Change app language programmatically in Android
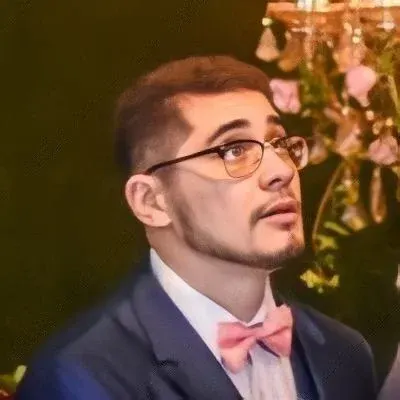
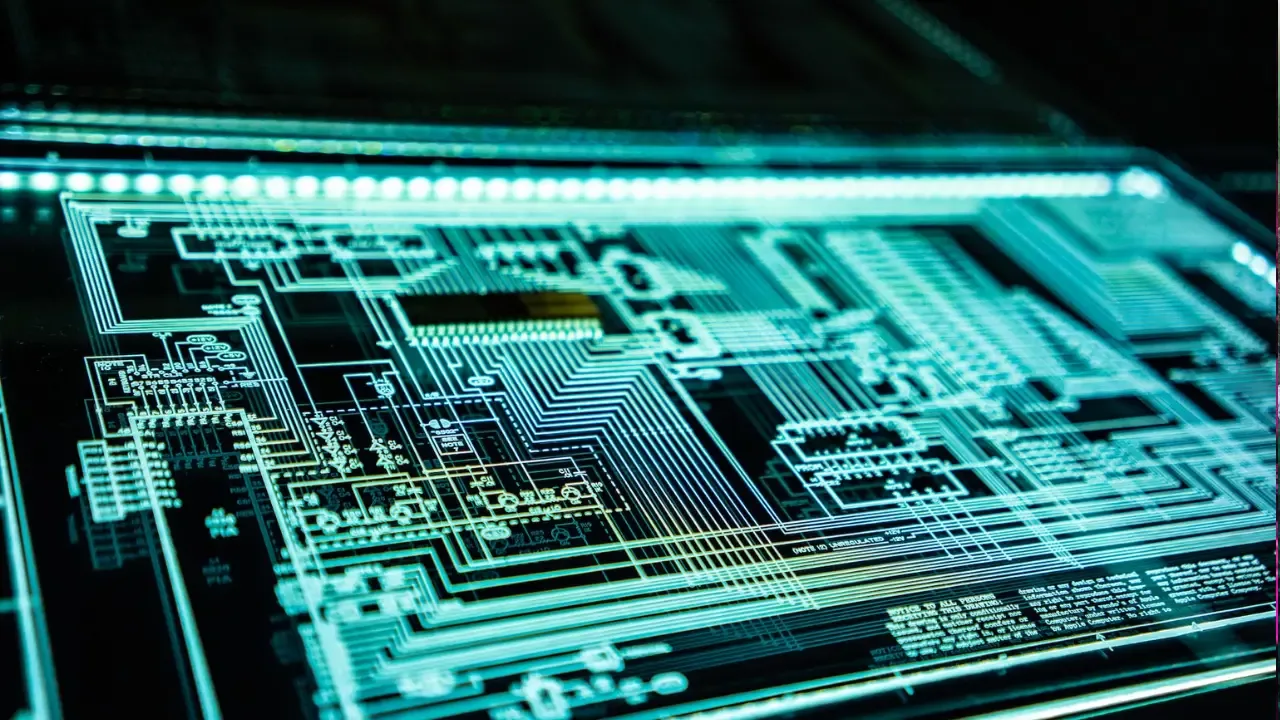
Change app language programmatically in Android: A Simple Guide ✨📱
Is it possible to change the language of an app programmatically while still using Android resources? Can we request a resource in a specific language? If you've ever wondered how to let the user change the language of the app from the app itself, you're in luck! In this blog post, we'll walk you through the process and provide easy solutions to these common issues. Let's dive in! 💪🚀
The Challenge 🤔
Changing the language of an app dynamically can be tricky, especially when trying to maintain the use of Android resources. However, it is possible to achieve this without reinventing the wheel. Let's explore some methods and best practices to tackle this challenge head-on!
Method 1: Configuration Overrides 🌍
To change the app's language programmatically, you can use Configuration
overrides provided by the Android framework. By updating the Locale
property of the app's configuration, you can achieve the desired language change.
Locale locale = new Locale("en"); // Specify the desired language code
Locale.setDefault(locale);
Configuration config = new Configuration();
config.setLocale(locale);
Context context = getApplicationContext();
context.getResources().updateConfiguration(config, context.getResources().getDisplayMetrics());
In the above example, we set the locale to English ("en") and update the app's configuration accordingly. This will cause Android resources to be fetched in the specified language.
Method 2: Custom Resource Handling 🌐
If you need more fine-grained control over your app's resources, you can create custom methods to load resources in a specific language. This approach allows you to bypass the configuration changes and fetch resources based on your implementation.
public static String getResourceString(Context context, String key, Locale locale) {
Configuration config = new Configuration(context.getResources().getConfiguration());
config.setLocale(locale);
Context localizedContext = context.createConfigurationContext(config);
return localizedContext.getResources().getString(context.getResources().getIdentifier(key, "string", context.getPackageName()));
}
With the above method, you can pass the desired language locale and fetch the appropriate resource string using the getResourceString
method. This way, you can have full control over your app's localization.
Here's the key takeaway:
You now have two methods to change the language of your Android app programmatically. The first method utilizes Configuration
overrides, providing a quick and easy way to update the app's language using standard Android resources. On the other hand, the second method allows for more flexibility by handling custom resource loading.
Implementing these solutions grants your users the power to explore your app in their preferred language, enhancing their experience and making your app more accessible. So go ahead and empower your users! 🌟
Have you faced any language-related challenges while developing an Android app? Share your experience in the comments below! We would love to hear from you and help you overcome any hurdles.
Keep coding, and until next time! 👩💻👨💻
Do you want more tips and tricks for Android app development? Stay tuned for our next post and subscribe to our newsletter to never miss an update! Subscribe now! 💌
Note: Don't forget to adapt the code snippets to fit your specific use case and error handling requirements.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
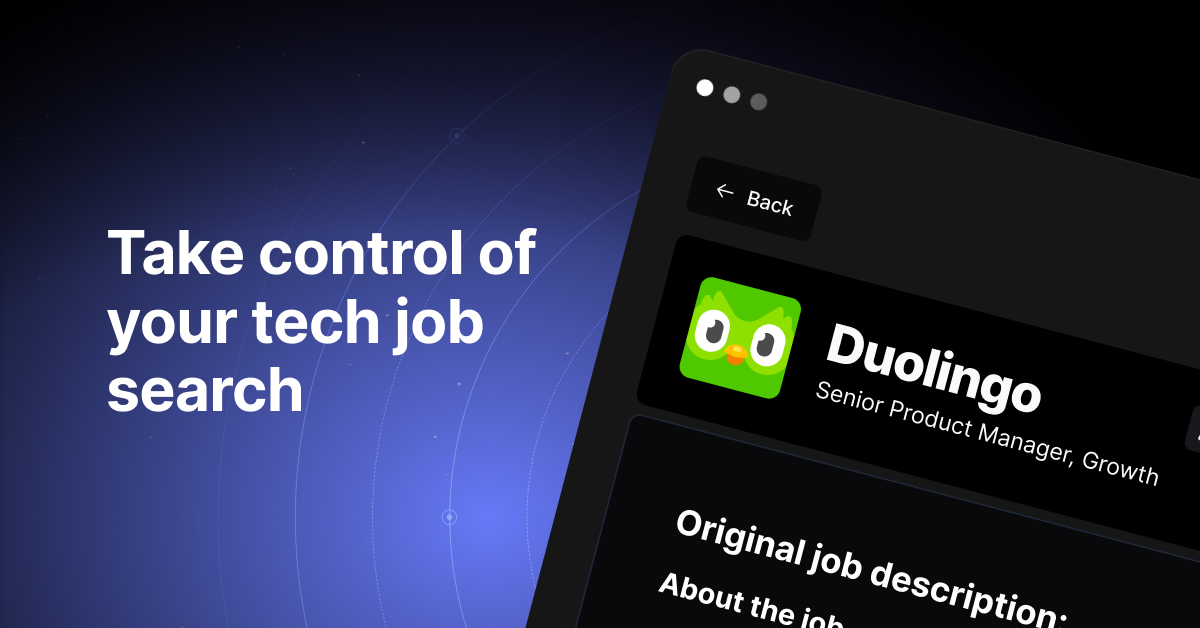