How to write unit testing for Angular / TypeScript for private methods with Jasmine
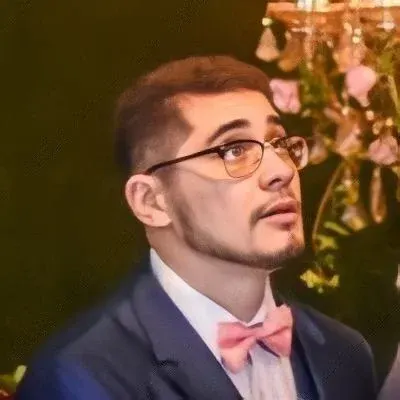
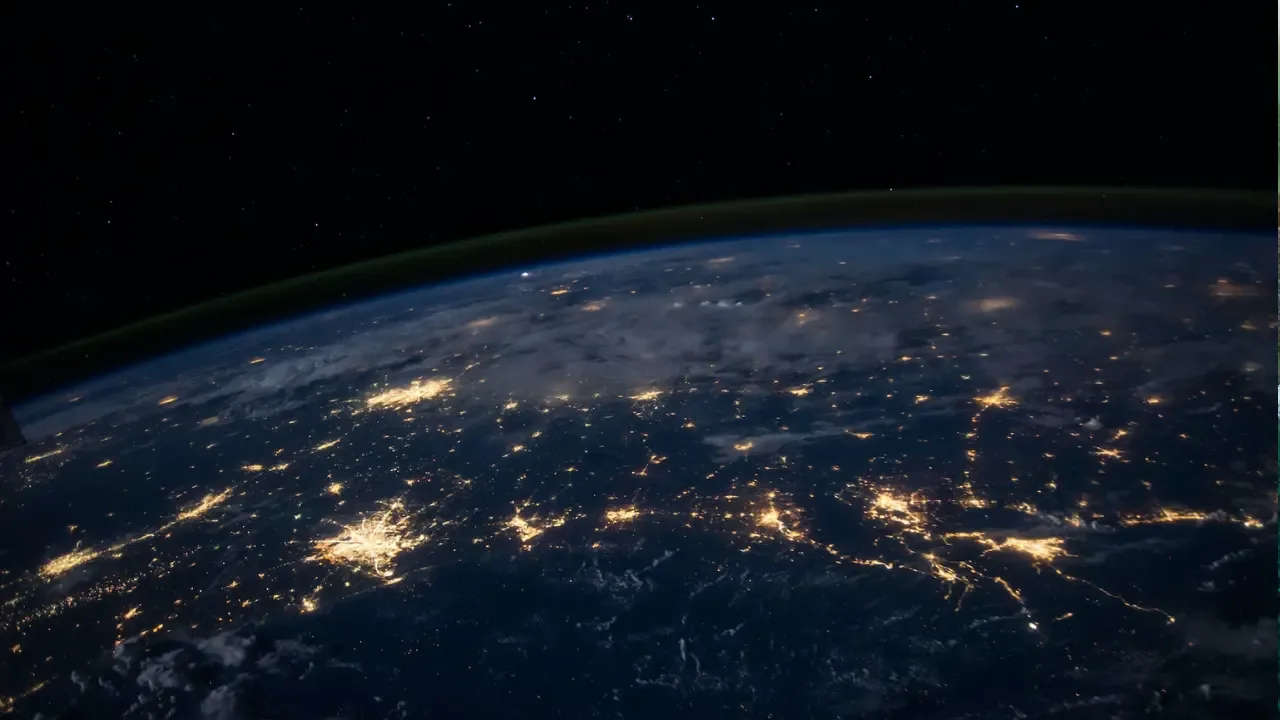
How to Write Unit Testing for Angular / TypeScript for Private Methods with Jasmine 💻
Testing private methods in Angular / TypeScript can be tricky since they are not directly accessible outside of the class. However, there are a couple of solutions that you can use to effectively write unit tests for private methods using Jasmine. In this blog post, we will explore one common approach and also invite readers to share their own solutions.
The Problem 💥
Let's start by examining the problem at hand. Say we have a class called FooBar
with a private method called initFooBar
. This private method is being called in the constructor, and we want to write unit tests specifically for this private method. Here's a simplified code snippet to illustrate the scenario:
class FooBar {
private _status: number;
constructor(private foo: Bar) {
this.initFooBar();
}
private initFooBar() {
this.foo.bar("data");
this._status = this.foo.foo();
}
public get status() {
return this._status;
}
}
As you can see, the private method initFooBar
is essential for the functionality of the FooBar
class. Now, let's explore a solution to write unit tests for this private method.
The Solution 💡
Option 1: Closure Technique
One approach to testing private methods is to put the test code itself inside the closure or add code inside the closure that stores references to the local variables on existing objects in the outer scope. This technique allows you to access and test private methods indirectly. However, it's worth noting that this solution might require additional tooling to strip out the test code in the production build.
Here's an example of how you can utilize the closure technique to test the private method initFooBar
:
describe('FooBar', () => {
let fooBar: FooBar;
let fooMock: jasmine.SpyObj<Bar>; // Using jasmine.SpyObj for mocking purposes
beforeEach(() => {
fooMock = jasmine.createSpyObj('Bar', ['bar', 'foo']);
fooBar = new FooBar(fooMock);
});
it('should properly initialize _status', () => {
fooBar['initFooBar'](); // Accessing the private method using ['methodName']
expect(fooBar['status']).toBe(/* expected value */);
});
// ... More tests for other scenarios related to initFooBar
});
In this example, we create a mock object for Bar
using Jasmine's createSpyObj
function. Then, in the beforeEach
block, we instantiate FooBar
and pass the mock object as a dependency. Inside the test case, we directly access the private method by using fooBar['initFooBar']()
notation and assert the expected outcome.
Share Your Solution ✨
We have presented one solution to test private methods in Angular / TypeScript using Jasmine. However, we understand that there might be alternative ways to tackle this problem. We encourage readers to share their own solutions or provide feedback on the provided solution.
Conclusion 🌟
Effective unit testing in Angular / TypeScript relies on the ability to test private methods. By using the closure technique or other creative solutions, it's possible to access and test private methods indirectly. Although testing private methods might not be a common practice, certain scenarios might require it. Keep experimenting and finding the best approach for your specific use case.
P.S.:
We have ensured to find a solution for the problem at hand, as some similar questions often go unanswered.
While some developers argue against testing private methods, we acknowledge that there are specific necessities where testing private methods becomes valuable.
Now it's your turn! Have you encountered a similar problem? How did you approach it? Share your solutions or thoughts in the comments below and let's discuss! 👇👇
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
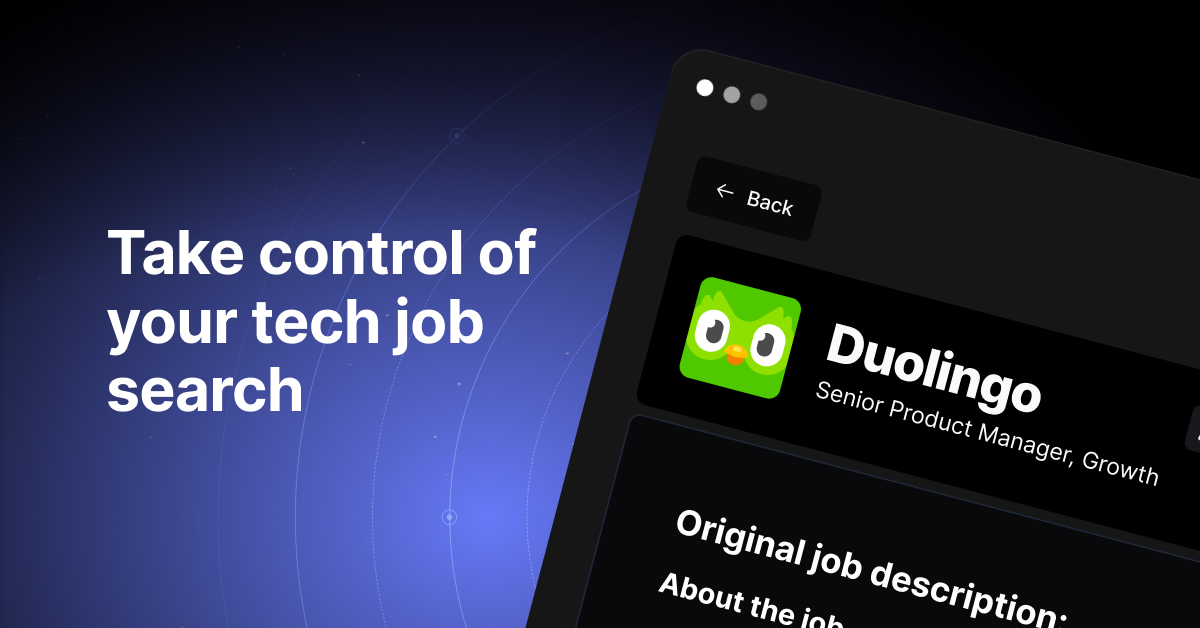