WPF - How to force a Command to re-evaluate "CanExecute" via its CommandBindings
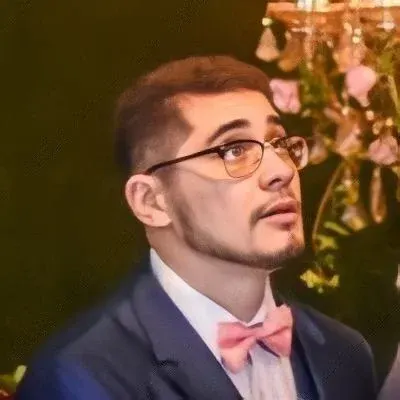
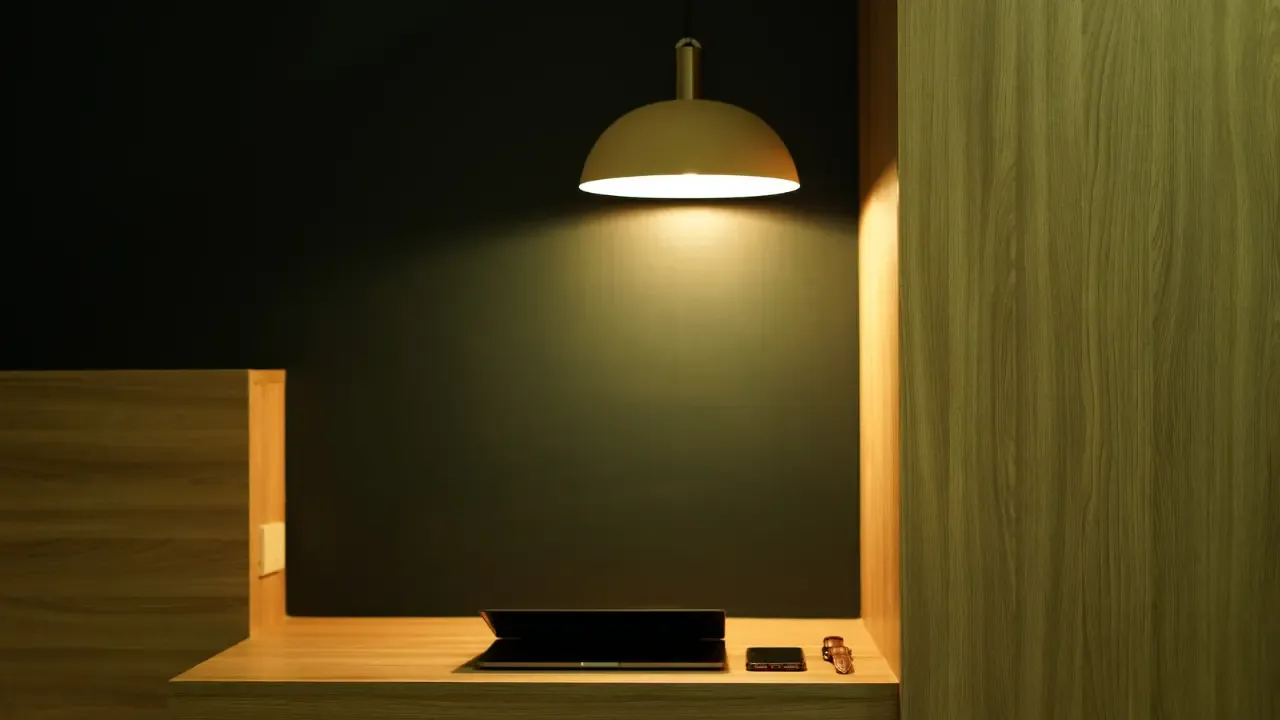
WPF - How to force a Command to re-evaluate 'CanExecute' via its CommandBindings
š® Are you struggling with a WPF menu where the command's CanExecute is not re-evaluated when the underlying data changes? Frustrating, right? š« Well, fear not! In this blog post, we'll address this common issue and provide you with easy solutions to make your commands reflect the latest state of your data. š
The Problem
š§ Let's set the context first. You have a Menu with multiple MenuItems, each bound to a RoutedCommand you've defined. In your CommandBinding, you have a callback that determines the CanExecute state of each MenuItem. Initially, everything seems to be working fine, with the menu items correctly enabled or disabled based on your callback logic.
š However, trouble arises when the data your CanExecute callback relies on changes. The command doesn't automatically re-evaluate CanExecute, causing the UI to become out-of-sync with the current state of your data. Yikes! š±
The Solution
š” Luckily, there are simple solutions to resolve this issue and force the command to re-evaluate CanExecute when your data changes. Let's dive into two approaches you can take:
Approach 1: Implement INotifyPropertyChanged
āļø One option is to implement the INotifyPropertyChanged interface on the class that holds your command's data. This interface provides an event, PropertyChanged, which you can raise whenever a property affecting CanExecute changes. By doing so, you'll notify the command bindings to check CanExecute and update the UI accordingly.
public class YourDataClass : INotifyPropertyChanged
{
// Your other properties
// Property affecting CanExecute
private bool _isDataValid;
public bool IsDataValid
{
get { return _isDataValid; }
set
{
_isDataValid = value;
OnPropertyChanged(nameof(IsDataValid));
}
}
// INotifyPropertyChanged implementation
public event PropertyChangedEventHandler PropertyChanged;
protected virtual void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
Don't forget to update your CanExecute callback to use the updated property:
private void YourCommand_CanExecute(object sender, CanExecuteRoutedEventArgs e)
{
YourDataClass data = e.Parameter as YourDataClass;
e.CanExecute = data?.IsDataValid == true;
}
Approach 2: Manually trigger CanExecute through CommandManager
āļø Another approach involves manually triggering the re-evaluation of CanExecute using the CommandManager. The CommandManager provides a method called InvalidateRequerySuggested that forces all commands to re-evaluate CanExecute. You can call this method whenever your data changes to ensure that the UI reflects the current state.
// Somewhere in your code when your data changes
YourDataClass data = ...; // Update your data here
CommandManager.InvalidateRequerySuggested();
With this approach, you don't need to implement INotifyPropertyChanged. However, please note that this method causes all commands in your application to re-evaluate CanExecute, which might impact performance if you have a large number of commands.
Conclusion
š Congratulations! You now have the power to force your commands to re-evaluate CanExecute and keep your UI in sync with your data changes. Whether you choose to implement INotifyPropertyChanged or use the CommandManager's InvalidateRequerySuggested method, you can eliminate the frustration of an outdated UI. šŖ
š ļø Try out these solutions in your WPF project today and let us know how they work for you! Have more questions or faced different challenges? Drop a comment below and join the conversation. We're here to help! š¤
š¢ Don't forget to share this blog post with other WPF enthusiasts who may be struggling with similar issues. Together, we can make WPF development easier for everyone! š
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
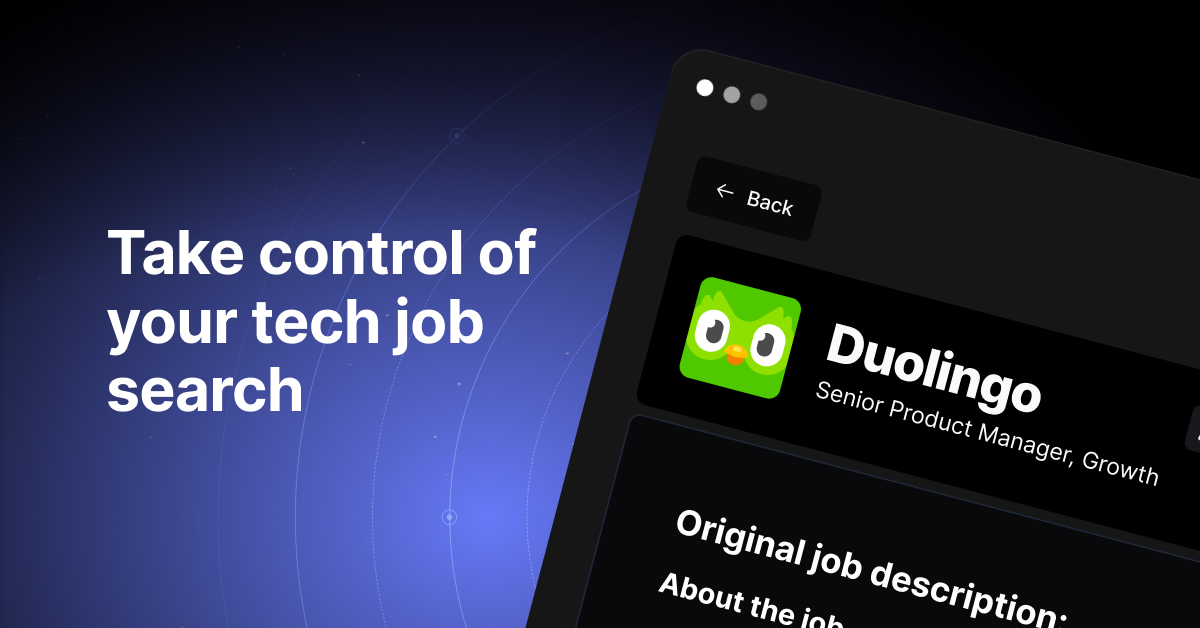