How do you pass a function as a parameter in C?
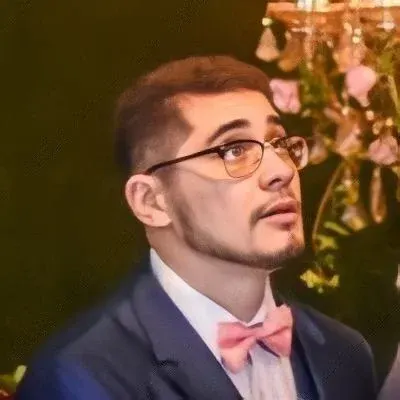
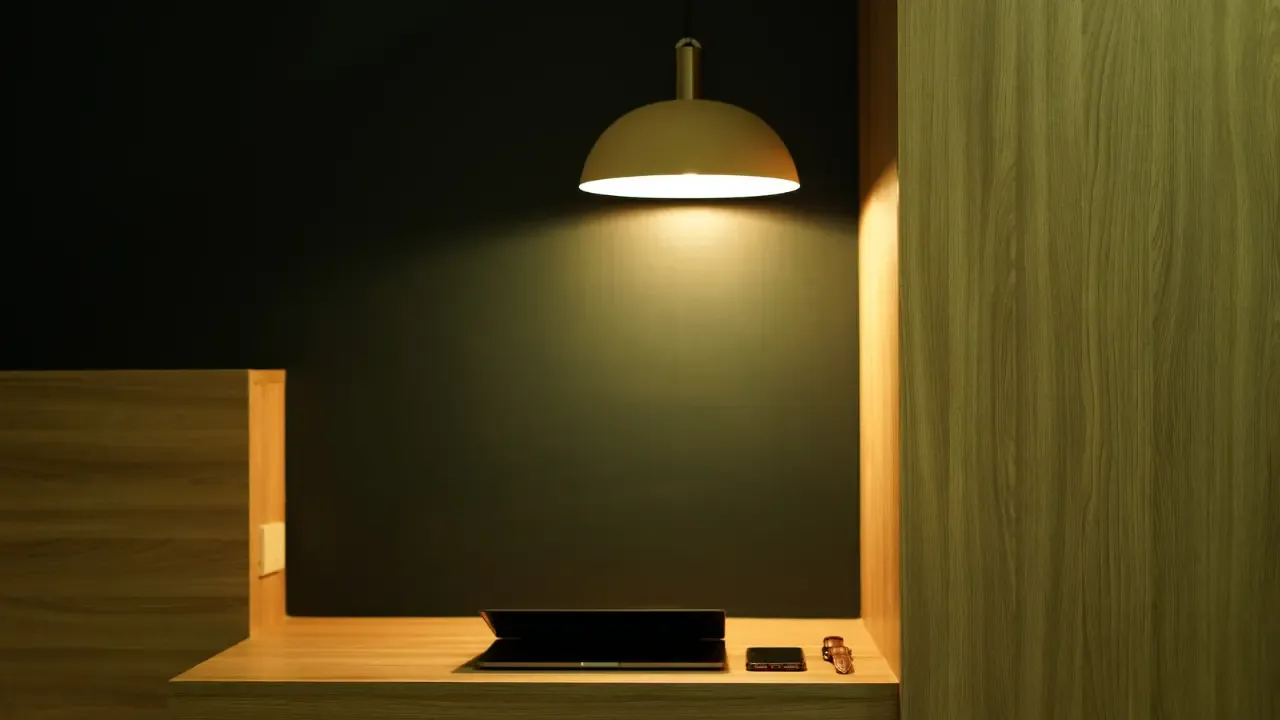
How to Pass a Function as a Parameter in C: A Complete Guide 😎
Have you ever wondered how to pass a function as a parameter in C? 🤔 It's a common question that often puzzles programmers but fear not! We're here to break it down for you in a clear and concise way. By the end of this guide, you'll be passing functions around like a pro! 💪
Understanding the Basics 📚
In C, functions are treated as first-class citizens, meaning they can be treated just like any other variable. This allows us to pass functions as parameters to other functions, an incredibly powerful feature that can lead to more modular and reusable code. 🙌
Defining Function Pointers 👇
Before we jump into passing functions as parameters, we need to understand function pointers. A function pointer is a variable that stores the memory address of a function. Here's an example of how to define a function pointer in C:
// Function prototype
void myFunction();
// Function pointer definition
void (*functionPointer)() = myFunction;
In the example above, we defined a function pointer named functionPointer
that points to a function with no arguments and a void
return type.
Passing a Function as a Parameter 🎁
Now that we know how to define function pointers, let's see how we can pass functions as parameters to other functions. 👩💻
// Function that takes a function pointer as a parameter
void performOperation(void (*operation)()) {
// Call the function using the function pointer
operation();
}
// Function to be passed as a parameter
void myOperation() {
// Do something
}
int main() {
// Pass myOperation as a parameter to performOperation
performOperation(myOperation);
return 0;
}
In the example above, we have a function called performOperation
that takes a function pointer named operation
as a parameter. Inside performOperation
, we simply call the function using the function pointer. Then, in main()
, we pass our desired function, myOperation
, as a parameter to performOperation
.
Common Pitfalls to Avoid 🚧
While passing functions as parameters in C can be a powerful technique, there are a few common pitfalls to be aware of. Here are a few tips to help you avoid potential issues:
Make sure the function signatures match: The function pointer's signature must match the signature of the function being passed as a parameter. Otherwise, you'll encounter errors.
Mind the parentheses: Remember to include parentheses after the function pointer name when calling it within the function that received it as a parameter.
Take Your Code to the Next Level! 🚀
Passing functions as parameters in C opens up a world of possibilities for code reuse and modularity. Now that you have a solid understanding of how to pass functions as parameters, take your code to the next level!
Start by identifying repetitive code that can be abstracted into separate functions. Then, consider how passing functions as parameters can help make your code more flexible and adaptable.
Remember, practice makes perfect! So, go ahead and experiment with passing functions as parameters in your C code. 🤓
Got any cool projects or code examples utilizing this concept? Share them with our community in the comments below! Let's learn from each other and level up our coding skills together. 💻💬
Keep coding and keep exploring! Happy programming! 🌟
P.S. If you found this guide helpful, don't forget to share it with your developer friends who might need a little guidance on passing functions as parameters in C. 😉
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
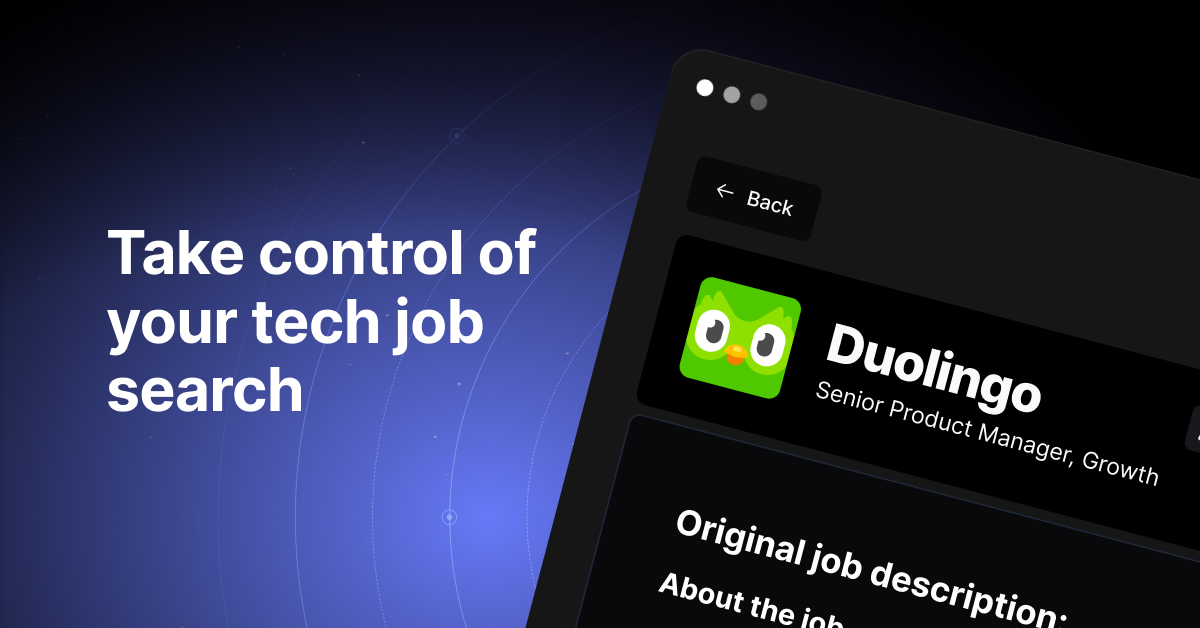