How to set RecyclerView app:layoutManager="" from XML?
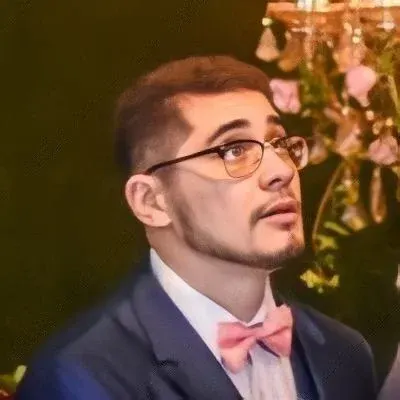
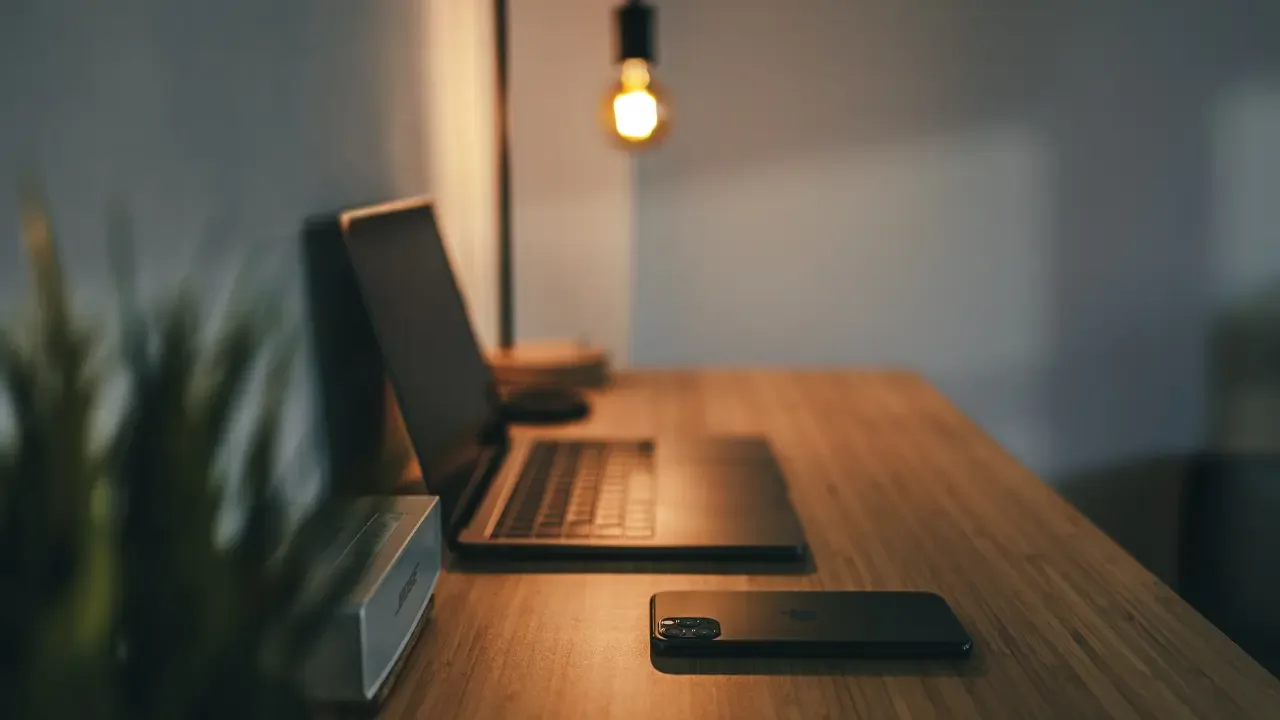
📝 How to set RecyclerView app:layoutManager="" from XML?
If you've ever worked with RecyclerView in Android, you know that setting its layoutManager is crucial for controlling how your data is displayed. However, figuring out how to set it from XML can be a bit tricky. But worry not, my friend! I've got your back. 👊
The Challenge
Take a look at the code snippet below:
<android.support.v7.widget.RecyclerView
app:layoutManager="???"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
You see that layoutManager="???"
bit? That's where the magic should happen. But how do we actually set the layoutManager from XML when there's no direct attribute for it? 🤔
The Solution
To set the RecyclerView's layoutManager from XML, we need to make use of custom attributes and a bit of code in our Java/Kotlin classes. Here's how to do it step by step:
Define a custom attribute in your
res/values/attrs.xml
file. If the file doesn't exist, create it. Add the following code:<resources> <declare-styleable name="CustomRecyclerView"> <attr name="customLayoutManager" format="enum"> <enum name="linear" value="0"/> <enum name="grid" value="1"/> <!-- Add more layoutManager options if needed --> </attr> </declare-styleable> </resources>
In your layout XML file, replace
app:layoutManager="???"
with your custom attribute:<com.example.yourpackage.CustomRecyclerView app:customLayoutManager="linear" android:layout_width="match_parent" android:layout_height="match_parent"/>
Create a new class file called
CustomRecyclerView
and make it extendRecyclerView
. In this class, add the following code:public class CustomRecyclerView extends RecyclerView { public CustomRecyclerView(Context context, AttributeSet attrs) { super(context, attrs); init(context, attrs); } private void init(Context context, AttributeSet attrs) { TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.CustomRecyclerView); int layoutManagerValue = typedArray.getInt(R.styleable.CustomRecyclerView_customLayoutManager, 0); typedArray.recycle(); if (layoutManagerValue == 0) { setLayoutManager(new LinearLayoutManager(context)); } else if (layoutManagerValue == 1) { setLayoutManager(new GridLayoutManager(context, 2)); } // Add more layoutManager options if needed } }
That's it! You're all set. Now, when you use your
CustomRecyclerView
in your layouts and setapp:customLayoutManager
to either "linear" or "grid", the corresponding layoutManager will be applied.
Conclusion
Setting the RecyclerView's layoutManager from XML might seem daunting at first, but with custom attributes and a little bit of code, it becomes a piece of cake. 🍰
I hope this guide helped you overcome the challenge and saved you some time and headaches. Now go ahead and rock your RecyclerViews like a boss! 💪
Your Turn
Have you ever faced any issues with RecyclerView's layoutManager? How did you solve them? Share your experiences in the comments below and let's help each other out! 😊
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
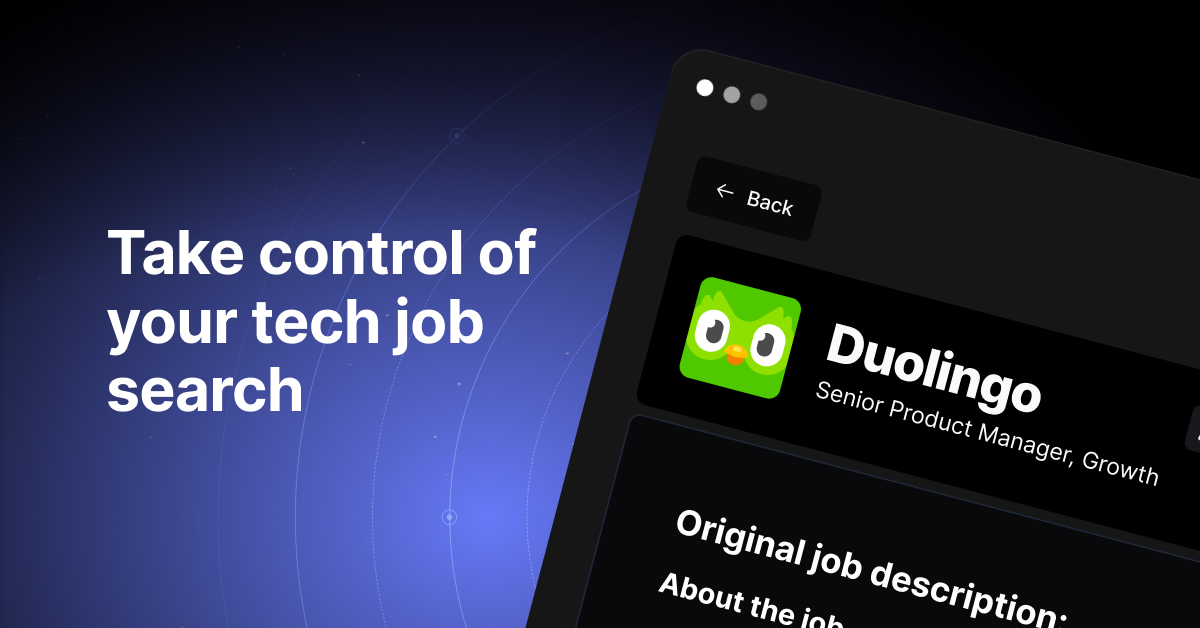