Android: How do I get string from resources using its name?
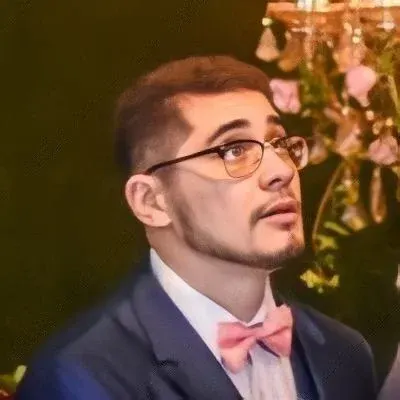
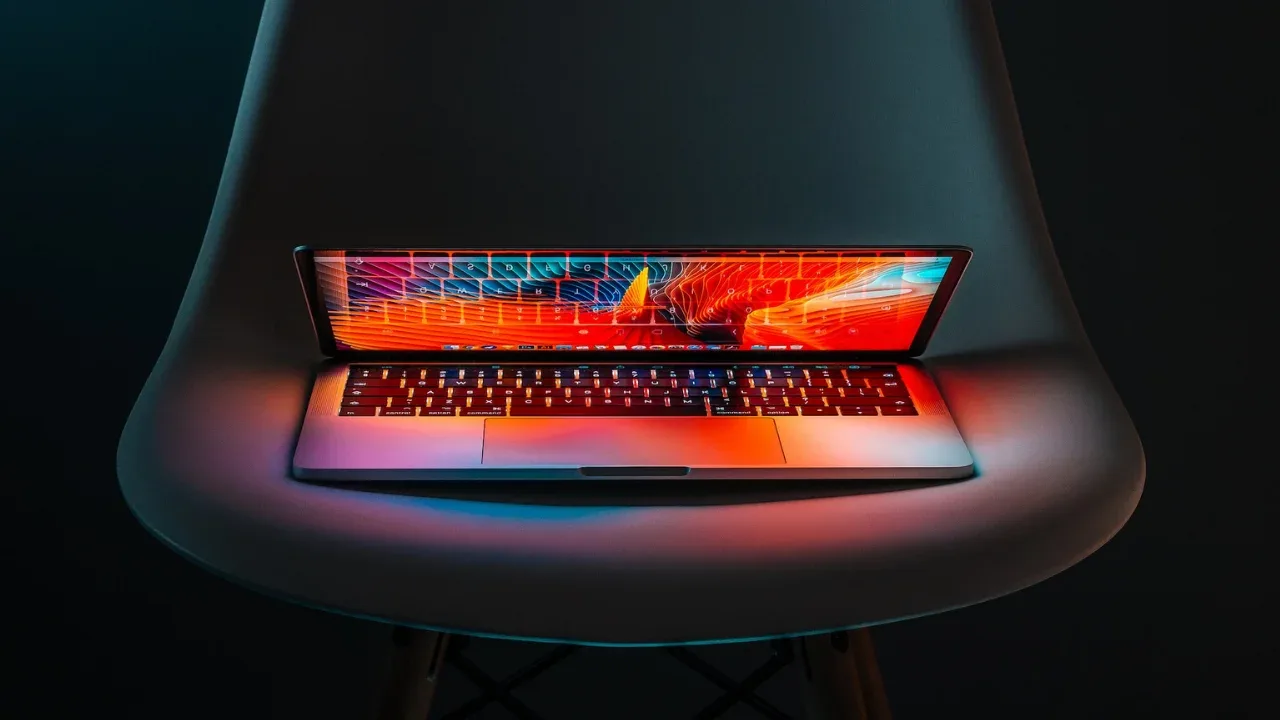
Android: How to Get a String from Resources Using Its Name
If you are developing an Android app that requires multiple languages for the user interface, you may encounter the need to retrieve string values from resources dynamically. In this blog post, we will address a common issue where the dynamic retrieval of string values fails and provide an easy solution to fix it.
The Problem
Let's say you have a resource file named strings.xml
located in the res/values
directory of your Android project. In this file, you have defined string values for multiple languages as follows:
<string name="tab_Books_en">Books</string>
<string name="tab_Quotes_en">Quotes</string>
<string name="tab_Questions_en">Questions</string>
<string name="tab_Notes_en">Notes</string>
<string name="tab_Bookmarks_en">Bookmarks</string>
<string name="tab_Books_ru">Книги</string>
<string name="tab_Quotes_ru">Цитаты</string>
<string name="tab_Questions_ru">Вопросы</string>
<string name="tab_Notes_ru">Заметки</string>
<string name="tab_Bookmarks_ru">Закладки</string>
Now, you want to retrieve these string values dynamically in your app using their names. You may try the following code snippet:
spec.setContent(R.id.tabPage1);
String pack = getPackageName();
String id = "tab_Books_" + Central.lang;
int i = Central.Res.getIdentifier(id, "string", pack);
String str = Central.Res.getString(i);
However, you encounter a problem where the value of i
is always 0, meaning that the string resource cannot be found.
The Solution
The issue in this case is the incorrect usage of the getIdentifier()
method. The getIdentifier()
method requires the full resource name, including the package and type information. In our case, we need to include the language suffix (_en
or _ru
) in the resource name.
To fix the problem, we need to modify the code as follows:
spec.setContent(R.id.tabPage1);
String pack = getPackageName();
String type = "string";
String id = pack + ":id/" + "tab_Books_" + Central.lang;
int i = Central.Res.getIdentifier(id, type, pack);
String str = Central.Res.getString(i);
By including the package name, type information (id/
), and the correct resource name with the language suffix, the getIdentifier()
method will be able to find the string resource successfully.
Conclusion
Retrieving string values from resources dynamically in Android can be tricky, especially when dealing with multiple languages. By following the easy solution provided in this blog post, you can avoid common issues like the one described. Remember to always provide the full resource name including the package and type information when using the getIdentifier()
method.
If you found this article helpful, please share it with your fellow Android developers. If you have any questions or further insights, feel free to leave a comment below. Happy coding!
📢 Call to Action
Did you face similar issues with your Android app's string resources? Don't worry, we've got you covered! Check out these other helpful guides on our blog to overcome common challenges in Android development:
"How to Localize Your Android App for Multiple Languages"
"Working with Android Resources: A Comprehensive Guide"
Remember to subscribe to our newsletter to stay updated with the latest tech tips and trends. Happy coding!
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
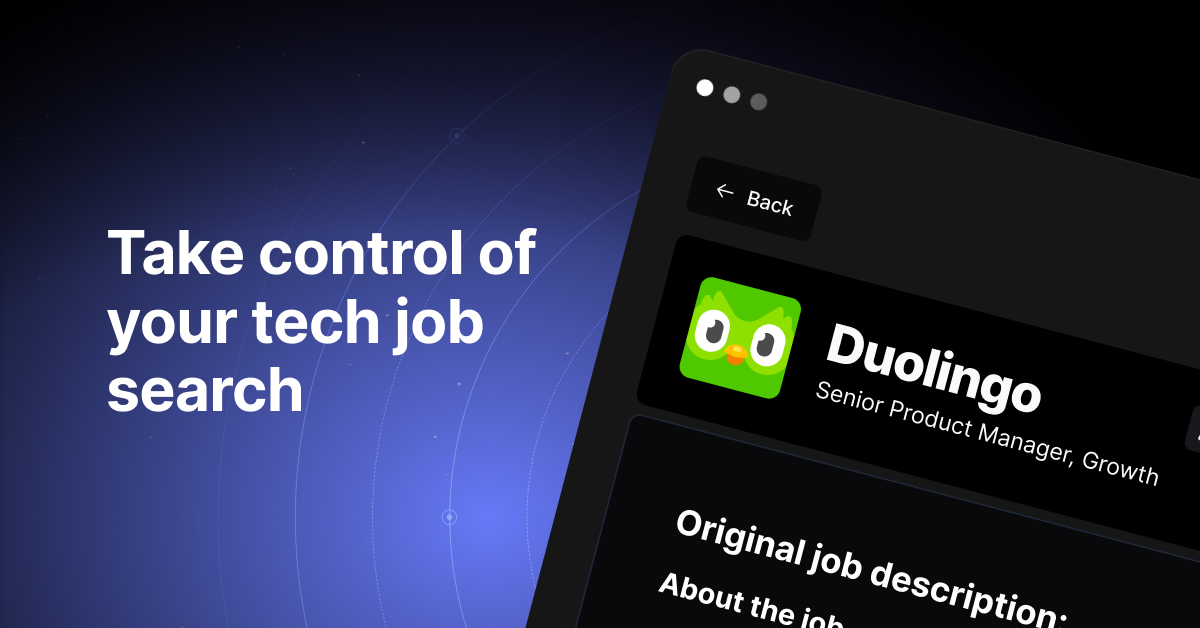