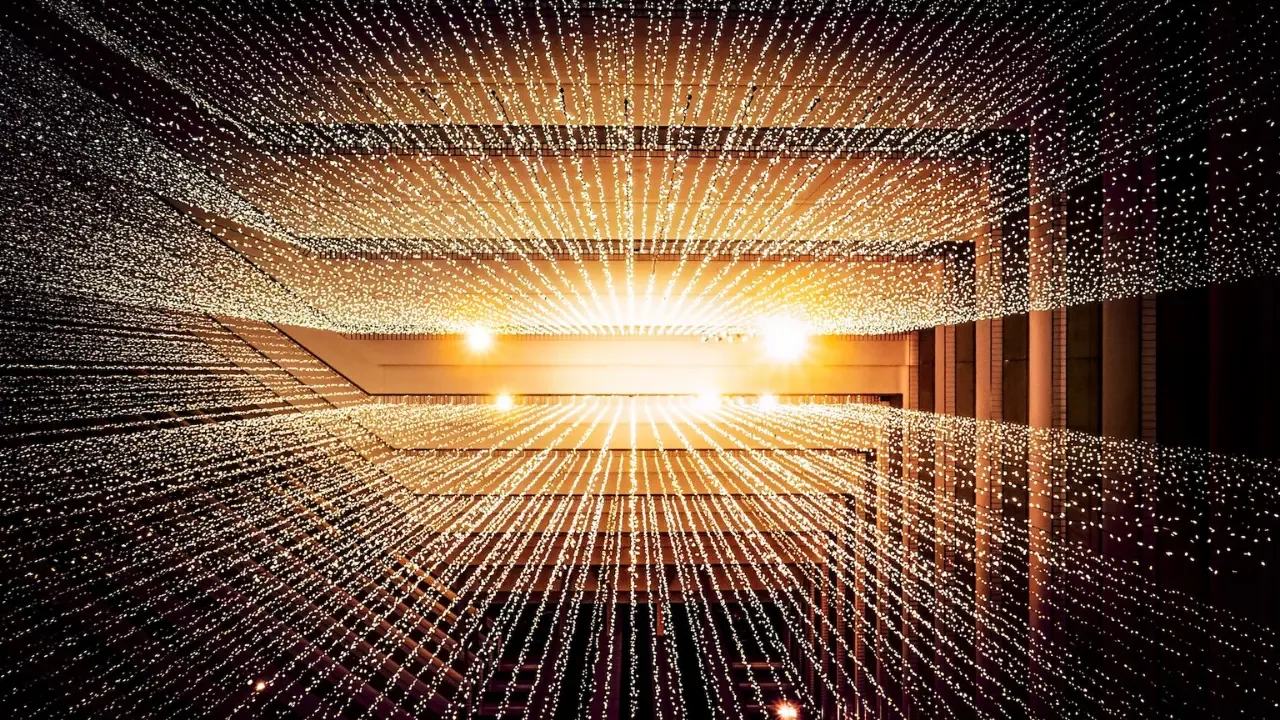
The Real Deal with Not Freeing Memory After Malloc
š§ Ever wondered what REALLY happens when you don't free memory after allocating it with malloc? Is it actually a big deal, or can you get away with skipping this step? Let's dig into this topic and unravel the mysteries surrounding it! š„
š¼ Common Issues and Concerns
Two common scenarios arise when considering whether to free memory after allocating it:
1ļøā£ Case 1: Memory Allocation within a Single Block
Imagine you have a code snippet like this:
int main()
{
char *a = malloc(1024);
/* Do some arbitrary stuff with 'a' (no alloc functions) */
return 0;
}
ā So, what happens in this case? Does it really matter if we skip the call to free
?
š¤ Technically, when the program terminates (with or without explicitly calling free
), all allocated memory is automatically freed by the operating system. This means that the heap space utilized by the allocated a
will be reclaimed. So, from a memory perspective, you might argue that skipping the call to free
in this case doesn't have a significant impact.
š” However, it is essential to note that even though skipping the deallocation might not cause memory leaks, it's still considered good practice to free memory explicitly. It promotes code clarity, maintainability, and reduces the risk of introducing issues in the future.
2ļøā£ Case 2: Dynamic Data Structures
Let's dive into a different scenario now. Consider a program that acts like a shell and allows users to declare variables:
typedef struct {
char *name;
int value;
} Variable;
Variable *variables = NULL;
void addVariable(const char *name, int value)
{
Variable *var = malloc(sizeof(Variable));
var->name = strdup(name);
var->value = value;
// Append var to the list of variables
// e.g., using a linked list or hashmap
// Don't free var here
}
int main()
{
/* ... some program logic ... */
addVariable("aaa", 123);
/* ... more program logic ... */
return 0;
}
š¼ In this case, variables are stored in a dynamic data structure (e.g., linked list or hashmap), and it doesn't seem practical to free the memory immediately after allocation. Instead, these variables need to persist throughout the program's execution.
š« However, completely relying on program termination to free the memory is generally considered bad design. It can lead to memory leaks and hinder the overall performance of the program.
š ļø The Alternative Solution
š Instead of delaying the deallocation until program termination, a cleaner and more efficient approach is to explicitly free the memory when it is no longer needed.
In the first scenario, where memory is allocated within a single block, it's best to free it once you're done using it. It ensures good code practices and future-proofing your program.
In the second scenario, rather than waiting until program termination, you can implement a cleanup function that deallocates the variables as needed. For example, you can have a function called
cleanupVariables
that frees all the dynamically allocated variables:
void cleanupVariables()
{
Variable *curr = variables;
while (curr != NULL) {
Variable *next = curr->next;
free(curr->name);
free(curr);
curr = next;
}
variables = NULL;
}
You can then invoke this function whenever required, such as before exiting the program or during specific cleanup operations.
š The Call-to-Action: Engaging with Your Thoughts
š¢ Now that you've learned about the consequences of not freeing memory after malloc, we want to hear your thoughts! Do you think it's okay to skip the free step in certain cases, or do you believe in always following best practices? Share your insights in the comments below and let's start a conversation! ā¬ļø
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
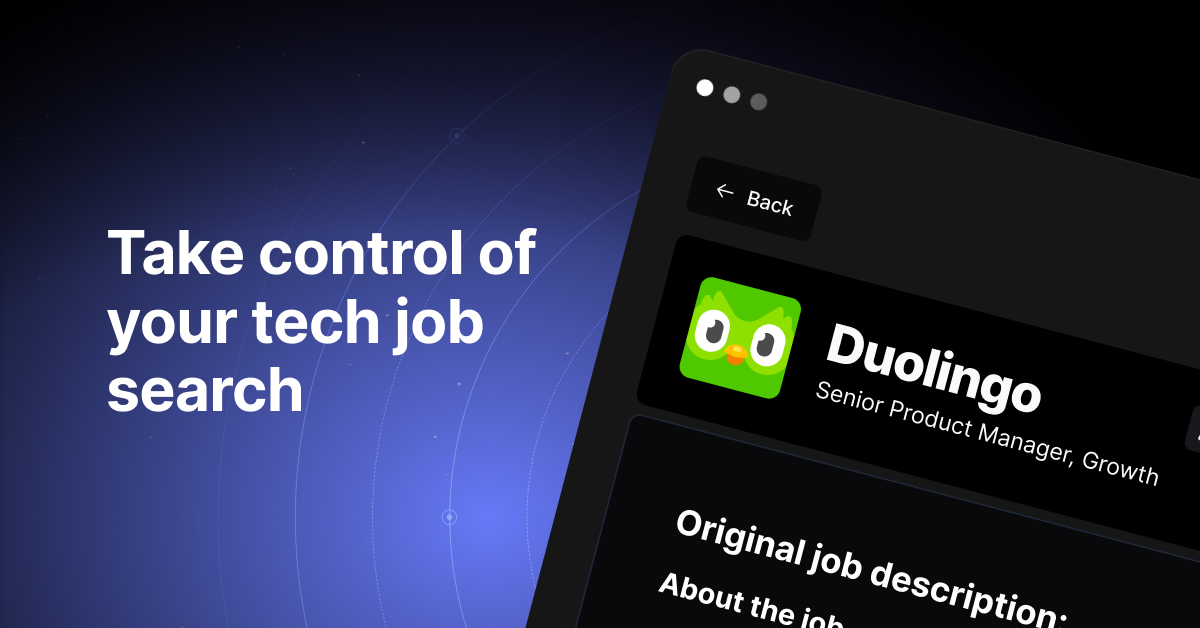