Can"t bind to "formGroup" since it isn"t a known property of "form"
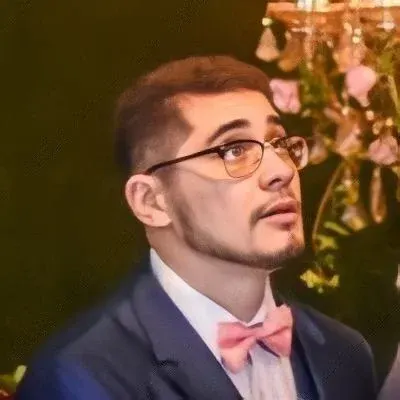

🚀 Angular FormGroup Error: Can't bind to 'formGroup' since it isn't a known property of 'form' 🚀
The Situation
So you're trying to create a simple form in your Angular application, but no matter what you do, it never works. 😩
The Angular Version
Angular 2.0.0 RC5 🎯
The Error
You encounter the following error message:
Can't bind to 'formGroup' since it isn't a known property of 'form'
The Code
Let's take a look at the view, controller, and ngModule code that you provided:
The View
<form [formGroup]="newTaskForm" (submit)="createNewTask()">
<div class="form-group">
<label for="name">Name</label>
<input type="text" name="name" required>
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
The Controller
import { Component } from '@angular/core';
import { FormGroup, FormControl, Validators, FormBuilder } from '@angular/forms';
@Component({
selector: 'task-add',
templateUrl: 'app/task-add.component.html'
})
export class TaskAddComponent {
newTaskForm: FormGroup;
constructor(fb: FormBuilder)
{
this.newTaskForm = fb.group({
name: ["", Validators.required]
});
}
createNewTask()
{
console.log(this.newTaskForm.value)
}
}
The ngModule
import { NgModule } from '@angular/core';
import { BrowserModule } from '@angular/platform-browser';
import { FormsModule, ReactiveFormsModule } from '@angular/forms';
import { routing } from './app.routing';
import { AppComponent } from './app.component';
import { TaskService } from './task.service'
@NgModule({
imports: [
BrowserModule,
routing,
FormsModule,
ReactiveFormsModule
],
declarations: [ AppComponent ],
providers: [
TaskService
],
bootstrap: [ AppComponent ]
})
export class AppModule { }
The Problem
The error is happening because the ReactiveFormsModule
is not imported in your AppModule
. The formGroup
directive is part of Angular's ReactiveFormsModule
and not the FormsModule
.
The Solution
To fix the error, follow these steps:
In your
AppModule
, importReactiveFormsModule
from@angular/forms
:import { ReactiveFormsModule } from '@angular/forms';
Add
ReactiveFormsModule
to theimports
array in@NgModule
:imports: [ BrowserModule, routing, FormsModule, ReactiveFormsModule ],
Save the changes and run your application again. 🏃
The Explanation
The ReactiveFormsModule
provides Angular with reactive forms capabilities, which include the formGroup
directive. By importing and including ReactiveFormsModule
in your AppModule
, you make those capabilities available in your application.
The Call-to-Action
Now that you have resolved the issue, it's time to celebrate and get back to coding! 🎉 If you found this guide helpful, don't be shy and share it with your fellow Angular developers. Let's spread the knowledge and help others tackle similar issues.
Feel free to leave a comment sharing your experiences or any other Angular topics you'd like to learn more about!
Keep coding, keep exploring! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
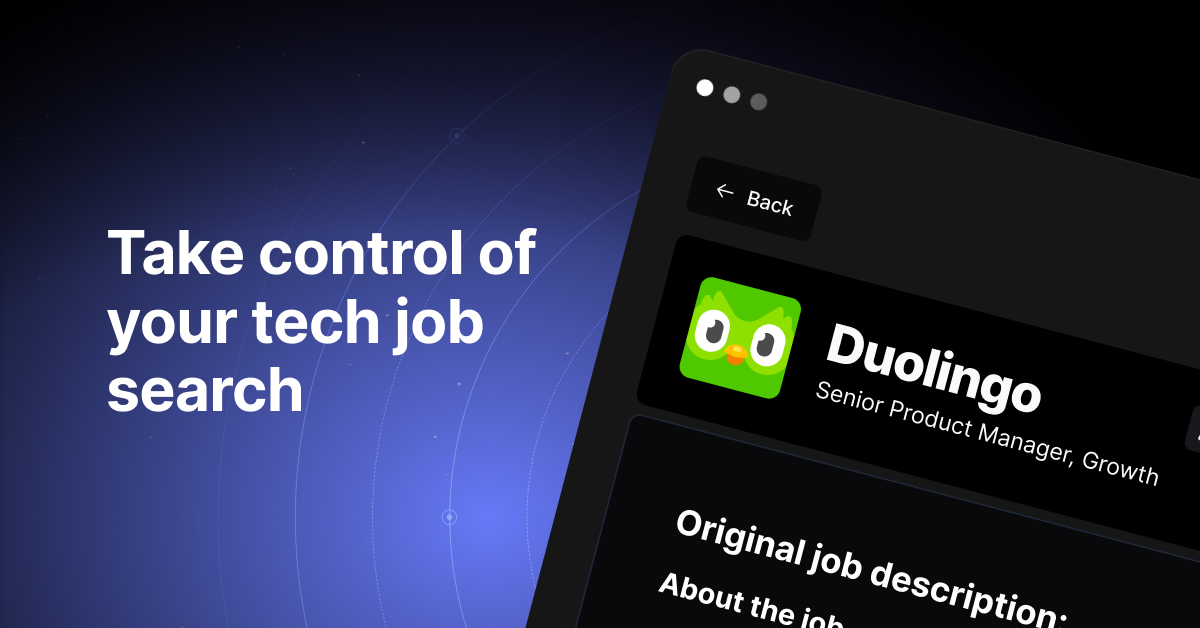