How to implement sleep function in TypeScript?
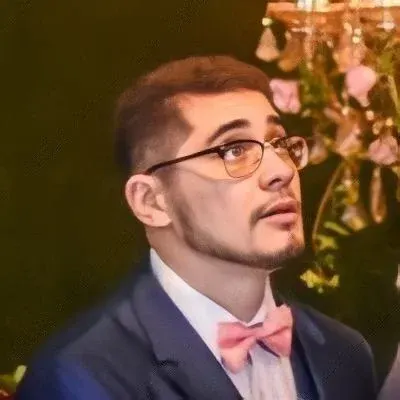
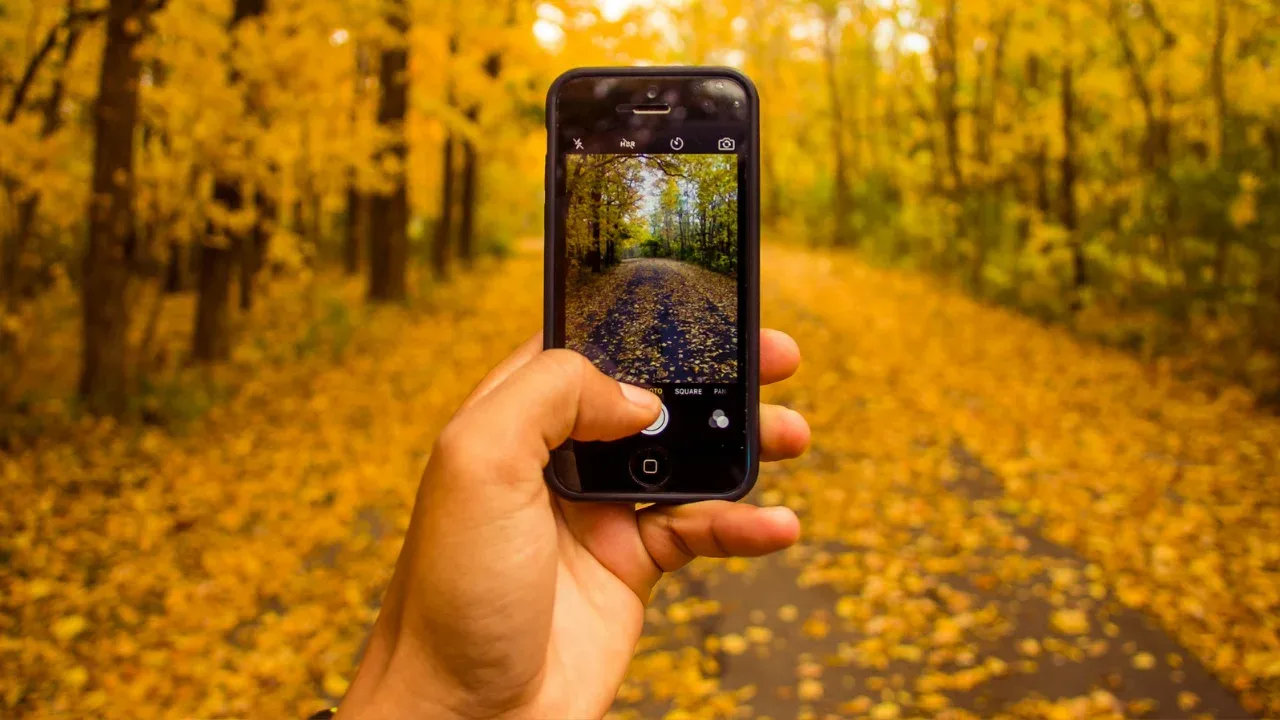
😴 How to Implement Sleep Function in TypeScript?
Have you ever been in a situation where you wanted to pause the execution of your code for a specific amount of time? Maybe you wanted to redirect users to a different page after a certain delay or simulate a loading spinner. If you're developing a website using Angular 2 and TypeScript, you might find yourself wondering how to implement the thread.sleep(ms)
functionality.
In this blog post, we'll tackle this common issue and provide you with easy solutions to implement a sleep function in TypeScript. By the end, you'll be equipped with the knowledge to handle delays in your code effortlessly. Let's dive in! 💪
The Problem: Implementing a Sleep Function in TypeScript
One of our readers asked us this question: "I'm developing a website in Angular 2 using TypeScript, and I was wondering if there was a way to implement thread.sleep(ms)
functionality."
Their use case was straightforward. They wanted to redirect users to a different page after submitting a form and introduce a brief delay to provide some visual feedback. While achieving this with JavaScript is a breeze, it's not immediately clear how to accomplish it with TypeScript.
The Solution: Using Promises and Async/Await
To implement a sleep function in TypeScript, we can leverage the power of Promises and the async/await syntax. Here's an example of how you can achieve this:
function sleep(ms: number): Promise<void> {
return new Promise((resolve) => setTimeout(resolve, ms));
}
async function redirectAfterDelay() {
// Delay for 3 seconds
await sleep(3000);
// Perform the redirection here
// e.g., window.location.href = 'https://example.com';
}
// Call the function
redirectAfterDelay();
In this example, we define a sleep
function that returns a Promise. The function takes the desired delay in milliseconds as an argument and uses setTimeout
to resolve the Promise after the specified time has passed.
The redirectAfterDelay
function demonstrates how to use the sleep function. By marking it as async
, we can use the await
keyword to pause the execution until the Promise is resolved. After the desired delay, you can perform the redirection or any other action you need.
Wrapping Up
Implementing a sleep function in TypeScript can be accomplished using Promises and async/await syntax. By applying the solution demonstrated in this blog post, you can easily introduce delays in your code and add cool effects to your website.
Now that you know how to implement a sleep function in TypeScript, go ahead and incorporate it into your own projects. Experiment, have fun, and let us know what creative uses you discover! 😄
If you have any questions or face any difficulties, feel free to leave a comment below. We're here to help! 🙌
Happy coding! 👨💻🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
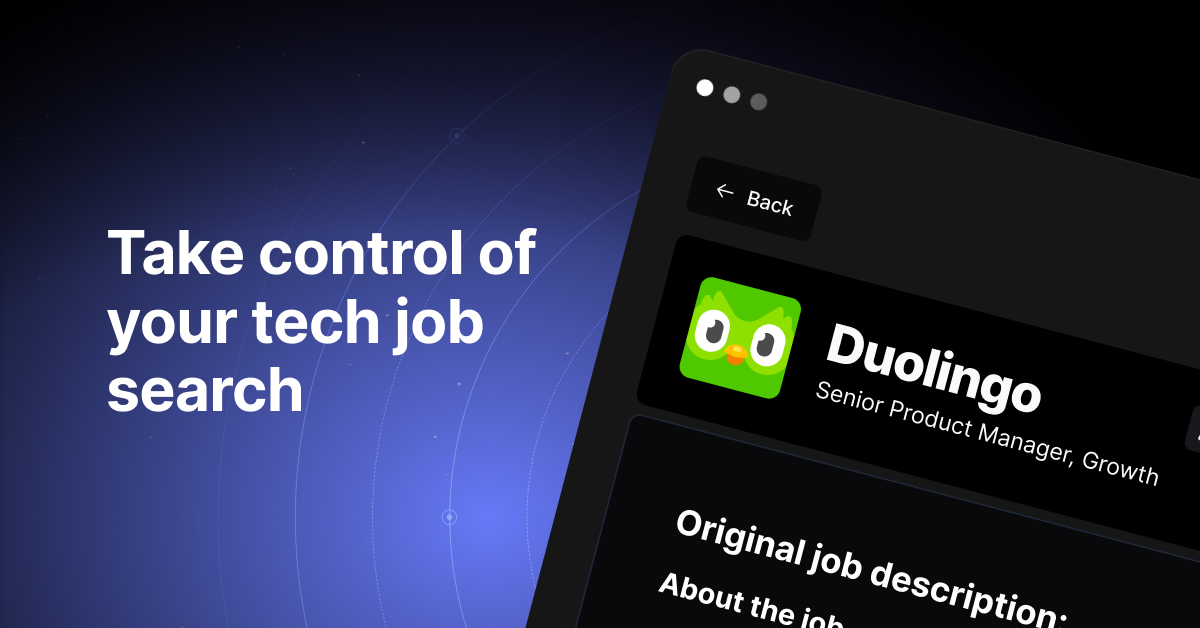