How to make modal dialog in WPF?
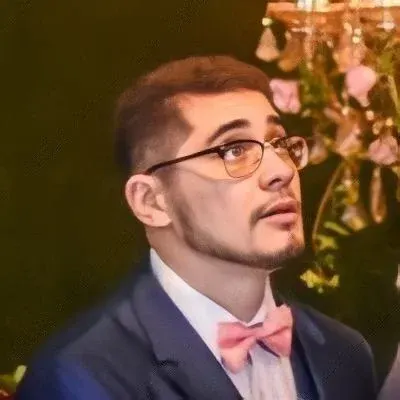
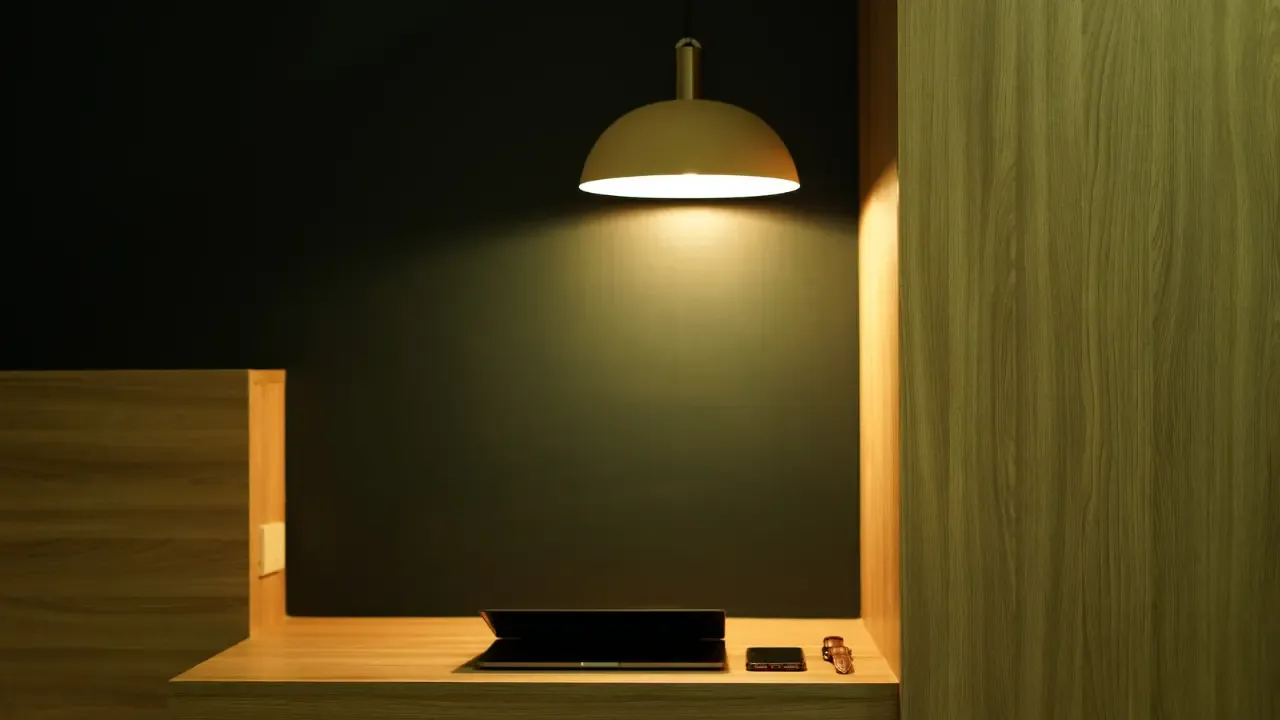
📢🔥**"Master the Art of Modal Dialogs in WPF!"**🔥📢
Are you struggling to create modal dialogs in Windows Presentation Foundation (WPF)? 😕 Don't worry, I've got you covered! In this blog post, I will guide you through the process of making modal dialogs in WPF, addressing common issues and providing easy solutions. So, let's get started and make your UI shine! 💫
✨Understanding Modal Dialogs in WPF✨
Before diving into the implementation, let's quickly understand what modal dialogs are. Modal dialogs are those delightful pop-up windows that capture the user's attention and require them to respond before proceeding further in the application. 🌟 Unlike non-modal windows, modal dialogs hold the user's focus until they interact with them, making them perfect for capturing important information or prompting user actions.
🏞️Creating the XAML Window🏞️
To begin, you should have a XAML window that you want to open as a child window. Let's say you have a "ChildWindow.xaml" file. Now, let's explore how to open it as a modal dialog.
🎬Implementing the Modal Dialog Behavior🎬
To create a modal dialog, follow these simple steps:
Add a reference to the Window class in your code-behind file (usually "ChildWindow.xaml.cs").
using System.Windows;
Inside your code-behind file, add the following code to open the modal dialog window:
// Create an instance of the ChildWindow ChildWindow childWindow = new ChildWindow(); // Set the Owner property of the ChildWindow to the parent window childWindow.Owner = Application.Current.MainWindow; // Show the ChildWindow as a modal dialog childWindow.ShowDialog();
Congratulations! 🎉 You have successfully implemented a modal dialog in WPF. The parent window will wait for the child window to close before continuing its execution.
💡Handling Modal Dialog Results💡
So far, we have seen how to open a modal dialog, but what if you want to capture some results or data from the dialog when it closes? No worries, I've got your back! 😉
To retrieve data from the modal dialog when it closes, you can utilize a property inside the modal dialog class. Let's assume your "ChildWindow" class has a property called "DialogResult" to hold the result:
Inside your "ChildWindow" class, create a public property called "DialogResult":
public bool DialogResult { get; set; }
Now, when the user interacts with the dialog and you want to close it, you can set the "DialogResult" property:
DialogResult = true; Close();
Back in the parent window's code, after calling the "ShowDialog" method, you can access the "DialogResult" property to get the result:
if (childWindow.DialogResult) { // Handle the dialog result here } else { // Do something else if the dialog result is false }
🌠**Time to Level Up Your UI!**🌠
Modal dialogs are an essential component of any interactive application. Now that you've mastered the art of modal dialogs in WPF, it's time to level up your UI and provide a seamless user experience. Remember, modal dialogs hold the user's attention, making them perfect for capturing critical information or guiding user actions.
So, go ahead, implement modal dialogs in your WPF application, and delight your users with a polished and professional interface. 💪✨
🚀**Share Your Success Stories!**🚀
I hope this guide helped you master modal dialogs in WPF like a pro! 😎 Now it's your turn to shine. Share your success stories, screenshots, or even code snippets in the comments section below. Let's learn and grow together! 🌟
💌**Join Our Tech Community!**💌
If you loved this blog post and want more exciting tech tips and tricks, join our exclusive tech community. Subscribe to our newsletter, and stay updated with the latest trends and insights. Together, let's conquer the tech world! 🌍🔥
Keep coding and keep shining! Happy modal dialog creating! 😊💻
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
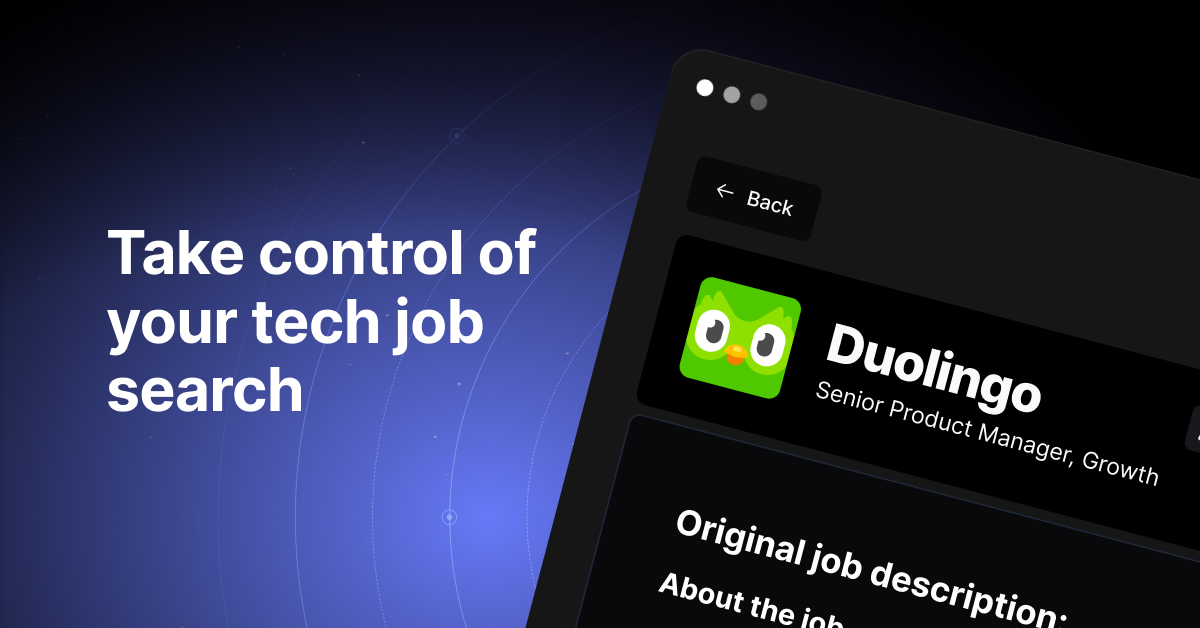