How to load an ImageView by URL in Android?
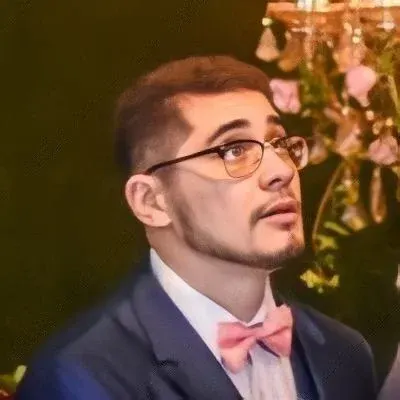
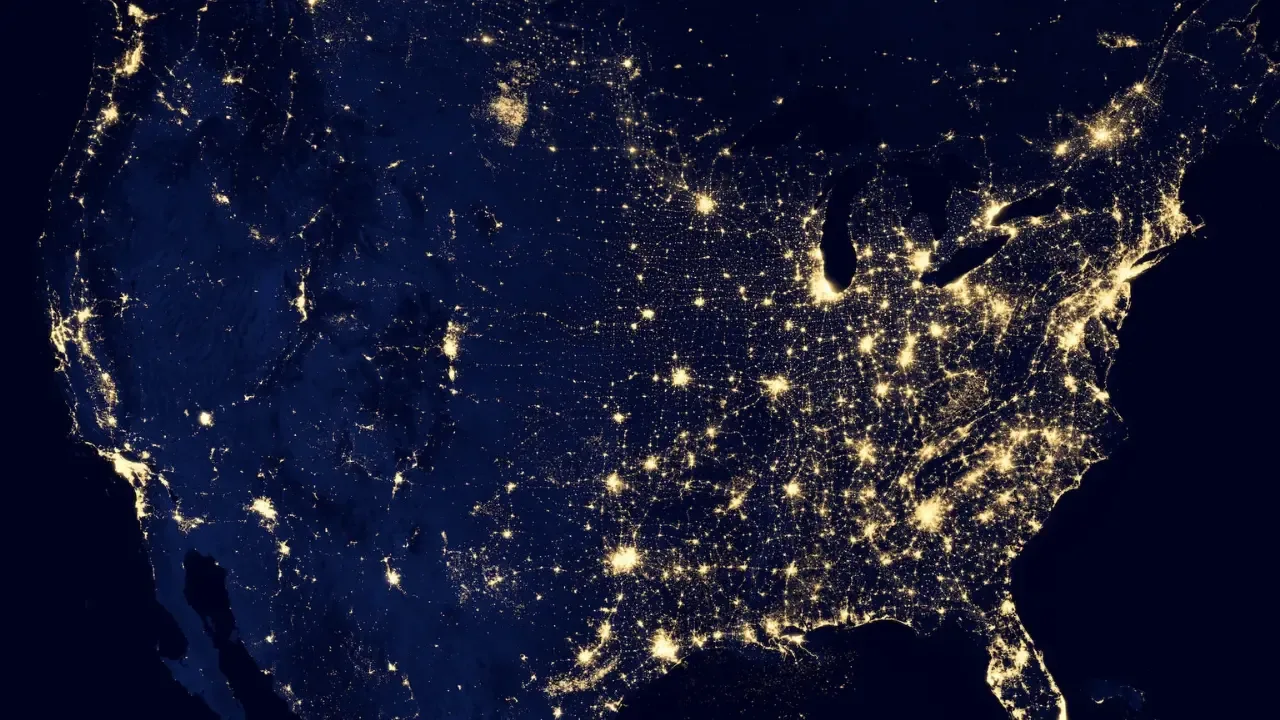
š·š» How to Load an ImageView by URL in Android? šš²
So, you want to display an image from a URL in your ImageView on Android? š¤ No worries, I got you covered! š
ā ļø Common Issues & The Problem ā ļø
The most common issue people face when trying to load an image from a URL into an ImageView is the dreaded NetworkOnMainThreadException. š¬ This exception occurs when you try to perform a network operation on the main UI thread, which is a big no-no.
š” Easy Solutions š”
1ļøā£ Using an External Library: One of the easiest ways to handle image loading from a URL is by using external libraries. Picasso and Glide are two popular options that make the process a breeze.
Here's an example using Picasso:
Picasso.get().load("your_image_url").into(imageView);
And here's an example using Glide:
Glide.with(context).load("your_image_url").into(imageView);
2ļøā£ Using AsyncTask: Another option to avoid the NetworkOnMainThreadException is by using AsyncTask. This will help you perform the network operation on a background thread.
Here's an example:
class ImageLoaderTask extends AsyncTask<String, Void, Bitmap> {
protected Bitmap doInBackground(String... urls) {
String imageUrl = urls[0];
Bitmap bitmap = null;
try {
InputStream in = new java.net.URL(imageUrl).openStream();
bitmap = BitmapFactory.decodeStream(in);
} catch (Exception e) {
Log.e("ImageLoadTask", e.getMessage());
e.printStackTrace();
}
return bitmap;
}
protected void onPostExecute(Bitmap result) {
imageView.setImageBitmap(result);
}
}
To load an image from a URL using AsyncTask, call the execute
method and pass the image URL:
new ImageLoaderTask().execute("your_image_url");
⨠Compelling Call-to-Action āØ
Now that you know how to load an ImageView by URL in Android, go ahead and implement it in your own app! š Whether you choose to use an external library like Picasso or Glide, or if AsyncTask feels more comfortable to you, just remember to avoid performing network operations on the main UI thread.
Remember, sharing is caring! If you found this guide helpful, make sure to share it with your fellow Android developers. And if you have any questions or other topics you'd like me to cover, leave a comment below! š
Happy coding! š»š„
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
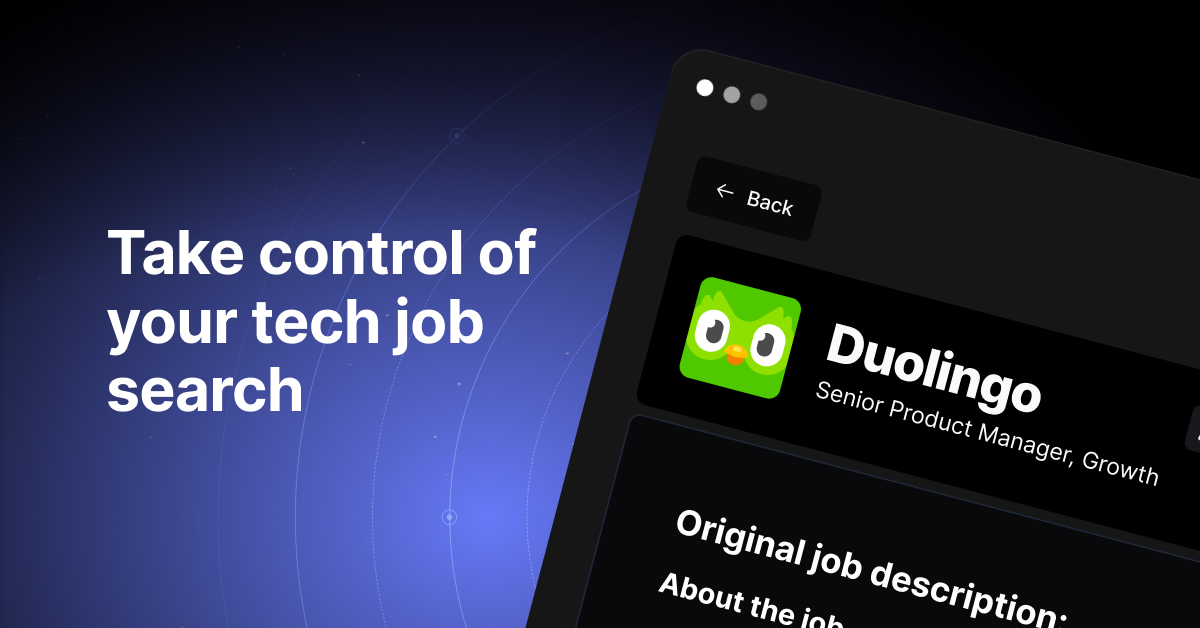