What is the correct way to add date picker in flutter app?
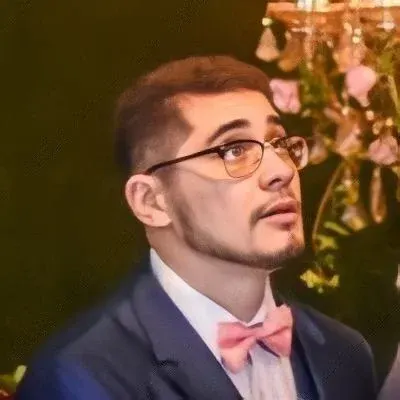
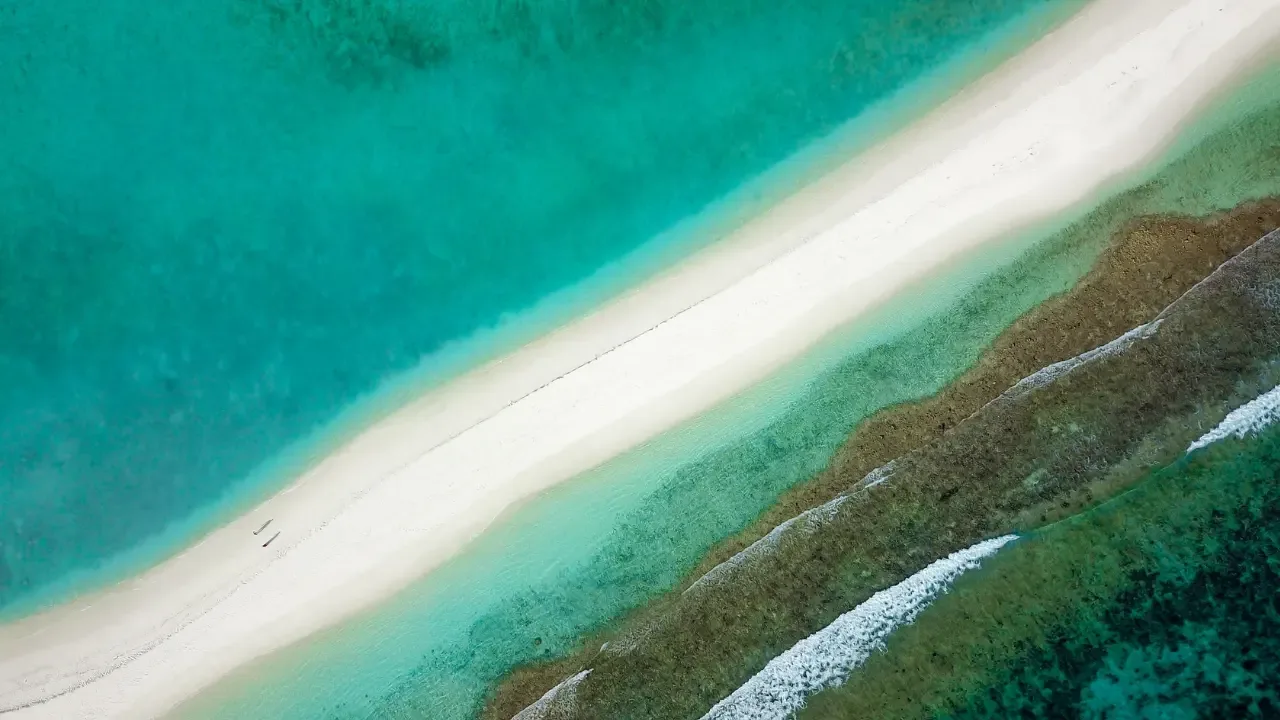
The Easy Way to Add a Date Picker in Your Flutter App 📅
So, you're working on your Flutter app and you've hit a roadblock. You want to add a date picker in your signup page for the user's date of birth (DOB). But fear not! I'm here to guide you through the process and help you find the correct way to achieve this ✨.
The Problem 💥
The common issue faced by users like you is not being able to find the correct way to add a date picker in their Flutter app. It can be frustrating when you're new to Flutter and still navigating your way around the framework.
The Solution 🔧
Fortunately, Flutter provides us with a simple and efficient way to add a date picker to our app. The first step is to add the flutter_datetime_picker
package to your pubspec.yaml
file. Here's how you can do it:
dependencies:
flutter:
sdk: flutter
flutter_datetime_picker: ^1.5.1
Once you've added the package, run flutter pub get
to fetch the dependencies.
Now, let's integrate the date picker in your signup page. First, import the necessary classes like this:
import 'package:flutter_datetime_picker/flutter_datetime_picker.dart';
Next, add a button or any other widget that will trigger the date picker to show up when tapped. For example, you could use a RaisedButton
as follows:
RaisedButton(
onPressed: () {
DatePicker.showDatePicker(
context,
showTitleActions: true,
onChanged: (date) {
// Do something with the chosen date
},
onConfirm: (date) {
// Do something with the confirmed date
},
currentTime: DateTime.now(),
locale: LocaleType.en,
);
},
child: Text('Select Date'),
),
When the user taps the "Select Date" button, the DatePicker.showDatePicker()
method will be called. This will display the date picker and allow the user to choose a date.
The showTitleActions
parameter is set to true
to show the "Cancel" and "Confirm" buttons at the top of the date picker for a better user experience.
The onChanged
callback function is called whenever the user selects a different date.
The onConfirm
callback function is called when the user confirms the chosen date.
The currentTime
parameter is set to DateTime.now()
in this example, which sets the initial date to the current date.
The locale
parameter is set to LocaleType.en
, which sets the date picker's locale to English.
Once the user selects or confirms a date, you can perform any necessary actions with the chosen date inside the respective callback functions.
Call-to-Action: Share your experience! 💬
Now that you know the correct way to add a date picker in your Flutter app, it's your turn to put it into practice! Try implementing it in your signup page and let me know how it goes. Did you encounter any issues? Do you have any other Flutter questions or problems?
Leave a comment below and share your experience with the Flutter community. Together, we can help each other overcome any coding challenges and create amazing apps! 😄🚀
Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
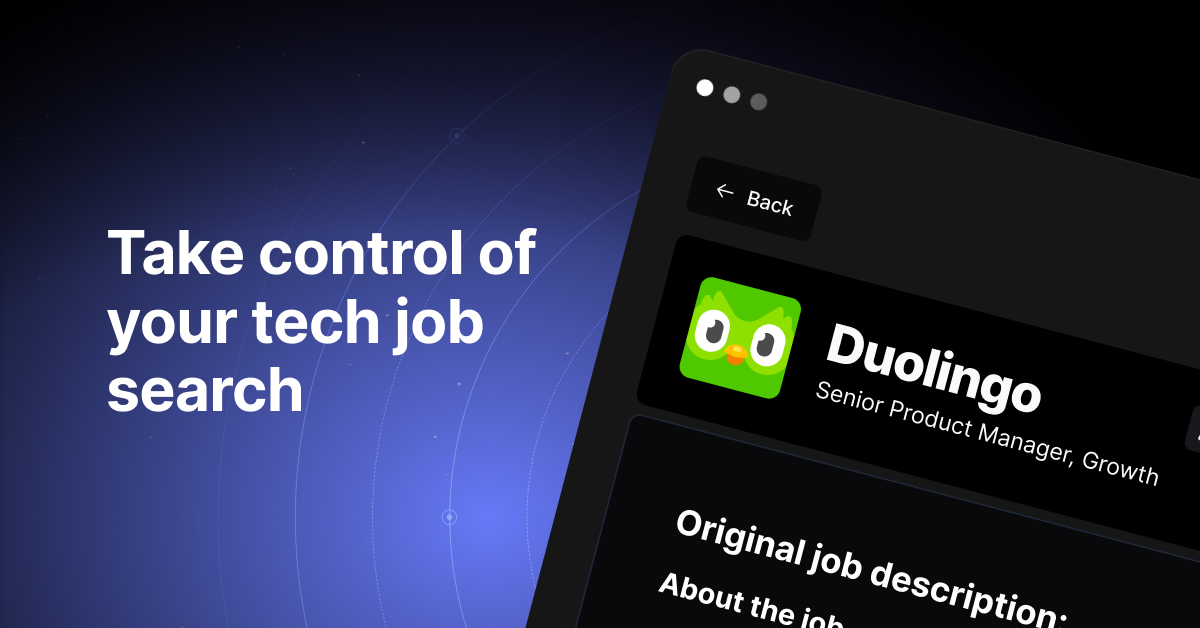