How to add dividers and spaces between items in RecyclerView
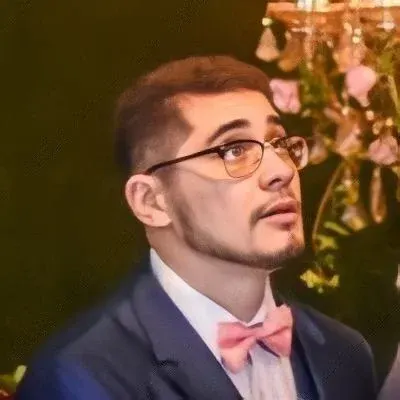
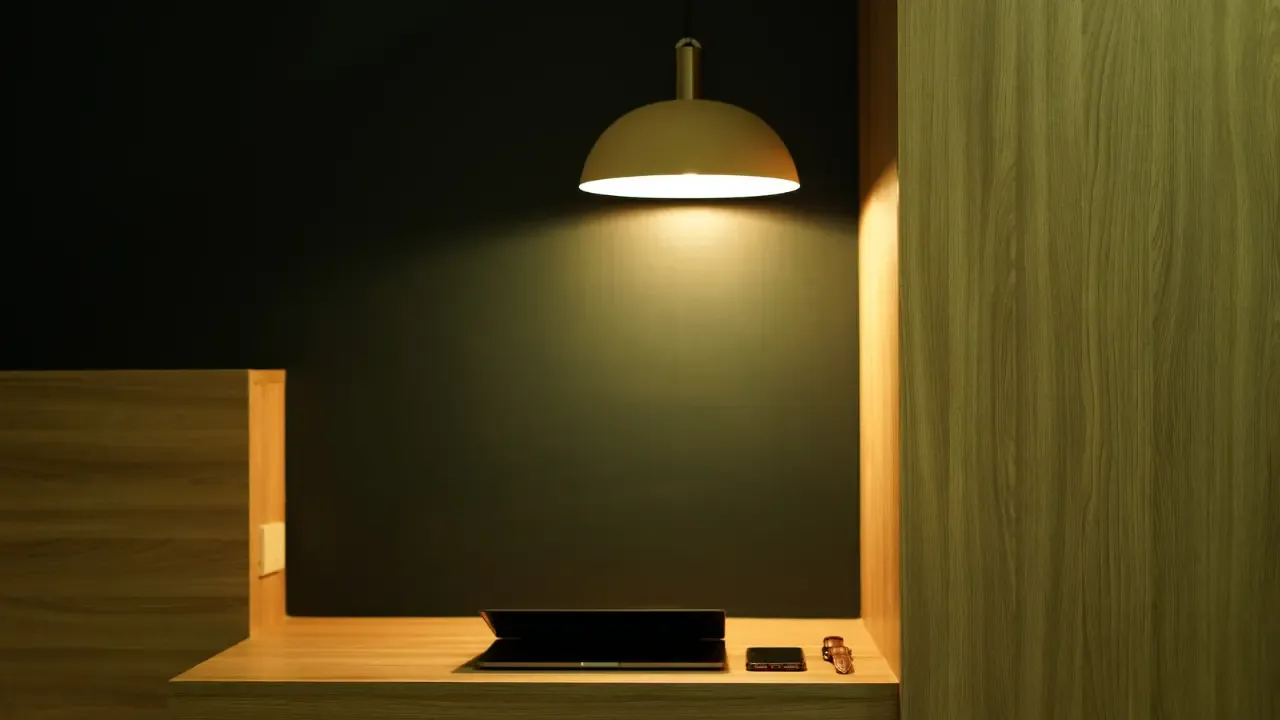
How to Add Dividers and Spaces Between Items in RecyclerView
You've probably encountered this problem before: you have a list of items in a RecyclerView, but there's no easy way to add dividers or spaces between them. In the past, you could easily accomplish this with the ListView class, using the "divider" and "dividerHeight" parameters. But what about RecyclerView? 🤔
The Challenge
Let's take a look at the code snippet below, which demonstrates how dividers were added in a ListView:
<ListView
android:id="@+id/activity_home_list_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:divider="@android:color/transparent"
android:dividerHeight="8dp"/>
Unfortunately, the RecyclerView class doesn't provide a similar option out of the box:
<android.support.v7.widget.RecyclerView
android:id="@+id/activity_home_recycler_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scrollbars="vertical"/>
So, how can we achieve the same result in RecyclerView? Let's find out! 😎
Solution 1: Define Margins
One way to add space between items is by defining margins directly in the list item's layout. For example, let's say we have a list item layout called "list_item.xml". We can modify it like this:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:layout_marginBottom="8dp">
<!-- Rest of the layout -->
</LinearLayout>
By adding top and bottom margins to the root layout of the list item, we create spacing between each item. This method gives you control over the amount of space you want to add.
Solution 2: Add a Custom Divider View
If you prefer using dividers instead of margins, you can add a custom divider view directly into the list item's layout. Here's how you can do it:
Create a new XML layout file called "divider.xml" that defines your custom divider. For example:
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@android:color/darker_gray"/>
In your list item layout ("list_item.xml"), add the custom divider view wherever you want it to appear. For example:
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<!-- Rest of the layout -->
<include layout="@layout/divider"/>
</LinearLayout>
By including the "divider.xml" layout within the list item layout, you achieve the desired divider effect.
The Best Approach
Both solutions mentioned above are valid, but which one is the best? 😕 Well, it depends on your specific use case.
Use margins if you want consistent spacing between items and don't need customized dividers.
Use custom divider views if you want more control over the appearance of dividers and need them to be different for each item.
Consider your design requirements and choose the solution that best fits your needs.
Your Turn
Now that you have the solutions to add dividers and spaces between items in RecyclerView, why not give it a try? 🚀 Experiment with different layouts, margins, and dividers to achieve the desired visual effect.
If you have any questions or feedback, feel free to leave a comment below! Let's make RecyclerView layouts look even better together! 💪💻
📢 Share Your Success
Have you successfully added dividers or spaces in your RecyclerView? We would love to hear about your experience! Share your success stories, code snippets, or screenshots with us on Twitter using the hashtag #RecyclerViewDividers.
Happy coding! ✨👩💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
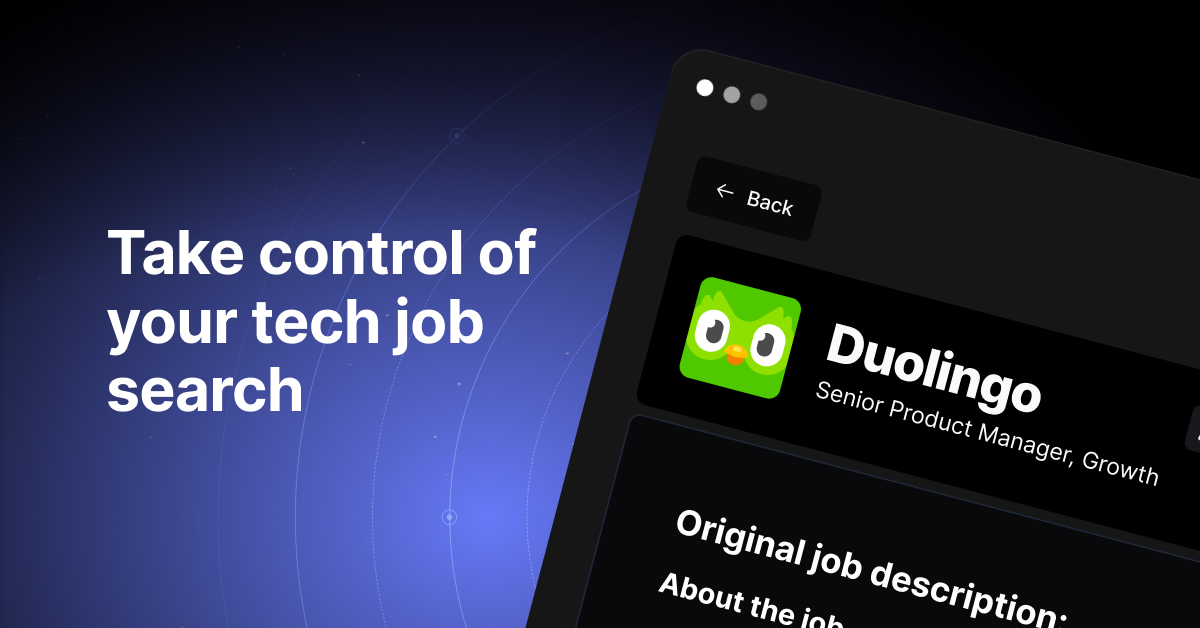