File Upload In Angular?
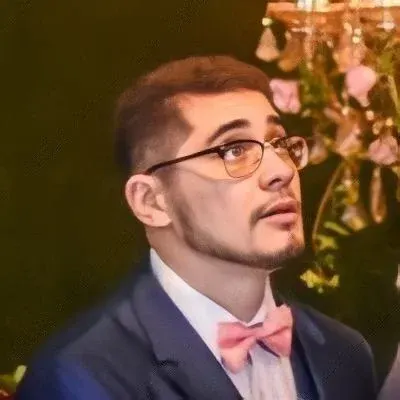
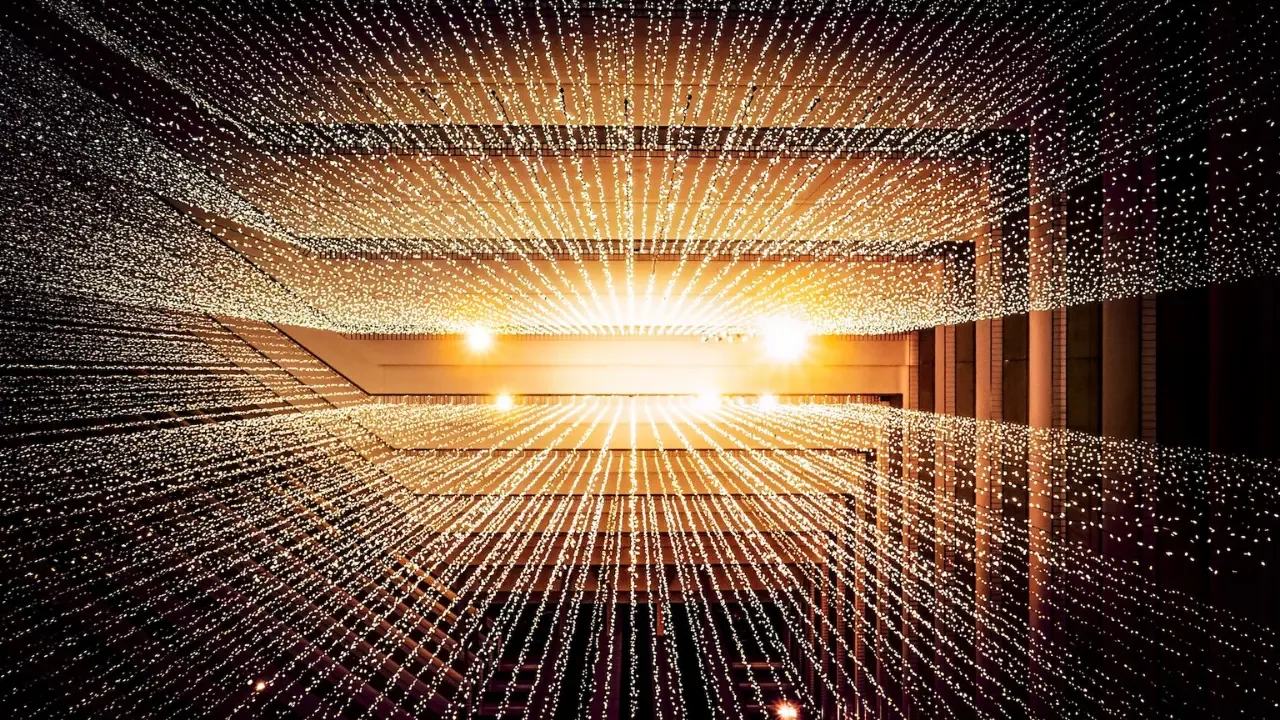
File Upload In Angular? Here's How to Do It! 📁📩
Are you struggling to upload a file in Angular 2? Don't worry, you're not alone! Many developers face this challenge when trying to implement file uploads in their applications. But fear not, because I'm here to guide you through the process and provide easy solutions to common issues. Let's get started! 😊
Common Issues and Frustrations 😩
Before diving into the solutions, let's address some common issues and frustrations you might encounter when trying to upload files in Angular.
Lack of clear documentation: It's frustrating when the available documentation on file uploads in Angular is scarce or unclear. This can make it challenging to understand the necessary steps and implementation details.
Compatibility problems: Some file upload libraries or approaches might not be compatible with Angular 2 or newer versions. This can lead to errors and difficulties in integrating them into your project.
Missing sample code or demos: Having access to sample code or demos makes it much easier to understand the implementation and see the file upload feature in action. Without it, developers have to rely on trial and error, wasting valuable time.
Now that we've identified these issues, let's explore some straightforward solutions to help you overcome these challenges. 💪
Easy Solutions and Tips 🛠️✨
Angular File Upload library: One popular and reliable library for file uploads in Angular is ng2-file-upload. It provides a simple API and comprehensive documentation, making it easier for you to understand and implement file uploads in your application. Here's a basic example of how to use it:
import { FileUploader } from 'ng2-file-upload';
// Create a new instance of the FileUploader
const uploader = new FileUploader({ url: '/api/upload' });
// Listen to events
uploader.onCompleteItem = (item, response, status, headers) => {
console.log('File uploaded successfully');
};
// Add files to the uploader queue
uploader.addToQueue(file);
ng2-uploader: Another fantastic library for file uploads in Angular is ng2-uploader. It offers various features and customization options. Here's a basic code snippet to get you started:
import { Component } from '@angular/core';
import { UploadFile, UploadInput, UploadOutput } from 'ng2-uploader';
@Component({
selector: 'app-upload',
template: `
<input type="file" (change)="uploadFile($event.target.files)">
`
})
export class UploadComponent {
uploadFile(fileList: FileList) {
const file: UploadFile = {
name: fileList[0].name,
file: fileList[0],
};
const uploadInput: UploadInput = {
type: 'uploadAll',
url: '/api/upload',
headers: { 'Authorization': 'Bearer YourAuthToken' },
data: { additionalData: 'Additional Data' },
method: 'POST',
file: file
};
this.uploadOutput.emit(uploadInput);
}
}
Remember to install these libraries using your favorite package manager, such as npm or yarn, before using them in your project.
A Compelling Call-to-Action: Share Your Experience! 📢⚡
Have you successfully uploaded a file in Angular? We want to hear from you! Share your preferred method, code snippets, and any additional tips or tricks you've discovered. Help your fellow developers overcome their file upload challenges by leaving a comment below. Let's build a supportive community together! 🤝✨
That's all for now, folks! I hope this guide helps you with your file upload endeavors in Angular. Remember, if you encounter any issues, don't hesitate to reach out for assistance. Happy coding! 💻💡
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
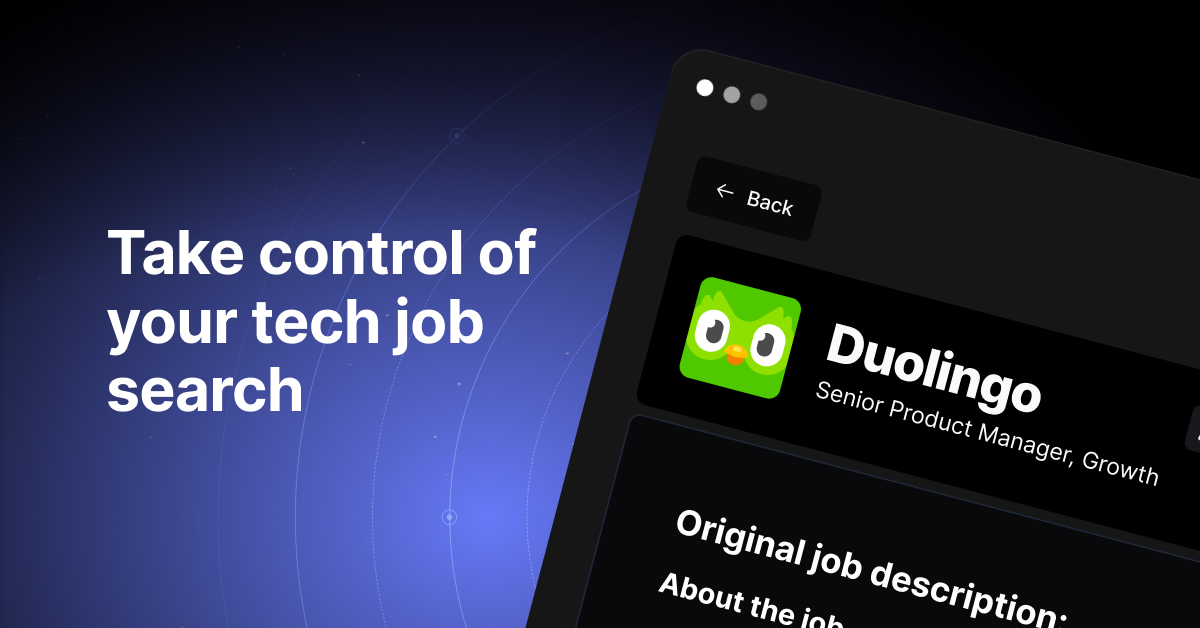