Android: combining text & image on a Button or ImageButton
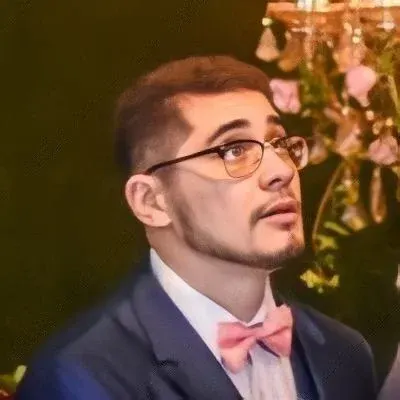
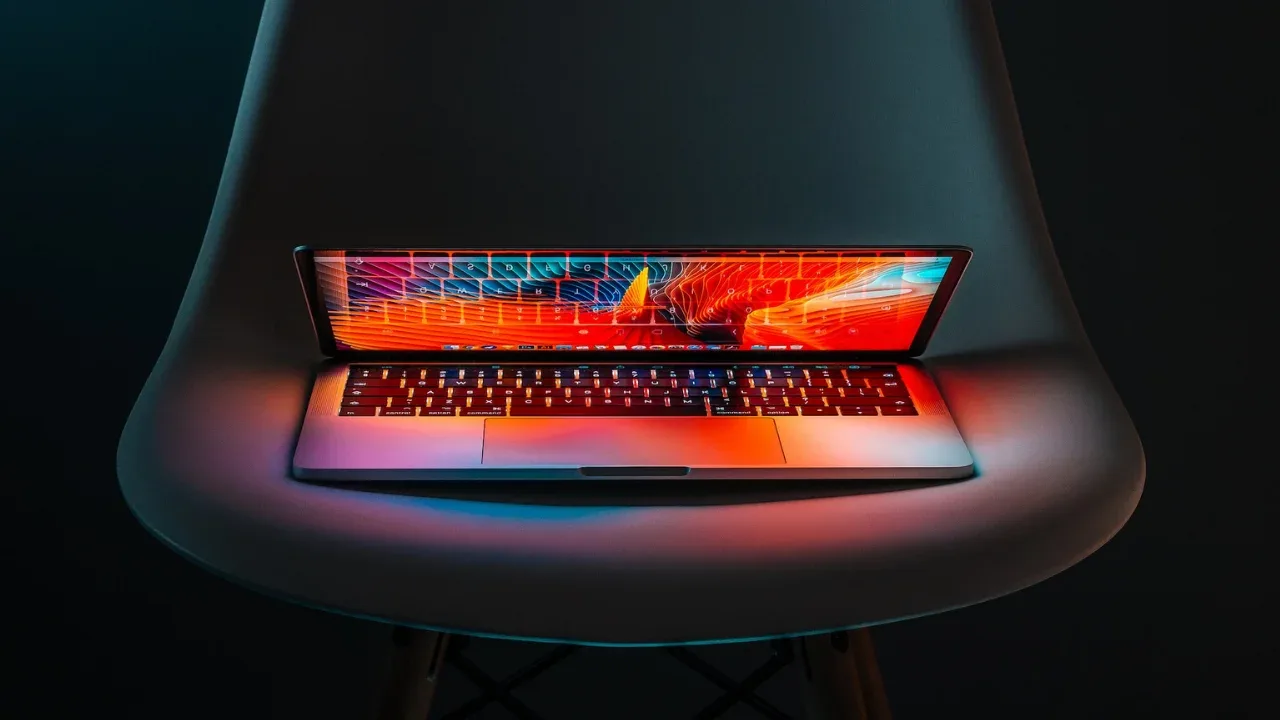
Combining Text & Image on an Android Button or ImageButton: Easy Solutions and Cool Tricks ๐๐ฑ๐จ
So, you want to spice up your Android app by combining a text and an image on a button or an image button? ๐ค Well, you've come to the right place! In this guide, we will address the common issues and provide easy and creative solutions to give your buttons a unique touch. Let's dive in! ๐ช
The Problem ๐
Let's start by understanding the problem. As stated by the user, they want to have an image as the background of a button and add dynamic text on top of the image. However, using the ImageButton
class doesn't allow adding text, and using the Button
class only enables defining an image with attributes like android:drawableBottom
. But this approach limits us to combining the text and image in the x- and y-dimensions, preventing us from placing the image below/under the text in the z-axis. ๐คทโโ๏ธ
Easy Solution - Layered Approach ๐ ๏ธ
One of the simplest solutions to achieve this effect is by using a layered approach with a combination of views. Here's how it works:
Wrap your image inside a
FrameLayout
. This will act as the container for our layered views.
<FrameLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<!-- Your image view here -->
<ImageView
android:id="@+id/image"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:src="@drawable/your_image" />
<!-- Your text view here -->
<TextView
android:id="@+id/text"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Your Text"
android:gravity="center"
android:textColor="@android:color/white" />
</FrameLayout>
Adjust the layout accordingly by setting
android:layout_width
andandroid:layout_height
attributes to eitherwrap_content
or specific dimensions, according to your requirements.
And voila! You have successfully layered your text on top of the image. You can further customize the appearance of both the image and the text, add padding, or even apply animations to make your button stand out! ๐ฅ
Taking it a Step Further - Custom Views ๐
If you're a developer willing to explore more advanced techniques, you can create your own custom view by extending either the Button
or ImageButton
class and overriding the onDraw()
method to achieve the desired effect. While this approach requires a deeper understanding of 2D rendering, it gives you unparalleled flexibility and control. Here's a high-level overview of the steps:
Create a new class that extends
Button
orImageButton
.
public class CustomButton extends Button {
// Constructor(s) if needed
@Override
protected void onDraw(Canvas canvas) {
// Implement your custom drawing logic here
super.onDraw(canvas);
}
}
Inside the
onDraw()
method, customize the drawing behavior to combine the text and the image using theCanvas
object.
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
Drawable drawable = getBackground();
// Retrieve and draw your image using drawable
// Retrieve and draw your text using getText()
// ... Implement the necessary positioning and sizing logic ...
}
Use your custom view in your layout XML by specifying the fully qualified name of your custom class.
<com.yourpackage.CustomButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
... />
Now your custom view will handle combining the text and image according to your specifications. ๐
Share Your Creativity! ๐ฃ
We hope this guide has helped you solve the issue of combining text and image on an Android button or image button. Whether you choose the layered approach or delve into the world of custom views, don't hesitate to show off your creations! Share your unique buttons on social media, tag us, and let the world appreciate your design skills. ๐
If you have any questions, suggestions, or more cool tricks to achieve this effect, feel free to leave a comment below. We're all ears! Happy coding! ๐๐ป
๐ฃ๐กโ๏ธ๐ ๏ธ๐ฑ๐ฅ๐จ๐ฃ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
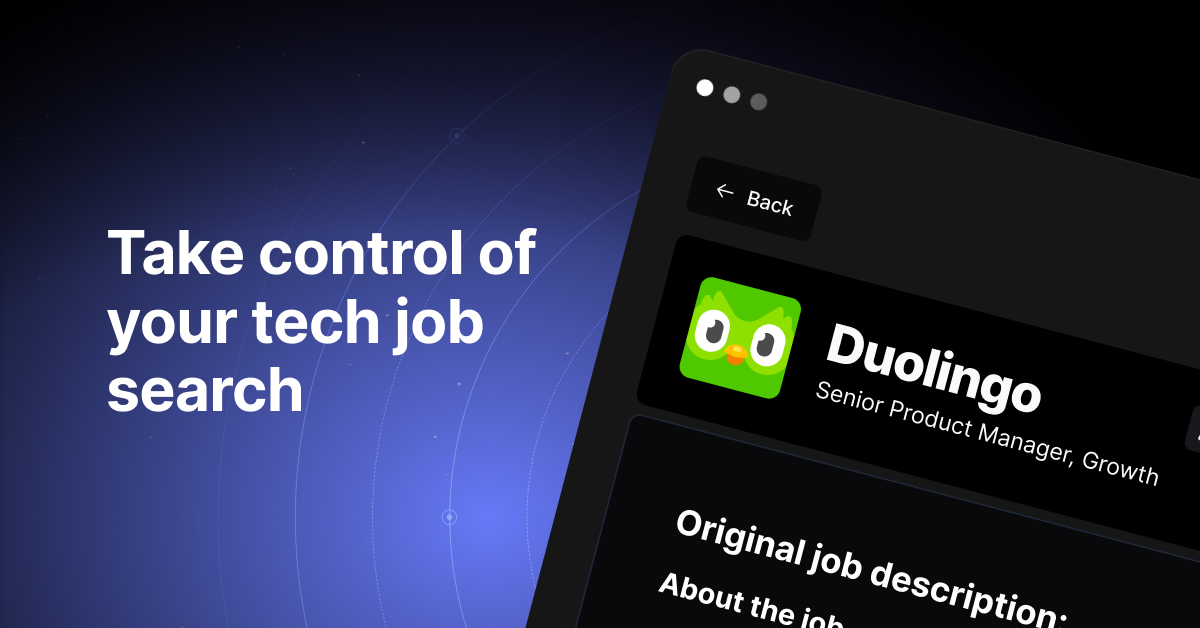