jQuery: Performing synchronous AJAX requests
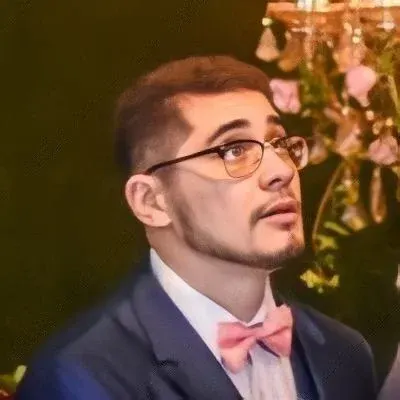
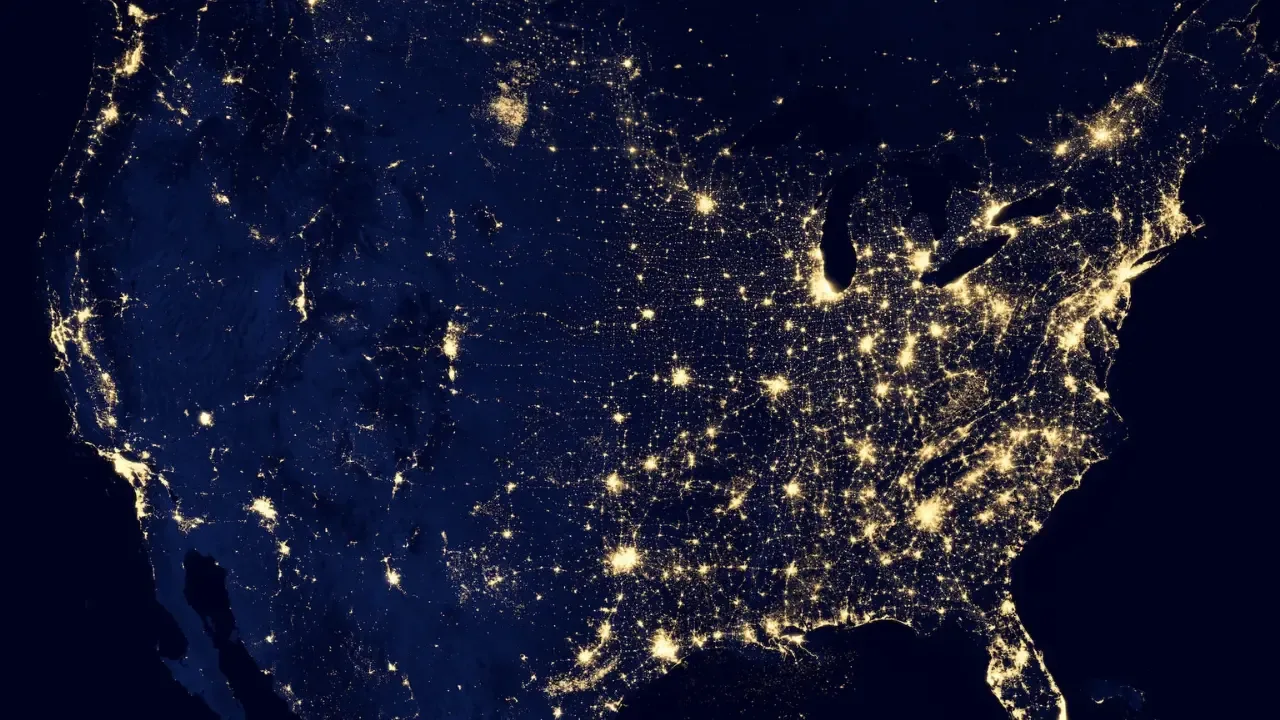
š Blog Post Title: "Synchronous AJAX Requests in jQuery: Solving the Mystery of Returning Properly"
Introduction: The Importance of Synchronous AJAX Requests
š Hey there tech enthusiasts! Are you all stuck on the elusive world of synchronous AJAX requests in jQuery? Look no further because we've got you covered! In this blog post, we're going to dive into the common issues faced when performing synchronous AJAX requests and provide you with easy solutions. So, let's get started and make those functions return properly! š
The Common Problem: Remote Page Loading, But No Return
š Picture this: you're using jQuery to perform a synchronous AJAX request, your remote page is loading successfully, but when you try to return the data, it's nowhere to be found! What's going on here? Don't worry, we've got your back! š
The Code Conundrum: Analyzing the getRemote() Function
To dig deeper into this issue, let's have a look at the getRemote() function:
function getRemote() {
var remote;
$.ajax({
type: "GET",
url: remote_url,
async: false,
success : function(data) {
remote = data;
}
});
return remote;
}
Unraveling the Mystery: The async: false and Return Values
š The culprit here lies in the async: false
option used in the AJAX request. When set to false
, it means the request is synchronous, causing the JavaScript execution to pause until the request completes. Sounds good in theory? Well, not exactly! š
The Underlying Issue: Blocking the Browser and Delays
ā Using synchronous AJAX requests may seem convenient at first, but it comes at a cost. It blocks the browser from rendering or handling any other requests until the current request is completed. This can lead to a sluggish user experience, delays, and even browser freezing. Yikes! š„
The Easy Solution: Embrace Asynchronous AJAX Requests
š” In most cases, switching to asynchronous AJAX requests is the way to go. By default, the async
option is set to true
, allowing requests to run asynchronously in the background while your code continues execution. This means no more blocking the browser and better overall performance! š
So, let's modify our getRemote()
function to use asynchronous AJAX requests:
function getRemote() {
return $.ajax({
type: "GET",
url: remote_url,
});
}
The Power of Promises: Handling Returned Data
š Great! Now that we're making asynchronous AJAX requests, how do we handle the returned data? Here comes the power of Promises! šŖ
getRemote().then(function(data) {
// handle the returned data here
});
š” By using the .then()
method, we can ensure that the returned data is properly handled when the AJAX request is completed. So, say goodbye to those missing return values and hello to rock-solid code! š¤
A Final Note: Considerations and Best Practices
ā Asynchronous AJAX requests are generally the recommended approach, but it's essential to consider your specific use case. If you truly require a synchronous request, evaluate the impact on user experience and ensure it's necessary.
Join the Conversation: Share Your AJAX Tales!
š£ Now that you've mastered synchronous AJAX requests in jQuery, we'd love to hear your thoughts and experiences! Have you encountered any unique challenges or found creative solutions? Share your stories in the comments below and let's keep the conversation going! š¬
Conclusion: Unleash the Power of Asynchronous AJAX Requests
š Congratulations, tech wizards! You've successfully navigated the world of synchronous AJAX requests in jQuery and discovered the wonders of asynchronous requests. Remember, great coding involves finding the right balance between convenience and efficiency. Happy coding and let the asynchronous magic flow through your projects! š
š Don't forget to subscribe to our newsletter for more exciting tech tips and tricks. Until next time, keep exploring, stay curious, and keep those AJAX requests flowing! šāØ
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
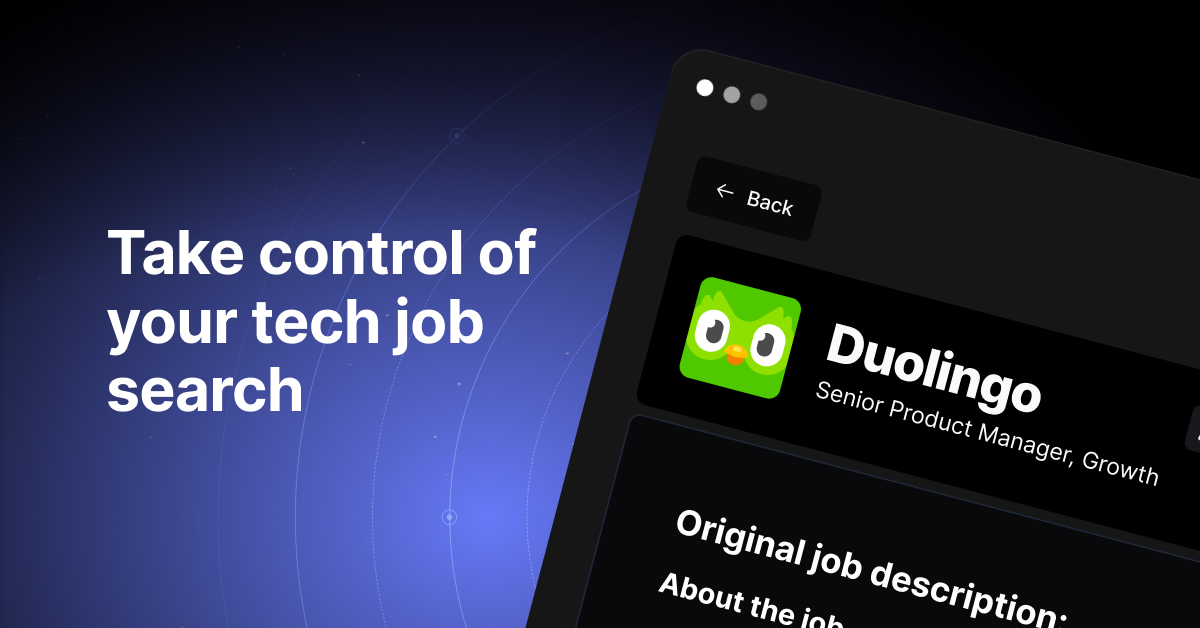